x86 NASM Assembly: Array Arguments
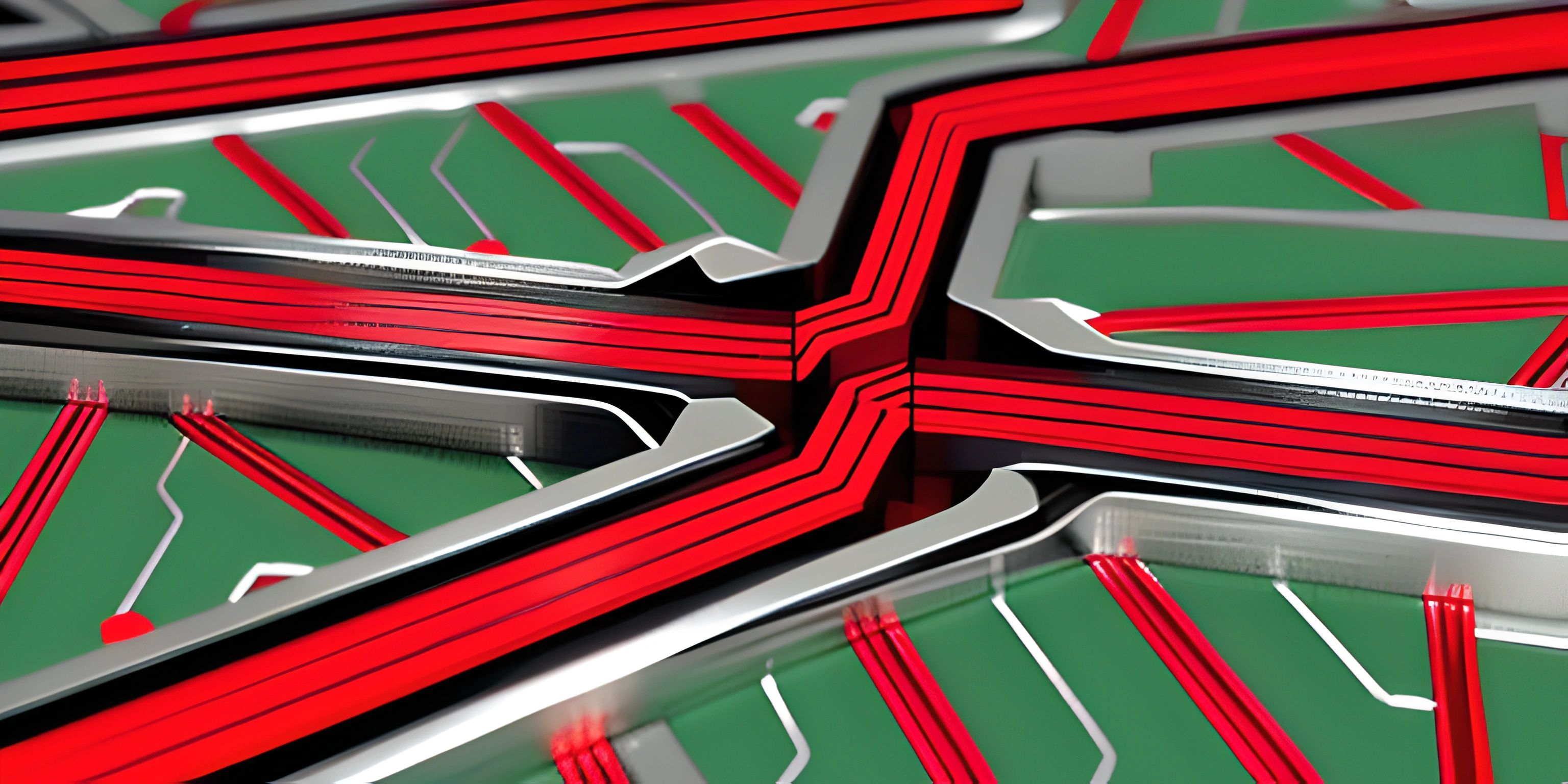
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
When writing programs in x86 NASM assembly, we often need to work with arrays, and there comes a time when we need to pass them as arguments to functions. Today, we'll explore how to do just that.
Arrays in x86 NASM Assembly
An array is a collection of elements, typically of the same data type, stored in contiguous memory locations. In x86 NASM assembly language, we can define an array by reserving memory space for its elements. Let's create an array of four 32-bit integers:
section .data myArray dd 1, 2, 3, 4
This code defines an array called myArray
with four integers: 1, 2, 3, and 4.
Passing Arrays to Functions
When passing an array to a function in x86 NASM assembly, we usually pass a pointer to the array's first element. The function can then use the pointer to access the array's elements. Let's see how we can pass myArray
to a function called printArray
:
Step 1: Prepare the Function Arguments
First, we need to store the address of myArray
in a register. We'll use the lea
(Load Effective Address) instruction to store the address in the eax
register:
section .text global _start _start: lea eax, [myArray] ; Load the address of myArray into eax
Step 2: Call the Function
Next, we'll call the printArray
function using the call
instruction:
call printArray
Step 3: Implement the Function
Now, let's implement the printArray
function. We know that the address of myArray
is in the eax
register, so we can use it to access the elements of the array:
printArray: ; Code to print the array elements using the address in eax ; ... ret ; Return from the function
Example: Print Array Elements
Let's create a complete example where we pass an array to a function and print its elements using the x86 Linux system calls:
section .data myArray dd 1, 2, 3, 4 myArrayLen equ 4 section .text global _start _start: lea eax, [myArray] ; Load the address of myArray into eax call printArray ; Call the printArray function ; Exit the program mov eax, 1 ; Exit syscall xor ebx, ebx ; Return 0 int 0x80 ; Trigger the syscall printArray: ; Print the array elements mov ecx, myArrayLen ; Set the loop counter to the array length .loop: ; Print the array element mov eax, 4 ; Write syscall mov ebx, 1 ; File descriptor (stdout) add esi, [eax] ; Add the array element to the offset lea ecx, [eax + esi] ; Calculate the address of the array element mov edx, 4 ; Element size (4 bytes) int 0x80 ; Trigger the syscall ; Increment the array pointer add eax, 4 ; Decrement the loop counter and loop dec ecx jnz .loop ret ; Return from the function
This code defines a function called printArray
, which prints the elements of myArray
. It passes the array as an argument by loading the address of the array into the eax
register and calling the function.
And there you have it! By following these steps, you can easily pass arrays as function arguments in x86 NASM assembly language. Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Palindromes (psst, it's free!).
FAQ
How do I pass an array as a function argument in x86 NASM assembly language?
To pass an array as a function argument in x86 NASM assembly language, you need to pass the address (or base pointer) of the array and the size of the array. Here's a simple example:
section .data myArray db 1, 2, 3, 4, 5 section .text global _start _start: ; Pass the array and its size to the function lea eax, [myArray] ; Load the address of myArray into eax push eax ; Push the address of myArray onto the stack push 5 ; Push the size of the array (5 elements) onto the stack call print_array ; Call the print_array function add esp, 8 ; Clean up the stack ; ... rest of the code
How can I access the elements of the passed array in the called function?
In the called function, you can access the elements of the passed array using the base pointer and an index. Here's an example of how to access the elements in the print_array
function:
print_array: push ebp ; Save the base pointer mov ebp, esp ; Set the base pointer to the current stack pointer mov eax, [ebp + 8] ; Get the address of the array from the stack mov ecx, [ebp + 12] ; Get the size of the array from the stack xor ebx, ebx ; Clear the ebx register for use as an index loop: cmp ebx, ecx ; Compare the index (ebx) to the size of the array (ecx) jge end_loop ; If the index is greater or equal to the size, jump to end_loop mov dl, [eax + ebx] ; Load the value of the array element at index ebx into dl ; ... print the value in dl inc ebx ; Increment the index (ebx) jmp loop ; Jump back to the start of the loop end_loop: pop ebp ; Restore the base pointer ret 8 ; Return from the function and clean up the stack
How can I pass a multidimensional array as an argument in x86 NASM assembly language?
To pass a multidimensional array, you need to pass the address (or base pointer) of the array, the dimensions of the array, and the size of each dimension. Here's an example:
section .data my2DArray db 1, 2, 3, 4, 5, 6, 7, 8, 9 section .text global _start _start: ; Pass the 2D array and its dimensions to the function lea eax, [my2DArray] ; Load the address of my2DArray into eax push eax ; Push the address of my2DArray onto the stack push 3 ; Push the number of rows (3) onto the stack push 3 ; Push the number of columns (3) onto the stack call print_2D_array ; Call the print_2D_array function add esp, 12 ; Clean up the stack ; ... rest of the code
How do I handle string arrays as arguments in x86 NASM assembly language?
To handle string arrays, pass the address of the array and its size. Each element in the string array should also be a pointer to the beginning of the string. Here's an example:
section .data string1 db 'Hello, world!', 0 string2 db 'Cratecode', 0 string3 db 'Assembly rocks!', 0 stringArray dd string1, string2, string3 section .text global _start _start: ; Pass the string array and its size to the function lea eax, [stringArray] ; Load the address of stringArray into eax push eax ; Push the address of stringArray onto the stack push 3 ; Push the size of the array (3 elements) onto the stack call print_string_array ; Call the print_string_array function add esp, 8 ; Clean up the stack ; ... rest of the code