Rust Variables
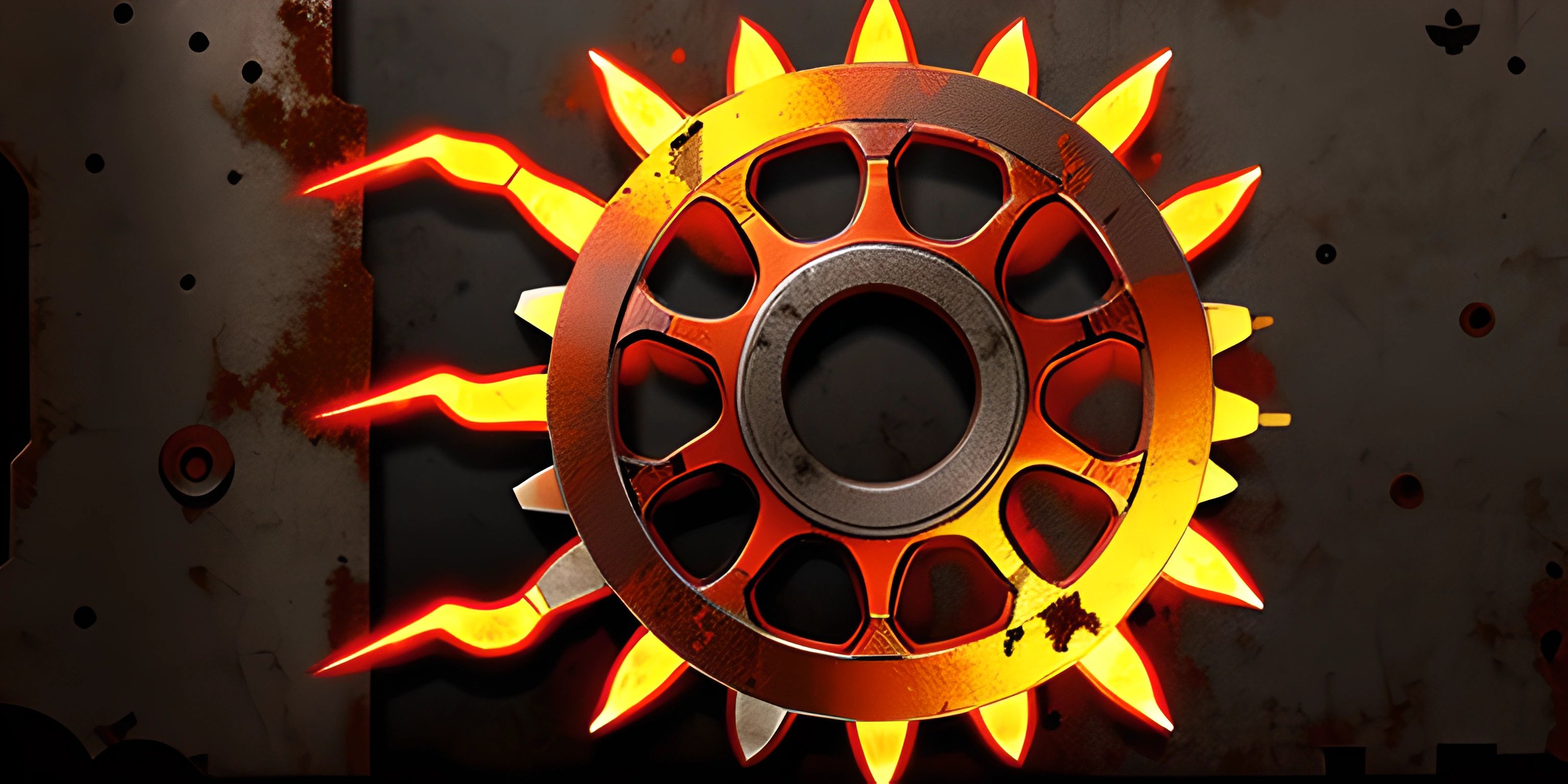
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
In the world of Rust programming, variables are essential building blocks. They allow you to store and manipulate data throughout your code. In this article, we'll dive into the world of Rust variables and discover how to declare and use them effectively.
Immutable Variables
In Rust, variables are immutable by default. This means that once you've given them a value, you cannot change it. Let's see an example:
fn main() { let x = 42; x = 13; // This will cause an error }
In the above code, we declare a variable x
and assign it the value 42
. Then, we try to change the value of x
to 13
. However, Rust will not allow this, and it will throw an error. Immutable variables help ensure the safety and consistency of your code.
Mutable Variables
Sometimes, you need a variable that can change its value. In Rust, you can create a mutable variable by adding the mut
keyword when declaring the variable. Here's an example:
fn main() { let mut x = 42; println!("The value of x is: {}", x); x = 13; // This is allowed because x is mutable println!("The new value of x is: {}", x); }
In this example, we declare a mutable variable x
and assign it the value 42
. We then change the value of x
to 13
, and Rust allows it because x
is mutable. Remember to use mutable variables wisely, as they can introduce complexity and potential bugs if not managed carefully.
Variable Types
Rust is a statically typed language, meaning it must know the type of each variable at compile time. However, Rust also has a powerful type inference system, which can often deduce the correct type for your variables automatically. For example:
fn main() { let a = 42; // Rust infers the type as i32 (a 32-bit integer) let b = 3.14; // Rust infers the type as f64 (a 64-bit floating-point number) }
In the above code, Rust automatically infers the type of a
as i32
and the type of b
as f64
. You can also explicitly specify the type of a variable using a type annotation, like this:
fn main() { let a: i32 = 42; let b: f64 = 3.14; }
Explicitly specifying the type of a variable can be helpful when you want to ensure the variable has a specific type, or when Rust cannot automatically infer the correct type.
Conclusion
Rust variables are a fundamental aspect of the language, allowing you to store and manipulate data in your programs. Remember that variables are immutable by default, and you must use the mut
keyword to create mutable variables. Rust has a powerful type inference system, but you can also explicitly specify the type of a variable using a type annotation. Now that you understand Rust variables, you're ready to conquer more complex programming tasks!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Ownership and Borrowing (psst, it's free!).
FAQ
What are the basic types of variables in Rust?
Rust has several basic types of variables, including:
- Integer types:
i8
,i16
,i32
,i64
,i128
,u8
,u16
,u32
,u64
,u128
- Floating-point types:
f32
,f64
- Boolean type:
bool
- Character type:
char
- String types:
&str
andString
How do I declare and initialize a variable in Rust?
To declare and initialize a variable in Rust, you use the let
keyword followed by the variable name, an equal sign, and the initial value. For example:
let my_var = 42;
Can I change the value of a variable after declaring it in Rust?
Yes, but you'll need to use the mut
keyword to indicate that the variable is mutable. Here's an example:
let mut count = 0; count = count + 1;
How do I specify the type of a variable in Rust?
To specify the type of a variable in Rust, you use a colon followed by the type after the variable name. For example:
let my_var: i32 = 42;
What is shadowing in Rust, and how do I use it?
Shadowing is a feature in Rust that allows you to reuse a variable name, effectively creating a new variable that "shadows" the original one. To use shadowing, declare a new variable with the same name as an existing one using the let
keyword. For example:
let x = 5; let x = x + 1; let x = x * 2;