Introduction to the Stack Data Structure
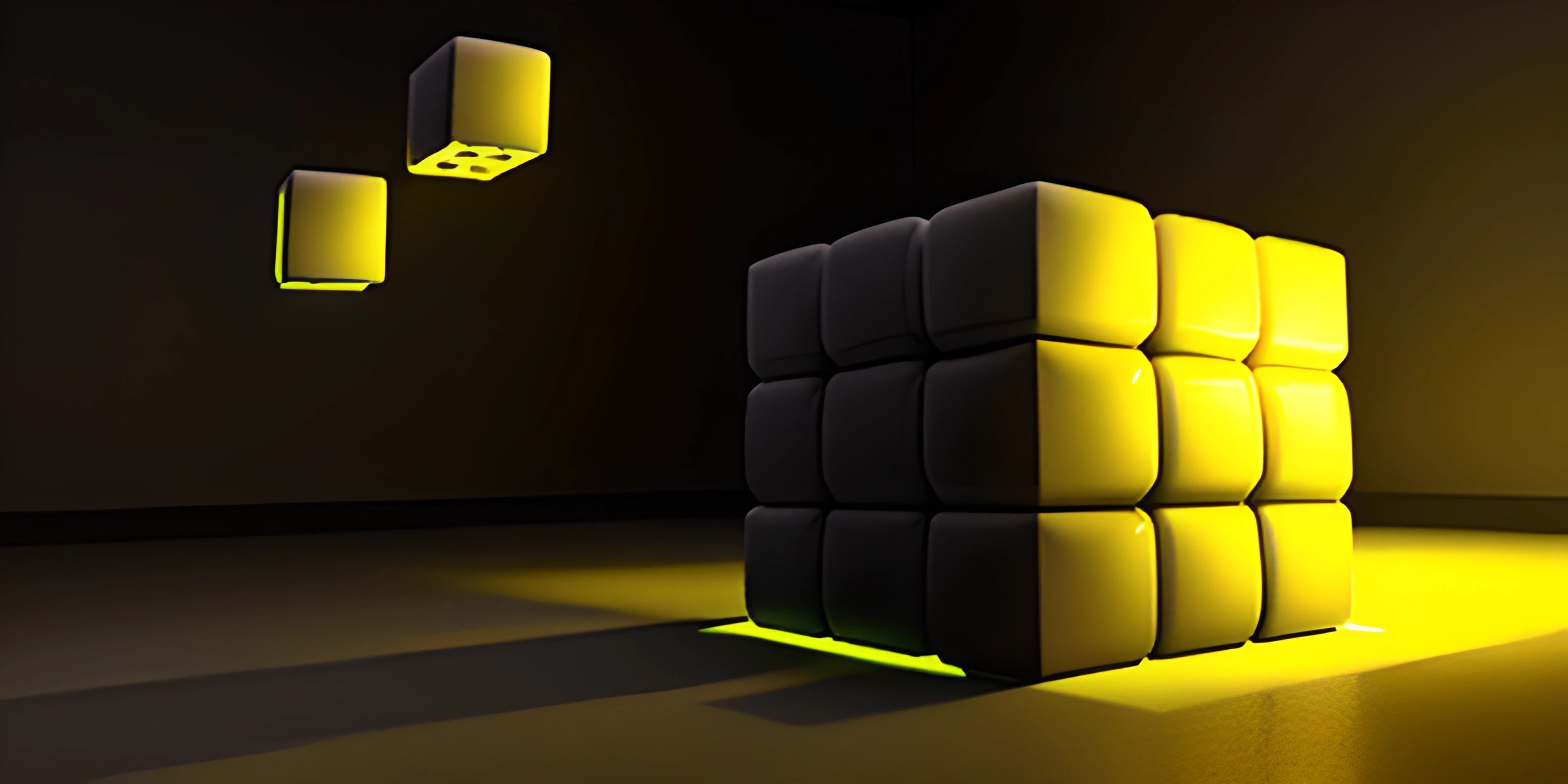
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
There's a data structure that stands tall and unwavering in the realm of programming, like a Jenga tower after a few turns. It's the stack! Stacks are simple, yet powerful, and have a unique way of storing and organizing data. Let's dive in and learn how stacks can give your code a new level of stability.
What is a Stack?
Imagine a stack of plates in a cafeteria. You can only add or remove plates from the top. The same principle applies to a stack data structure. It's a linear data structure that follows the Last-In-First-Out (LIFO) principle, meaning the element added most recently is the first to be removed.
Stacks are used in various programming scenarios, such as managing function calls, handling undo/redo actions, or even parsing expressions.
Operations on a Stack
A stack has two primary operations:
- Push: Adds an element to the top of the stack.
- Pop: Removes the top element from the stack.
Additionally, stacks often include a peek operation to view the top element without removing it and a way to check if the stack is empty.
Here's a simple pseudocode example of a stack:
stack.push("Plate 1") stack.push("Plate 2") stack.push("Plate 3") print stack.pop() // Output: Plate 3 print stack.peek() // Output: Plate 2
Implementing a Stack
A stack can be implemented using an array or a linked list. The choice depends on your specific use case.
Array-based Stack
An array-based stack implementation uses an array to store the elements. The push operation adds an element at the end of the array, and the pop operation removes the last element. Array-based stacks have a fixed size, so you need to resize the array if the stack grows beyond its capacity.
Linked List-based Stack
A linked list-based stack implementation uses a linked list to store the elements. The push operation adds an element at the beginning of the list, and the pop operation removes the first element. Linked list-based stacks can grow and shrink dynamically without resizing.
Stack Limitations and Alternatives
The primary limitation of a stack is its LIFO behavior, which might not suit all situations. If you need a data structure that follows the First-In-First-Out (FIFO) principle, consider using a queue instead.
Don't let the simplicity of the stack fool you. This resilient data structure can help you solve many programming problems and build a strong foundation for your code. Now go stack up on knowledge and let the power of the stack propel you forward!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Full-stack Web Frameworks (Next.js) (psst, it's free!).