x86 NASM Assembly Arrays
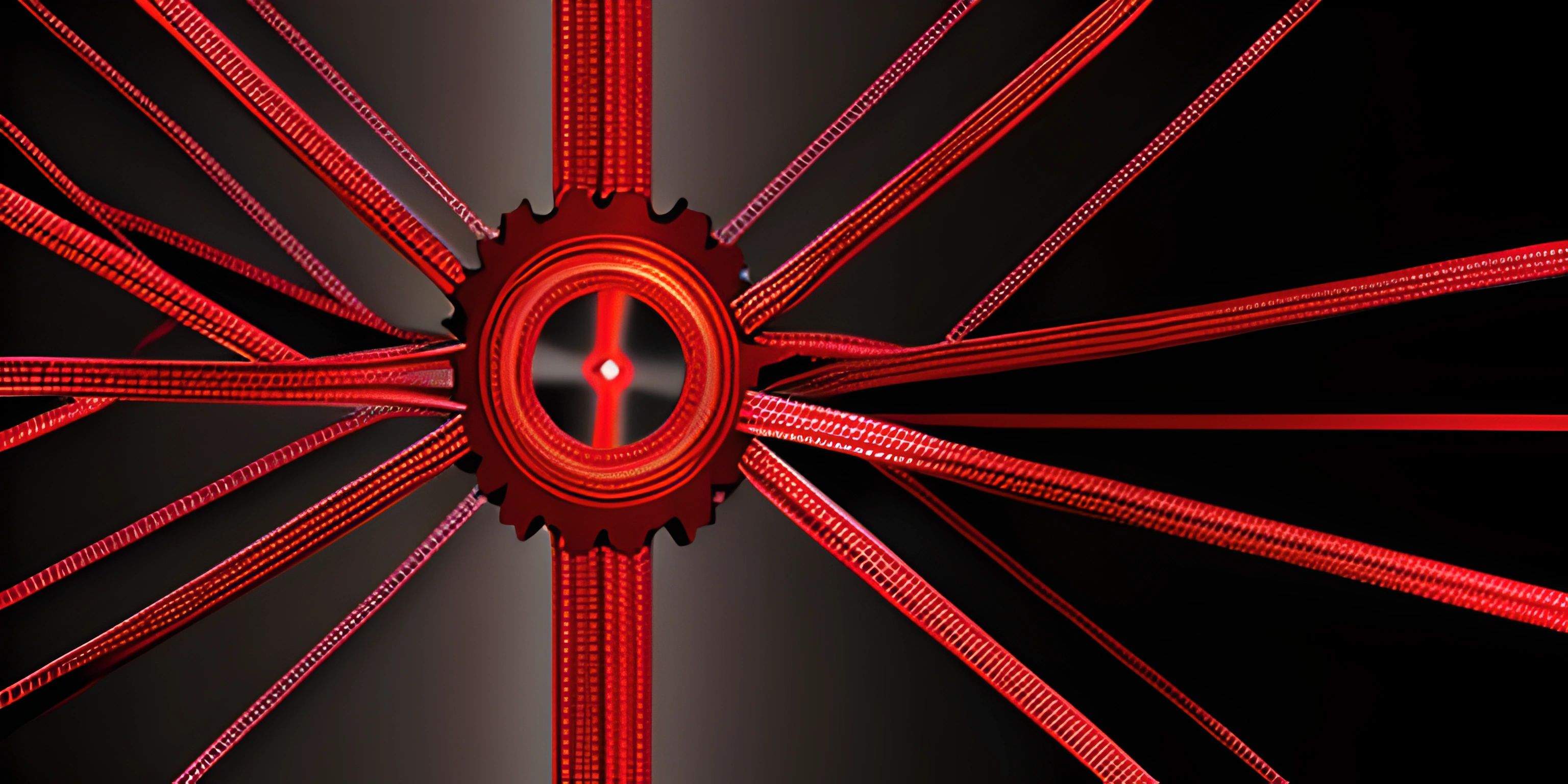
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
When programming in x86 NASM Assembly, arrays can be a vital tool to store and organize large amounts of data. Just like in any other programming language, arrays in Assembly are collections of elements, all of which share a common data type. Let's dive into how arrays can be implemented in x86 NASM Assembly!
Array Allocation
To allocate an array in x86 NASM Assembly, we need to first reserve some memory space. In Assembly, the section .bss
directive helps us reserve uninitialized memory. Here's an example of how to allocate an array of 10 integers (assuming each integer occupies 4 bytes):
section .bss myArray resd 10 ; Reserve space for 10 integers (each 4 bytes long)
Accessing Array Elements
Once we have an array, it's essential to know how to access its elements. In x86 NASM Assembly, we use the []
notation to access memory locations relative to a base address. The base address is the address of the first element, and we calculate the offset of the desired element by multiplying its index by the size of each element. For example, if we want to access the 5th element of myArray
:
mov eax, [myArray + 4 * 4] ; Access the 5th element (zero-based index)
Looping Through Arrays
Looping through the elements of an array is a common operation. In x86 NASM Assembly, we can use loops and index registers to achieve this. Let's say we want to sum all the elements of myArray
:
section .data arrayLength equ 10 ; Define the length of the array sum dd 0 ; Define a variable to store the sum section .text xor eax, eax ; Clear eax xor ecx, ecx ; Clear ecx (it will be our index register) sum_loop: add eax, [myArray + ecx * 4] ; Add the current array element to eax inc ecx ; Increment the index cmp ecx, arrayLength ; Compare the index with the array length jl sum_loop ; If index < arrayLength, jump back to the beginning of the loop ; At this point, eax contains the sum of all elements in the array mov [sum], eax ; Store the sum in the 'sum' variable
Now you have a basic understanding of arrays in x86 NASM Assembly, including how to allocate memory for arrays, access their elements, and loop through them. Keep practicing and experimenting with arrays to become more proficient in Assembly programming. Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Reverse Word Splitter (psst, it's free!).
FAQ
What is an array in x86 NASM Assembly?
In x86 NASM Assembly, an array is a continuous block of memory that stores multiple elements of the same data type, such as integers or characters. Arrays provide an efficient way to store and manage data in a program, as they allow for easy access and manipulation of these elements.
How do I allocate memory for an array in x86 NASM Assembly?
To allocate memory for an array in x86 NASM Assembly, you can use the times
directive. This directive repeats the specified data type a certain number of times, effectively creating an array. For example, to create an integer array with 5 elements, you can do the following:
section .data array: times 5 dw 0
This will allocate 5 times the size of a 2-byte word (double word) in memory, initializing each element to 0.
How can I access elements in an x86 NASM Assembly array?
You can access elements in an x86 NASM Assembly array by calculating the element's memory address based on its index within the array. To do this, you can use the base address of the array and add the product of the element's index and the size of an individual element. For example, to access the third element in a 2-byte word array:
mov ax, [array + 2 * 2]
This calculates the memory address of the third element (index 2) and loads its value into the AX register.
How do I loop through an array in x86 NASM Assembly?
To loop through an array in x86 NASM Assembly, you can use a combination of loop control instructions (such as loop
, jmp
, and labels) and array element access techniques. Here's a simple example of looping through a 5-element integer array and incrementing each element by 1:
section .data array: times 5 dw 0 section .text global _start _start: ; Initialize loop counter mov ecx, 5 loop_start: ; Load array element into AX register mov ax, [array + ecx * 2 - 2] ; Increment array element by 1 add ax, 1 ; Store updated value back into the array mov [array + ecx * 2 - 2], ax ; Decrement loop counter and jump to loop_start if not zero loop loop_start ; Exit the program mov eax, 1 xor ebx, ebx int 0x80
This example demonstrates how to set up a loop counter, access array elements, perform operations on them, and use the loop
instruction to control the loop flow.