Polymorphism in Programming
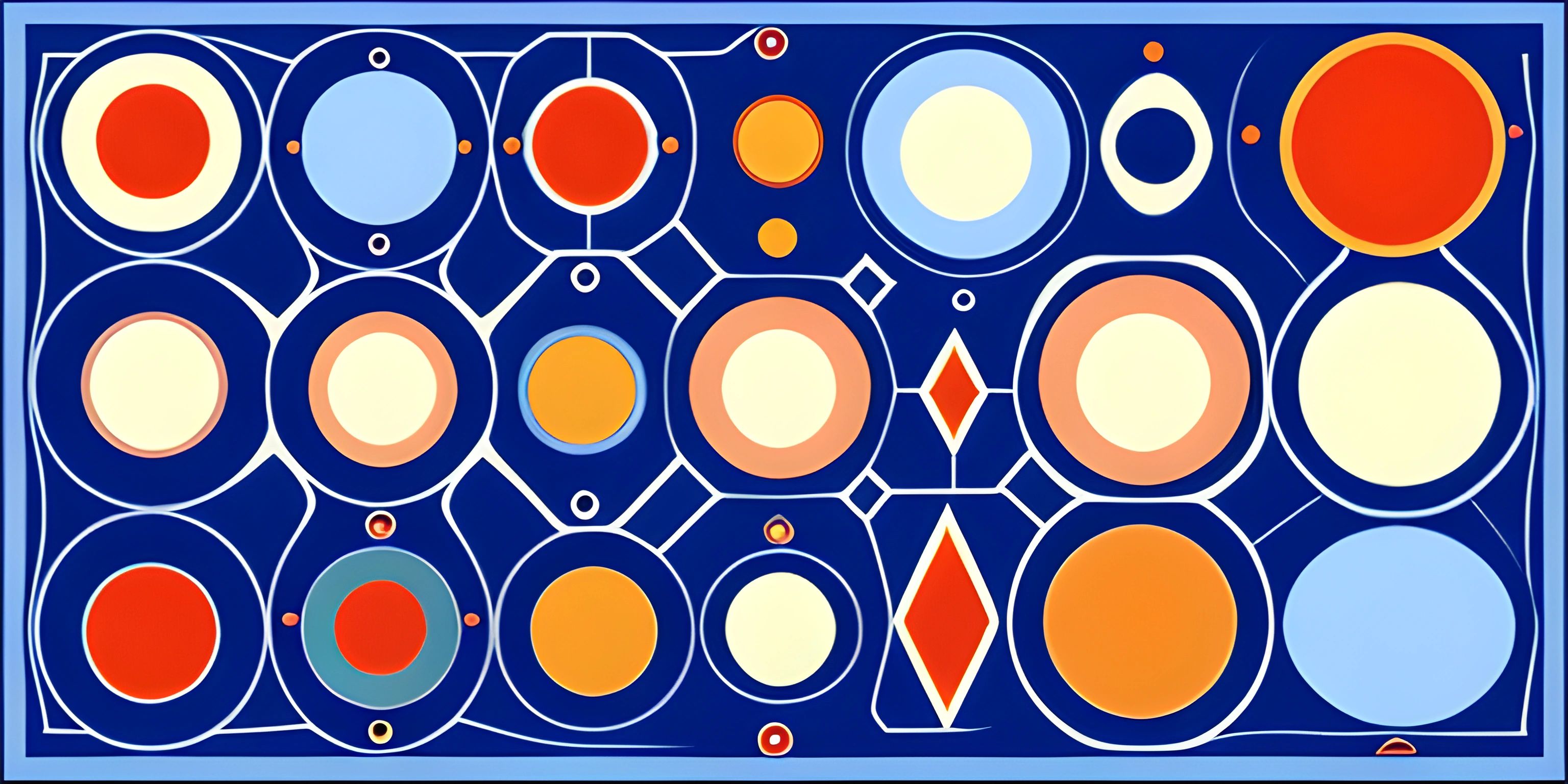
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Polymorphism is a concept that plays a significant role in object-oriented programming (OOP) and makes our code more flexible and extensible. To understand how it works, let's start with a brief overview of OOP.
Object-Oriented Programming
In object-oriented programming, we represent real-world entities using classes and objects. Classes define the properties and behaviors of objects, while objects are instances of these classes.
Now, let's dive into the magical world of polymorphism!
Understanding Polymorphism
Polymorphism is a Greek word that means "many forms." In the OOP context, it refers to the ability of a single function, method, or operator to work with multiple types or classes of objects. Polymorphism allows us to write more flexible and reusable code by enabling these elements to adapt to different situations.
There are two primary types of polymorphism in programming: compile-time (or static) and runtime (or dynamic).
Compile-Time Polymorphism
Compile-time polymorphism occurs when the appropriate method or function is determined during compilation. A common example of this is function overloading, where multiple functions have the same name but differ in the number or types of their parameters. The compiler picks the suitable function based on the function call.
Let's take a look at a simple example in Python:
def add(a, b): return a + b def add(a, b, c): return a + b + c result = add(1, 2, 3) # Calls the second add function print(result) # Output: 6
Runtime Polymorphism
Runtime polymorphism occurs when the appropriate method or function is determined during the execution of the program. This is often achieved using inheritance and method overriding.
In method overriding, a subclass provides a new implementation for a method that is already defined in the parent class. This allows the subclass to inherit the properties and methods of the parent class while customizing specific behaviors.
Here's an example in Python:
class Animal: def speak(self): pass class Dog(Animal): def speak(self): return "Woof!" class Cat(Animal): def speak(self): return "Meow!" my_dog = Dog() my_cat = Cat() print(my_dog.speak()) # Output: Woof! print(my_cat.speak()) # Output: Meow!
The speak
method is overridden in both Dog
and Cat
subclasses, providing different implementations while still adhering to the same method signature in the Animal
class.
Benefits of Polymorphism
Polymorphism offers several benefits in programming:
- Flexibility: Polymorphism allows us to define a single interface for different types, making it easier to add new classes or modify existing ones without changing the overall structure.
- Reusability: By enabling a function, method, or operator to work with multiple types, we can write more reusable code and reduce duplication.
- Extensibility: Polymorphism simplifies code maintenance and makes it easier to extend the functionality of a program by adding new classes or modifying existing ones.
In conclusion, polymorphism is an essential concept in object-oriented programming that allows us to write more flexible, reusable, and extensible code. By understanding and applying polymorphism, we can create programs that are easier to maintain and adapt to different scenarios.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is polymorphism in programming?
Polymorphism is a concept in object-oriented programming that allows objects of different classes to be treated as objects of a common superclass. It enables a single function or method to handle different data types and class objects, making the code more reusable and easier to maintain. In simple terms, polymorphism allows one interface to represent multiple forms of data.
Can you give an example of polymorphism in programming?
Certainly! Let's consider a simple example in Python:
class Animal: def speak(self): pass class Dog(Animal): def speak(self): return "Woof!" class Cat(Animal): def speak(self): return "Meow!" def make_sound(animal): print(animal.speak()) dog = Dog() cat = Cat() make_sound(dog) # Output: Woof! make_sound(cat) # Output: Meow!
In this example, both Dog
and Cat
classes inherit from the Animal
class, and they implement their own version of the speak
method. The function make_sound
takes an object of type Animal
as an argument and calls its speak
method. Since Dog
and Cat
are both subclasses of Animal
, we can pass objects of both classes to the make_sound
function, and it will work correctly due to polymorphism.
How does polymorphism benefit code maintainability and reusability?
Polymorphism allows you to write more flexible and reusable code by letting a single function or method handle multiple data types or objects of different classes. This reduces code duplication, as you don't need separate functions for each specific class or data type. It also makes the code more maintainable, as changes to the behavior of a function can be made in one place instead of multiple places, reducing the risk of introducing bugs.
Are there different types of polymorphism in programming?
Yes, there are two primary types of polymorphism in programming:
- Compile-time polymorphism (also known as static polymorphism or method overloading): This occurs when the function or method to be called is determined during compile time. In languages like Java or C++, method overloading is an example of compile-time polymorphism.
- Run-time polymorphism (also known as dynamic polymorphism or method overriding): This occurs when the function or method to be called is determined during run time. In object-oriented programming languages, method overriding in inherited classes is an example of run-time polymorphism.
Can polymorphism be applied to non-object-oriented programming languages?
Polymorphism is mainly associated with object-oriented programming (OOP) languages, but the concept can also be applied to some extent in non-OOP languages. For example, in functional programming languages, higher-order functions (functions that accept other functions as arguments) can provide a form of polymorphism. In procedural languages like C, function pointers and callback functions can also facilitate polymorphic behavior, although it's not as seamless as in OOP languages.