x86 Assembly: NASM Function Definition
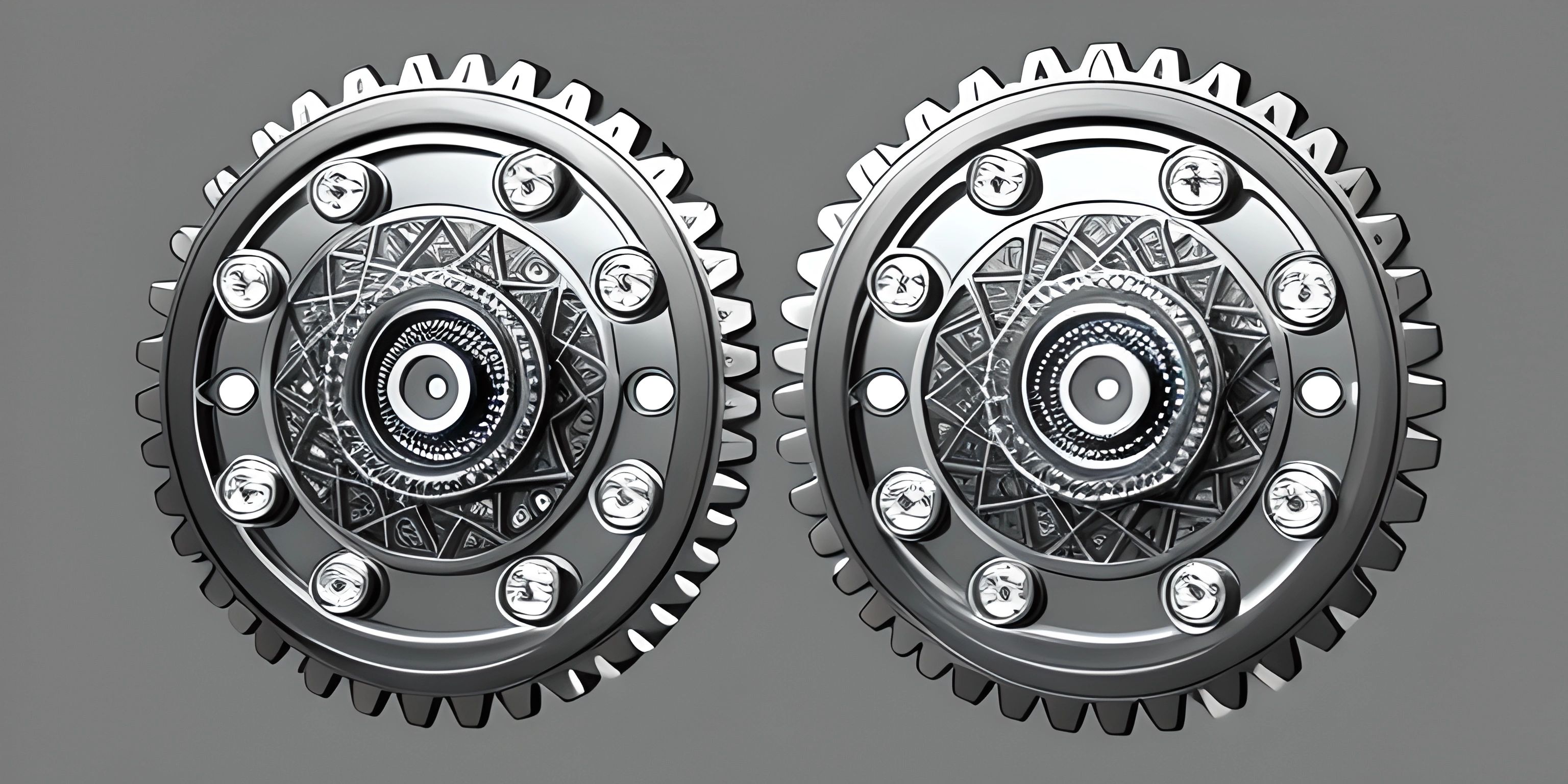
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Delve into the world of x86 Assembly as we explore how to define and call functions using the NASM assembler. Functions, or subroutines, are an essential part of modern programming, allowing us to reuse code and keep our programs organized.
Defining a Function in x86 Assembly with NASM
In x86 Assembly, functions are chunks of code with a label at the beginning, followed by a series of instructions. When defining a function, it's important to adhere to the calling conventions for your platform. We'll be following the cdecl calling convention in this example.
; Function label my_function: ; Save the base pointer push ebp ; Set up the new base pointer mov ebp, esp ; ... Your function's code goes here ... ; Clean up the base pointer mov esp, ebp ; Restore the base pointer pop ebp ; Return from the function ret
This is a basic structure for defining a function in x86 Assembly using NASM. The function starts with the label my_function
. The base pointer is saved, and the stack pointer is set as the new base pointer. The function's instructions are executed, and finally, the base pointer is restored before returning.
Calling a Function in x86 Assembly with NASM
To call a function, you need to pass arguments, if any are required, and then use the call
instruction. In the cdecl convention, arguments are passed on the stack in reverse order. Here's an example of calling a function that takes two arguments:
; Function call example main: ; Push arguments onto the stack (reverse order) push 456 push 123 ; Call the function call my_function ; Clean up the stack add esp, 8 ; ... Continue with the rest of the program ...
In this example, we push the arguments 123
and 456
onto the stack and then call my_function
. After the function has been called and returned, we clean up the stack by adding 8 (the size of the two arguments) to the stack pointer.
A Sample Function in x86 Assembly with NASM
Let's take a look at a complete example of defining and calling a function in x86 Assembly. This function will add two integers and return the result:
; Add two integers and return the result add_integers: ; Save the base pointer push ebp ; Set up the new base pointer mov ebp, esp ; Get the arguments from the stack mov eax, [ebp + 8] ; First argument mov ebx, [ebp + 12] ; Second argument ; Add the two arguments add eax, ebx ; Clean up the base pointer mov esp, ebp ; Restore the base pointer pop ebp ; Return from the function ret ; Function call example main: ; Push arguments onto the stack (reverse order) push 456 push 123 ; Call add_integers function call add_integers ; The result is now in eax register ; Clean up the stack add esp, 8 ; ... Continue with the rest of the program ...
In this example, we define a function add_integers
that adds two integers and returns the result. We then call this function from the main
label.
Now you know how to define and call functions in x86 Assembly using the NASM assembler. This knowledge will allow you to write more modular and organized programs in Assembly, making your programming experience smoother and more enjoyable.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Structs and Traits (psst, it's free!).
FAQ
What is a function definition in x86 Assembly using NASM?
A function definition in x86 Assembly using the NASM (Netwide Assembler) is a way to encapsulate a sequence of instructions that can be called and executed from various parts of your code. Functions help in organizing your code, making it more modular, and easier to maintain.
How do I define a function in x86 Assembly using NASM?
To define a function in x86 Assembly using NASM, follow these steps:
- Choose a unique label for the function, e.g.,
my_function
. - Write the label followed by a colon (e.g.,
my_function:
) to mark the beginning of the function. - Write the function's assembly instructions.
- End the function with a
ret
instruction, which returns control to the calling code. Here's an example of a simple function definition:
my_function: ; Function's assembly instructions go here ret
How do I call a function in x86 Assembly using NASM?
To call a function in x86 Assembly using NASM, use the call
instruction followed by the function's label. For example, to call a function named my_function
, write:
call my_function
The call
instruction saves the current address on the stack and jumps to the specified function. When the called function's ret
instruction is executed, control returns to the instruction after the call
.
How can I pass arguments to a function in x86 Assembly using NASM?
In x86 Assembly using NASM, you can pass arguments to a function by pushing them onto the stack before calling the function. Here's an example of passing two arguments to a function named my_function
:
push arg2 push arg1 call my_function add esp, 8 ; Clean up the stack (assuming each argument is 4 bytes)
Inside the function, you can access the arguments using base pointer-relative addressing, like this:
my_function: push ebp mov ebp, esp ; Access arg1 using [ebp + 8] and arg2 using [ebp + 12] pop ebp ret
Remember to clean up the stack after the function call to remove the pushed arguments.