System Calls: The Bridge Between Programs and the Operating System
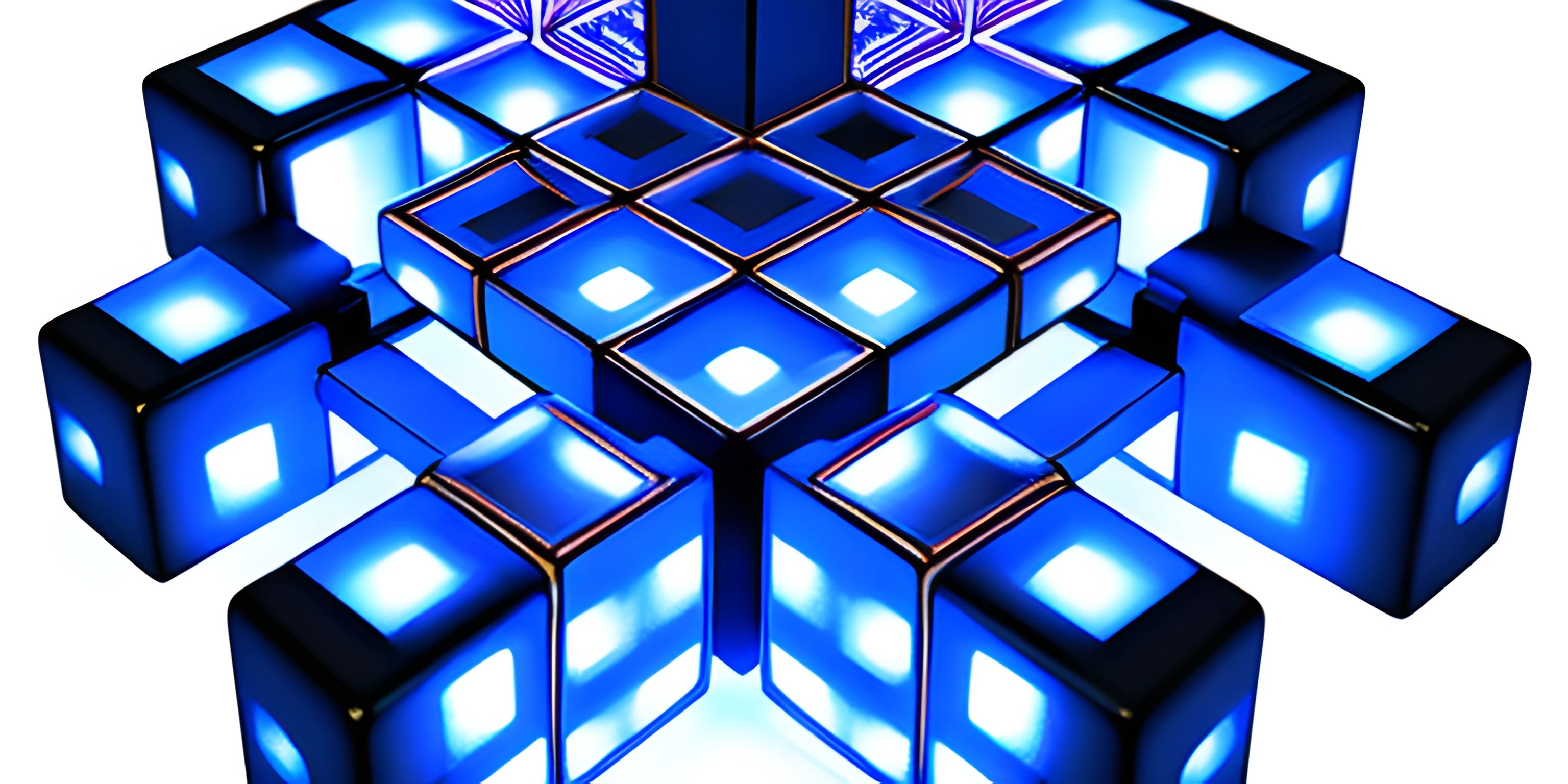
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Picture an orchestra conductor directing a group of musicians. They control the performance, ensuring that each section comes in on cue and that the ensemble creates a harmonious sound. In a similar way, system calls act as the conductors of computer programs, orchestrating the communication between programs and the operating system (OS).
The Role of System Calls
System calls are APIs that provide an interface between a program and the OS. They enable applications to request services from the operating system, such as file handling, memory management, and process control. This allows programmers to access essential system resources without needing to know the intricate details of the underlying hardware or OS.
Types of System Calls
System calls can be broadly categorized into several groups:
-
Process Control: These calls deal with the creation, termination, and management of processes. Examples include forking new processes, ending a process, or waiting for a process to complete.
-
File Management: File-related system calls handle the opening, closing, reading, writing, and deleting of files. They manage file descriptors and ensure proper file access permissions.
-
Device Management: These calls control the interaction between software and physical devices like keyboards, mice, and printers. They manage device drivers and allow programs to access hardware components.
-
Information Maintenance: These calls are responsible for retrieving and setting system information, such as the time, date, or environment variables.
-
Communication: Communication-related system calls manage data transfer between processes, either within the same system (inter-process communication) or across a network (sockets).
System Call Mechanics
When a program needs to make a system call, it transitions from user mode to kernel mode by triggering a software interrupt. The kernel then takes over, performing the necessary operations and returning the results to the user mode application.
Here's a simple example illustrating a system call in action:
#include <stdio.h> #include <unistd.h> int main() { write(1, "Hello, World!\n", 14); return 0; }
In this C program, the write()
function is used to write the string "Hello, World!\n" to the standard output (file descriptor 1
). The write()
function is a wrapper for the write
system call, which performs the actual operation by communicating with the OS.
Why are System Calls Important?
System calls have several key benefits:
-
Abstraction: They provide a consistent interface for accessing system resources, hiding the complexities of the underlying hardware and OS. This makes it easier for developers to create applications that run on a variety of platforms.
-
Security: By limiting direct access to system resources, system calls help maintain the stability and security of the OS. Applications run in user mode with restricted privileges, preventing them from directly manipulating critical system components.
-
Resource Management: System calls allow the OS to manage resources effectively, ensuring that programs have the resources they need while preventing conflicts and resource exhaustion.
In conclusion, system calls are the indispensable bridge between programs and the operating system. They facilitate communication, provide abstraction, and maintain security and resource management, making it possible for applications to harness the power of the computer's hardware and OS in a controlled and efficient manner.
FAQ
What are system calls and why are they important?
System calls are the interface between user programs and the operating system. They allow programs to request services from the OS, such as creating processes, accessing files, and managing memory. System calls are crucial because they enable communication between the program and the OS, providing a way for programs to use the system's resources without directly interacting with the hardware.
How do system calls work?
When a program needs to perform an operation that requires the OS's help, it invokes a system call. The program passes the necessary arguments, such as file names, memory addresses, or process IDs, to the system call. The OS then takes over, performing the requested operation on behalf of the program. Once the operation is complete, the OS returns the result to the program, allowing it to continue executing.
Can you provide an example of a common system call?
Sure! One common system call is the "read()" system call, used to read data from a file. Here's an example of how it might be used in a C program:
#include <unistd.h> #include <fcntl.h> int main() { int file_descriptor = open("example.txt", O_RDONLY); char buffer[1024]; ssize_t bytes_read; if (file_descriptor != -1) { bytes_read = read(file_descriptor, buffer, sizeof(buffer)); close(file_descriptor); } else { // Handle the error } return 0; }
In this example, the program opens a file called "example.txt" and reads its contents into a buffer. The "read()" system call is used to perform the actual reading operation.
Are there different types of system calls?
Yes, system calls can be broadly categorized into different types based on the services they provide. Some common categories include:
- Process Control: These system calls manage processes, such as creating, terminating, or waiting for a process to finish.
- File Management: These system calls handle file operations like opening, closing, reading, and writing.
- Device Management: These system calls deal with device operations, such as controlling and configuring hardware devices.
- Information Maintenance: These system calls provide information about the system, its processes, and resources.
- Communication: These system calls manage inter-process communication and networking.
Can I create my own system calls?
Creating your own system calls is generally not recommended, as it requires modifying the operating system kernel, which can be complex and risky. Instead, it is usually better to use the existing system calls provided by the OS or create user-level libraries that wrap around system calls to provide additional functionality. In some cases, though, creating a custom system call might be necessary for specialized applications or research purposes. Doing so would require a deep understanding of the kernel and OS internals.