Working with Arrays in x86 NASM Assembly Language
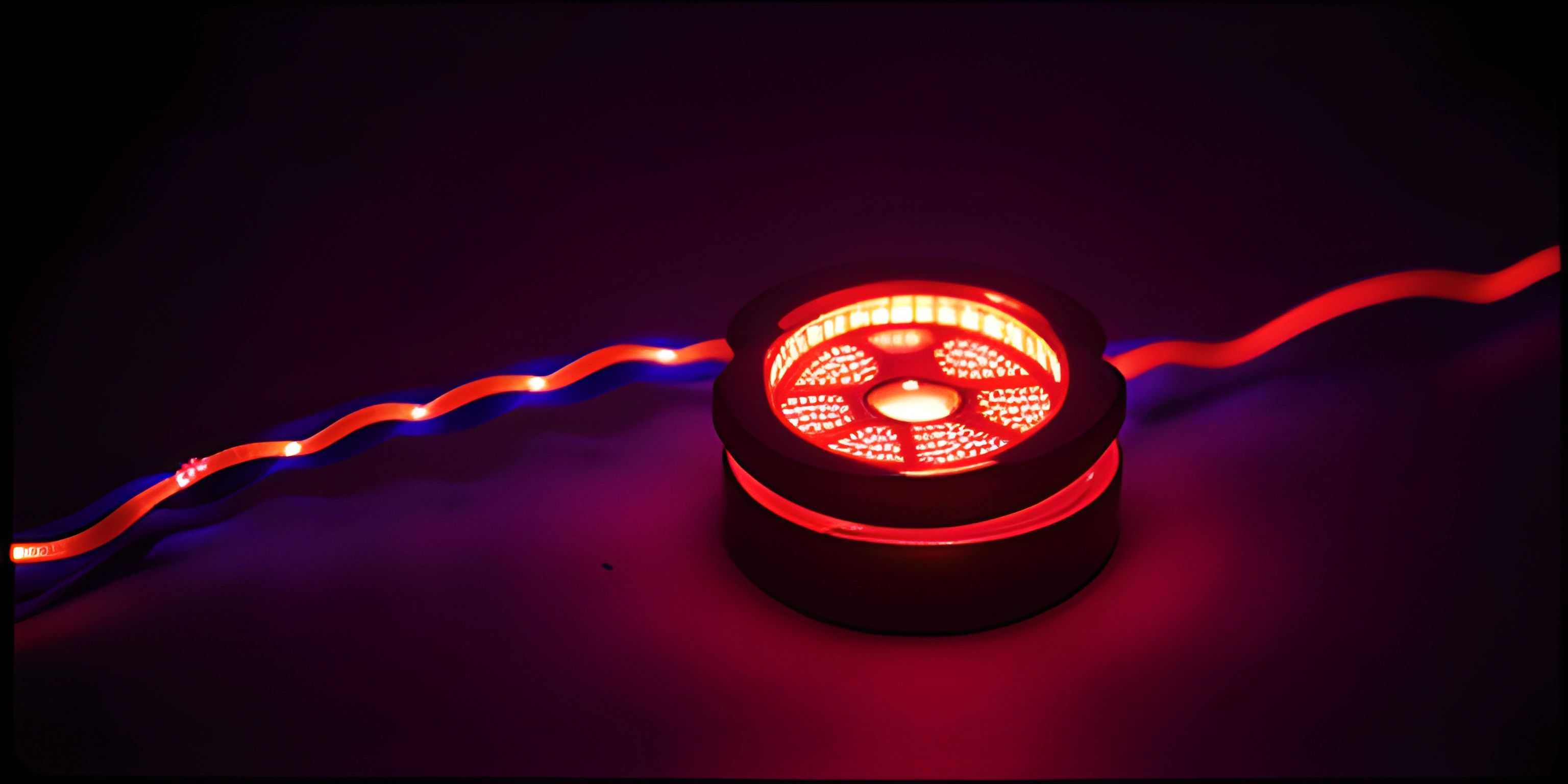
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
When dealing with programming languages like x86 NASM Assembly, manipulating data structures like arrays might seem daunting. Fear not! In this article, we'll cover the essentials of working with arrays in x86 NASM Assembly Language, from creating and initializing arrays to traversing and manipulating their elements.
Creating and Initializing Arrays
In x86 NASM Assembly, arrays are essentially contiguous blocks of memory. To create an array, you'll need to reserve space in memory for it using the db
, dw
, or dd
directive, depending on the size of your elements (byte, word, or double word).
Let's create an array of bytes:
section .data my_array db 1, 2, 3, 4, 5 ; An array of 5 bytes
Here, we've created an array called my_array
with 5 elements (each a byte in size). The elements are initialized to the numbers 1 through 5.
Accessing Array Elements
To access an element in the array, you'll use the base address of the array and an offset. The base address is the address of the first element (my_array
in our example), and the offset is the index of the element you want to access. For example, to access the third element of my_array
, the offset would be 2 (since we start counting from 0).
Here's an example of accessing the third element of my_array
:
section .data my_array db 1, 2, 3, 4, 5 ; An array of 5 bytes section .text mov eax, [my_array + 2] ; Move the third element of my_array into eax
Traversing Arrays
In order to traverse an array, you'll typically use a loop combined with an index (or pointer), which will be incremented to access the next element in the array. For example, here's a loop that traverses my_array
:
section .data my_array db 1, 2, 3, 4, 5 ; An array of 5 bytes array_length equ 5 ; Define the length of the array section .text mov ecx, array_length ; Set the loop counter mov esi, my_array ; Set the pointer to the first element of the array .loop_start: ; Do something with [esi], the current element of the array add esi, 1 ; Move to the next element in the array loop .loop_start ; Decrement ecx and loop if ecx is not zero
In this example, we use esi
as a pointer to the current element of the array and increment it to move through the array. We use ecx
as a loop counter to ensure we don't go past the end of the array.
Manipulating Array Elements
Finally, let's look at how to manipulate the elements of an array. For example, let's say we want to add 2 to each element of my_array
:
section .data my_array db 1, 2, 3, 4, 5 ; An array of 5 bytes array_length equ 5 ; Define the length of the array section .text mov ecx, array_length ; Set the loop counter mov esi, my_array ; Set the pointer to the first element of the array .loop_start: add byte [esi], 2 ; Add 2 to the current element of the array add esi, 1 ; Move to the next element in the array loop .loop_start ; Decrement ecx and loop if ecx is not zero
In this code snippet, we use the add
instruction to add 2 to each element of the array, updating its value in memory.
Now you have a better understanding of how to work with arrays in x86 NASM Assembly Language. Keep practicing and experimenting to become more fluent in array manipulation and other aspects of this powerful low-level programming language. Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Palindromes (psst, it's free!).
FAQ
How do I declare an array in x86 NASM Assembly Language?
To declare an array in x86 NASM Assembly Language, you need to use the db
, dw
, or dd
directives, depending on the size of the elements in your array. For example, to declare an array of bytes, you can use the following code:
section .data array_name db 10, 20, 30, 40, 50 ; Declare an array of 5 bytes
How do I access an element of an array in x86 NASM Assembly Language?
To access an element of an array in x86 NASM Assembly Language, you can use the lea
(Load Effective Address) or mov
instructions, along with the array name and an index value. For example, to access the third element of an array, you can use the following code:
lea esi, [array_name + 2] ; Load the address of the third element into ESI mov al, [esi] ; Load the value of the third element into AL
How can I iterate through an array in x86 NASM Assembly Language?
To iterate through an array in x86 NASM Assembly Language, you can use a loop with a counter. Here's an example of iterating through an array of 5 bytes and adding each element to the eax
register:
section .data array_name db 10, 20, 30, 40, 50 ; Declare an array of 5 bytes section .text mov ecx, 5 ; Set the loop counter to the number of elements in the array lea esi, [array_name] ; Load the address of the first element into ESI loop_start: add eax, [esi] ; Add the value of the current element to EAX inc esi ; Move to the next element dec ecx ; Decrement the loop counter jnz loop_start ; If the loop counter is not zero, jump back to loop_start
How can I perform a specific operation on each element of an array?
You can use a loop to iterate through the array and perform an operation on each element using the appropriate x86 NASM Assembly Language instructions. For example, to multiply each element of an array by 2, you can use the following code:
section .data array_name db 10, 20, 30, 40, 50 ; Declare an array of 5 bytes section .text mov ecx, 5 ; Set the loop counter to the number of elements in the array lea esi, [array_name] ; Load the address of the first element into ESI loop_start: mov al, [esi] ; Load the value of the current element into AL add al, al ; Multiply the value by 2 mov [esi], al ; Store the result back into the array inc esi ; Move to the next element dec ecx ; Decrement the loop counter jnz loop_start ; If the loop counter is not zero, jump back to loop_start
How do I find the length of an array in x86 NASM Assembly Language?
In x86 NASM Assembly Language, you need to manually keep track of the length of an array, as there is no built-in method to find the length. When you declare an array, you should also store its length in a constant or a register. For example:
section .data array_name db 10, 20, 30, 40, 50 ; Declare an array of 5 bytes array_length equ 5 ; Store the length of the array as a constant