x86 NASM Array Creation
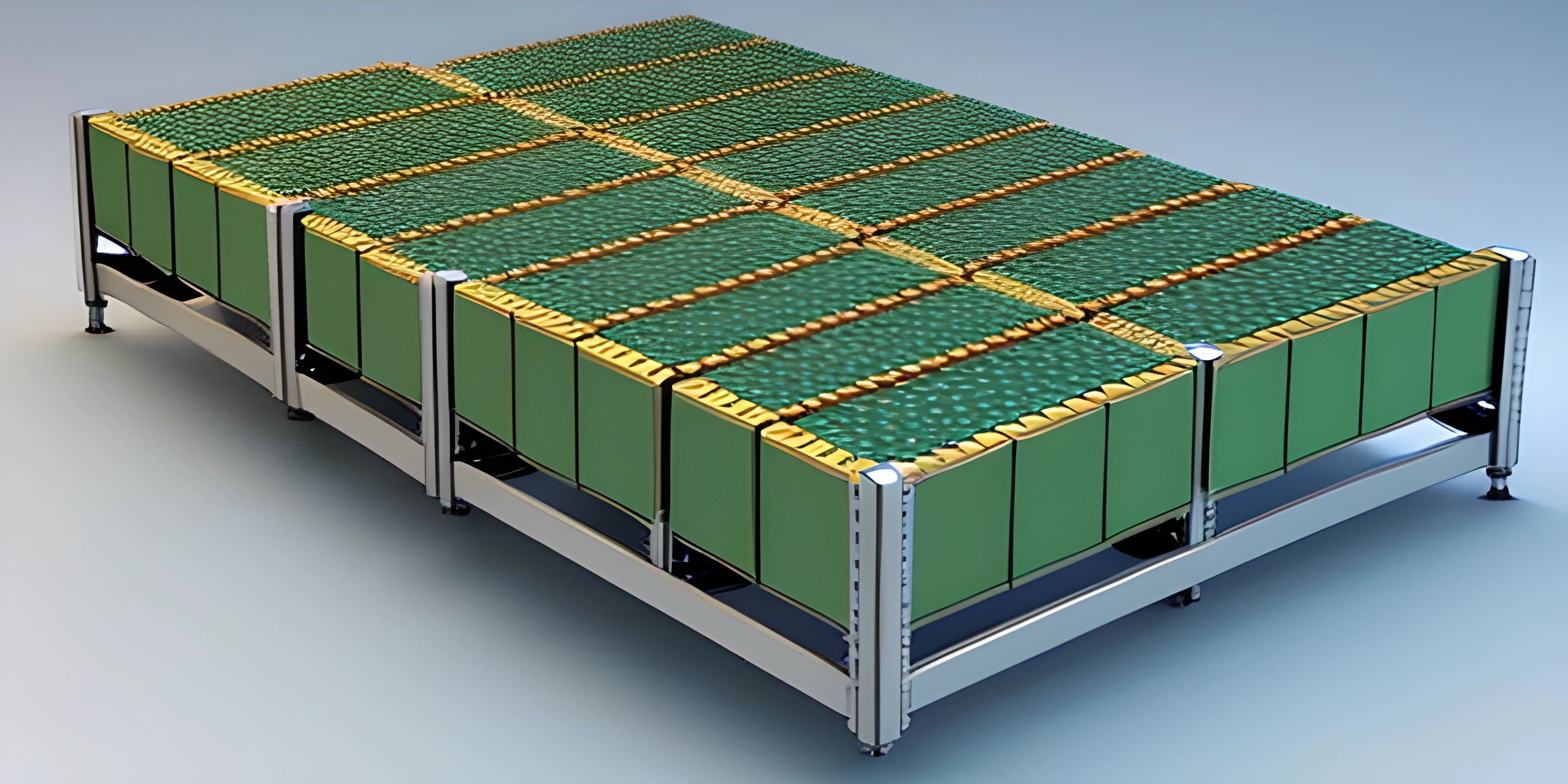
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Creating and initializing arrays in x86 NASM assembly can be a bit tricky, but have no fear! This article will guide you through the process step by step, so you can handle arrays like a champ. Grab your favorite assembler, and let's jump right in!
What is an Array?
An array is a collection of elements, usually of the same data type, stored in contiguous memory locations. They are convenient for organizing and accessing related data. Think of an array as a row of lockers in a school hallway, each containing a piece of data, and you have a key to access each one of them by their index.
Declaring an Array in NASM
First, let's declare an array in NASM. We'll use the section .data
directive to define a data section to store our array. Next, we'll use a label to give our array a name, followed by the db
, dw
, or dd
directive, depending on the size of the elements we want to store.
For example, let's create an array of 5 integers:
section .data array_name db 0, 1, 2, 3, 4
Here, array_name
is the label we gave to the array, and db
(define byte) is the directive to store 8-bit integer values. The numbers 0 through 4 are the elements of our array.
Accessing Array Elements
To access an element in the array, we can use its base address (the label) and an offset (the index). Let's say we want to access the third element (with an index of 2) of the array_name
array:
mov al, [array_name + 2]
In this example, we move the value at the address array_name + 2
(the third element in the array) into the AL
register.
Initializing an Array with a Loop
Sometimes, you may want to initialize an array with a specific pattern or a set of values. This can be accomplished using a loop. Let's create an array of 10 bytes and initialize it with increasing even numbers:
section .data array_even db 10 dup(0) ; Declare an array of 10 bytes initialized with 0 section .text global _start _start: ; Initialize array_even with increasing even numbers mov ecx, 10 ; Set loop counter to 10 (number of elements in the array) mov edi, 0 ; Set current index to 0 loop_start: mov [array_even + edi], edi ; Store the current index (even number) in the array add edi, 2 ; Move to the next even number dec ecx ; Decrement the loop counter jnz loop_start ; If the counter is not 0, jump back to the loop_start
At the end of this loop, array_even
will contain the values 0, 2, 4, 6, 8, 10, 12, 14, 16, and 18.
Now you know how to create and initialize arrays in x86 NASM assembly! With this knowledge, you're one step closer to becoming an assembly wizard. Keep experimenting and have fun with your newfound array powers!
FAQ
What is an array in x86 NASM assembly?
An array in x86 NASM assembly is a contiguous block of memory that stores multiple data elements of the same type. Arrays are useful when you need to work with a large number of data elements and want to access them efficiently using their index.
How do I create an array in x86 NASM assembly?
To create an array in x86 NASM assembly, you need to reserve memory space for the array using the times
directive and the db
, dw
, or dd
directives, depending on the size of the data elements. Here's an example of creating an integer array with four elements:
section .data array1 times 4 dd 0 ; Reserve space for an array of 4 integers (each 4 bytes in size)
How can I initialize an array with specific values in x86 NASM assembly?
You can initialize an array with specific values by providing the values after the db
, dw
, or dd
directives. Here's an example of initializing an integer array with four elements:
section .data array2 dd 10, 20, 30, 40 ; Initialize an array of 4 integers with specific values
How do I access elements in an array using x86 NASM assembly?
To access elements in an array, you can use index-based addressing. You'll need to calculate the memory address of the element using the base address of the array, the index of the element, and the size of each element. Here's an example of accessing the second element (index 1) of an integer array:
section .text mov eax, [array1 + 1 * 4] ; Load the second element of the integer array into eax
How can I iterate through an array in x86 NASM assembly?
You can use a loop to iterate through an array in x86 NASM assembly. Here's an example of a loop that iterates through an integer array with four elements and adds each element to a sum:
section .text mov ecx, 4 ; Set the loop counter to the number of elements mov esi, 0 ; Initialize the index mov eax, 0 ; Initialize the sum loop_start: add eax, [array1 + esi * 4] ; Add the current element to the sum inc esi ; Increment the index dec ecx ; Decrement the loop counter jnz loop_start ; Jump to loop_start if ecx is not zero (i.e., there are still elements to process)