Stacks and Queues
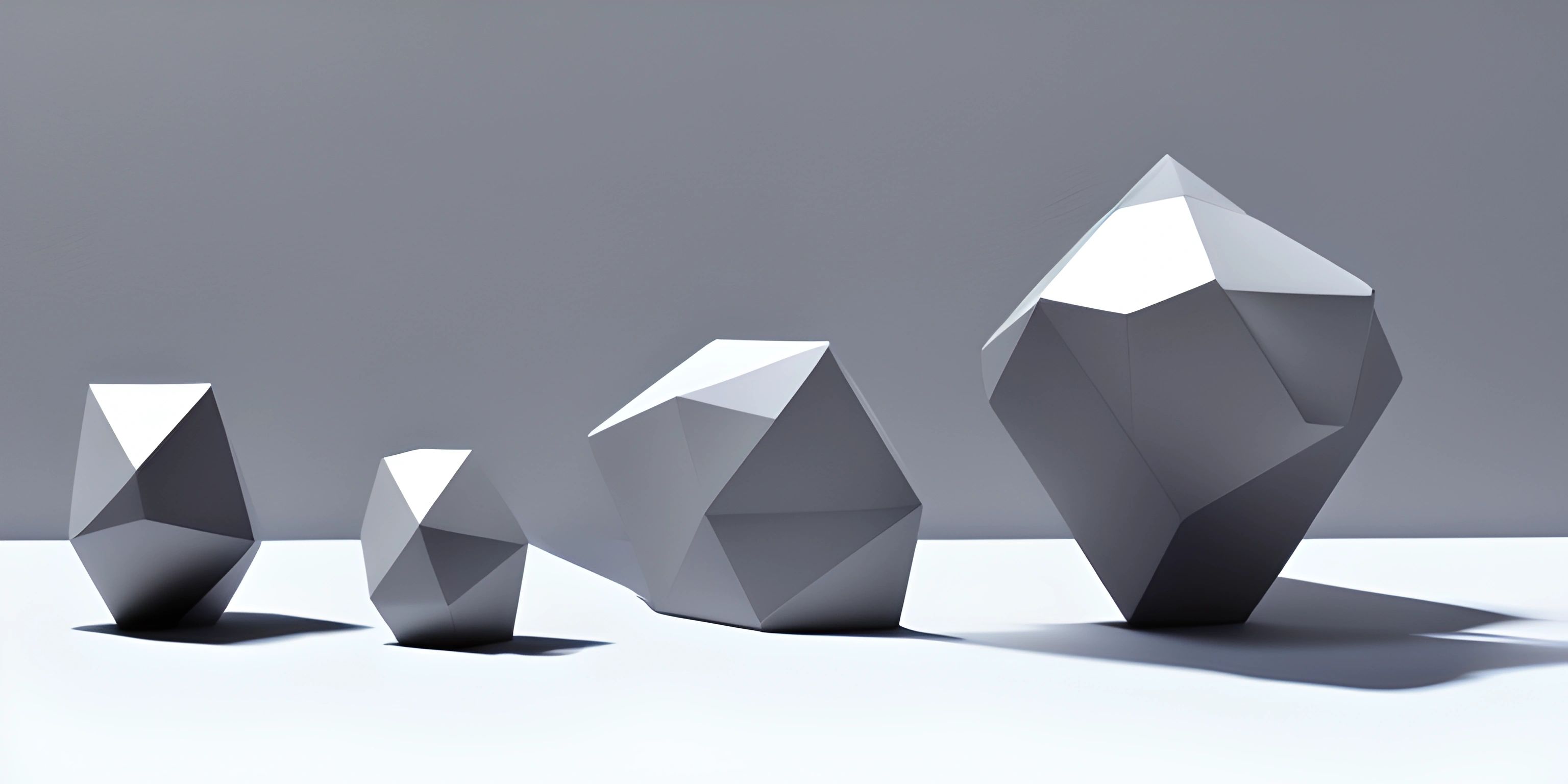
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
When working with data structures in programming, two important concepts to grasp are stacks and queues. Imagine stacks and queues as ways to organize a collection of items, like books in a library or tasks in a to-do list.
Stacks
A stack is a data structure that follows the Last-In, First-Out (LIFO) principle. Think of a stack of plates: you can only add or remove plates from the top of the stack. When you add a plate, it becomes the new top, and when you remove a plate, you take it from the top.
In programming, the main operations performed on a stack are:
- Push: Add an item to the top of the stack.
- Pop: Remove the top item from the stack.
- Peek: Look at the top item without removing it.
Here's a simple example in Python:
stack = [] # Push items onto the stack stack.append("apple") stack.append("banana") stack.append("cherry") # Peek at the top item print(stack[-1]) # Output: cherry # Pop items off the stack print(stack.pop()) # Output: cherry print(stack.pop()) # Output: banana
Queues
A queue is a data structure that follows the First-In, First-Out (FIFO) principle. Picture a queue of people waiting in line: the person who arrived first will be served first, and new people can only join at the end of the line.
In programming, the main operations performed on a queue are:
- Enqueue: Add an item to the end of the queue.
- Dequeue: Remove the item from the front of the queue.
- Front: Look at the front item without removing it.
Here's a simple example in Python:
from collections import deque queue = deque() # Enqueue items queue.append("apple") queue.append("banana") queue.append("cherry") # Look at the front item print(queue[0]) # Output: apple # Dequeue items print(queue.popleft()) # Output: apple print(queue.popleft()) # Output: banana
Real-World Applications
Both stacks and queues can be applied to various real-world scenarios:
Stacks
- Undo/Redo: In text editors or graphic design software, stacks can be used to keep track of actions for undo and redo functionality.
- Expression evaluation: Stacks are used in evaluating arithmetic expressions and converting notations (e.g., infix to postfix).
- Syntax parsing: Compilers use stacks for syntax parsing and checking the correctness of parentheses, brackets, and braces in the code.
Queues
- Task scheduling: Operating systems use queues to manage processes and allocate resources based on their arrival order.
- Printing: Print spoolers use queues to manage print jobs in the order they are received.
- Customer service: Queues are used in call centers to manage the order in which customers are served.
Understanding stacks and queues will make you a better programmer and help you tackle complex problems in creative ways. So, next time you're faced with a challenge, remember these data structures and how they can be applied.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Why Program? (psst, it's free!).