Understanding Assembly Languages
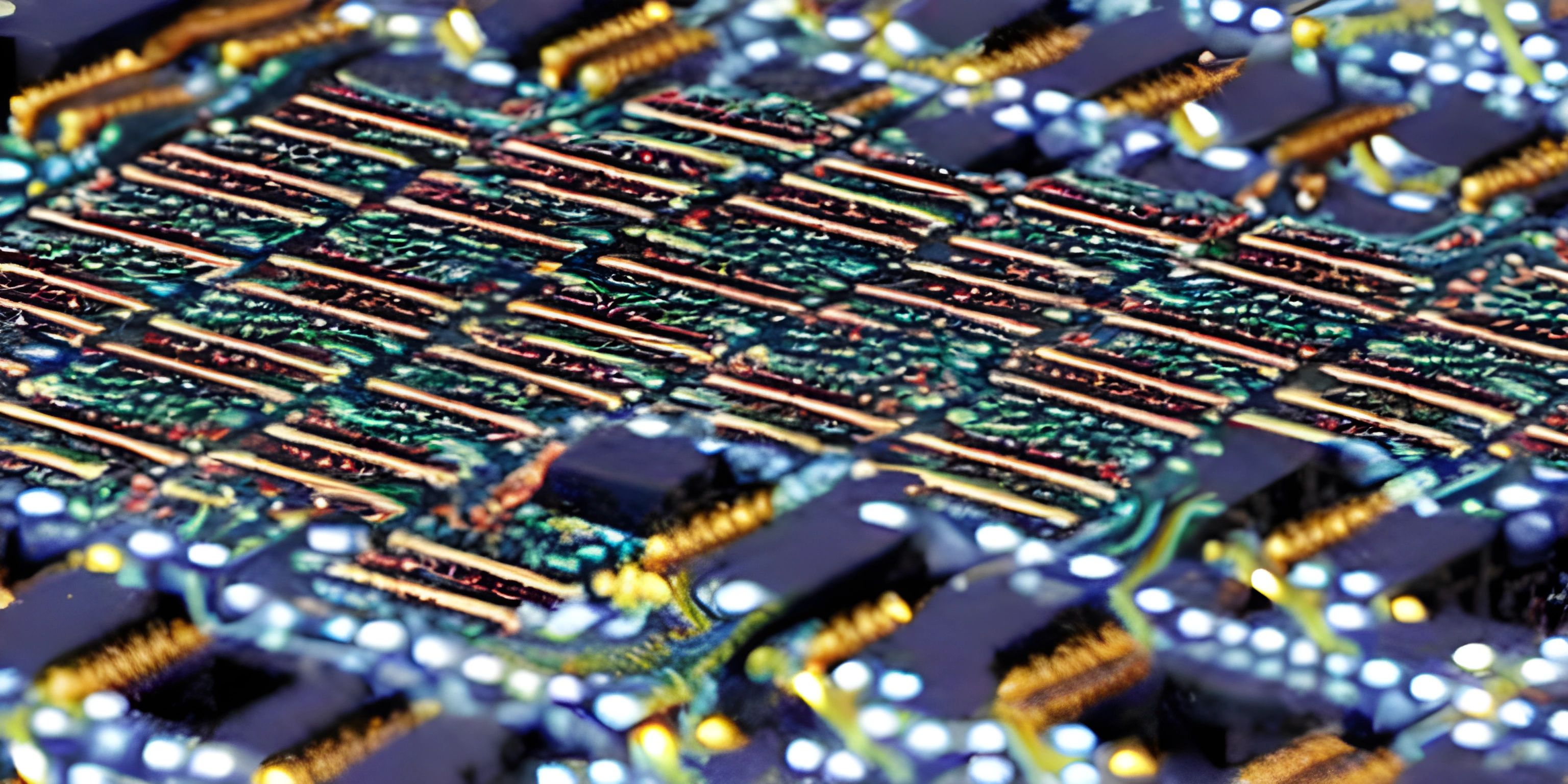
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Welcome to the world of assembly languages! Picture this: you're a wizard, and instead of waving a magic wand, you're commanding a computer with the most basic and primal incantations known to coders. That's essentially what working with assembly language feels like. It's like talking directly to the soul of the machine.
What is Assembly Language?
Imagine you're building a LEGO castle. High-level programming languages like Python or Java are like a box of pre-assembled LEGO structures – you can just snap them together. Assembly language, on the other hand, is like having a huge pile of individual LEGO bricks, and you have to meticulously piece them together one by one. With great power comes great responsibility!
Assembly language is a low-level programming language that is one step above machine code. It gives you direct control over the hardware, which makes it incredibly powerful but also quite complex. Think of it as the Rosetta Stone of programming languages. It translates human-readable code into machine code that the CPU understands.
Purpose of Assembly Language
So why would anybody want to use assembly language? Good question! Here are a few reasons:
- Performance: Assembly language programs can be highly optimized for performance. Since you're writing code that interacts directly with the hardware, you can make it run as fast as possible.
- Control: Sometimes, you need to have precise control over what the hardware is doing. Assembly language allows you to manipulate hardware registers and memory addresses directly.
- Learning: Understanding assembly language helps you gain a deeper understanding of how computers work under the hood. It's like peeking behind the curtain of the great and powerful Oz.
- Legacy Systems: Many older systems and embedded systems still use assembly language. Knowing how to read and write it can be crucial for maintaining and updating these systems.
The Basics of Assembly Language
Instructions and Registers
At the heart of assembly language are instructions and registers. Instructions are the commands you give to the CPU, and registers are small storage locations within the CPU that hold data temporarily.
Here's a simple example for an x86 assembly language:
MOV AX, 5 ; Move the value 5 into register AX MOV BX, 10 ; Move the value 10 into register BX ADD AX, BX ; Add the value in BX to the value in AX
In this example, we're performing basic arithmetic by moving values into registers and then adding them. It's like giving the CPU a step-by-step recipe to follow.
Memory and Addressing
In assembly, memory is your playground, but you need to know how to navigate it. Every piece of data has a specific address. Think of memory as a huge hotel, and each room has a number. You need to know the room number (address) to put something in or take something out.
MOV [1000H], AX ; Move the value in AX to the memory address 1000H
Here, we're moving the value stored in the AX register to the memory address 1000H.
Labels and Branching
Labels are like bookmarks in your code. They help you navigate by marking important spots. Branching allows you to jump to different parts of your code based on certain conditions, similar to "choose your own adventure" stories.
START: ; Label named START MOV AX, 0 ; Initialize AX to 0 MOV CX, 10 ; Initialize CX to 10 LOOP: ; Label named LOOP ADD AX, 1 ; Increment AX by 1 LOOP LOOP ; Decrement CX and loop if CX is not zero
In this example, the LOOP
instruction will repeatedly execute until the value in the CX register is zero.
Assembly Language in Action
To really appreciate assembly language, let's look at a simple program that prints "Hello, World!" on the screen. This will involve direct interaction with the system's hardware.
Here is an example using the NASM assembler for Linux:
section .data hello db 'Hello, World!',0 ; The string to print section .text global _start _start: ; Write the string to stdout mov eax, 4 ; Syscall number (sys_write) mov ebx, 1 ; File descriptor (stdout) mov ecx, hello ; Pointer to the string mov edx, 13 ; Length of the string int 0x80 ; Call kernel ; Exit the program mov eax, 1 ; Syscall number (sys_exit) xor ebx, ebx ; Exit code 0 int 0x80 ; Call kernel
This code may look a bit intimidating, but let's break it down:
- We define a data section to store our string "Hello, World!".
- We define a text section where the actual code lives.
- The
_start
label marks the beginning of our program. - We use a syscall to write our string to the standard output (stdout).
- Finally, we exit the program with another syscall.
Conclusion
Understanding assembly language can feel like learning an ancient and mystical dialect. It might be challenging, but it gives you unparalleled insight into how computers work. It's a powerful tool that's essential for certain types of programming, especially when you need performance, control, or are working with legacy systems.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
Why is assembly language difficult to learn?
Assembly language is challenging because it requires a detailed understanding of computer architecture, including memory management and CPU instructions. It's less abstract than high-level languages and demands meticulous attention to detail.
What are some common uses for assembly language today?
Assembly language is commonly used in embedded systems, real-time systems, operating system kernels, and performance-critical applications. It is also used for reverse engineering and debugging.
How does assembly language differ from machine code?
Assembly language is a human-readable representation of machine code. While machine code consists of binary instructions, assembly language uses mnemonics to represent these instructions, making it easier to read and write.
Can I write an entire application in assembly language?
Yes, you can write an entire application in assembly language, but it's generally impractical for large projects due to its complexity and verbosity. Instead, assembly is often used for performance-critical sections of code within applications written in higher-level languages.
Are there different types of assembly languages?
Yes, each processor architecture has its own assembly language. Common examples include x86 assembly, ARM assembly, and MIPS assembly. Each has its own syntax and instruction set.