x86 NASM Assembly Function Calls
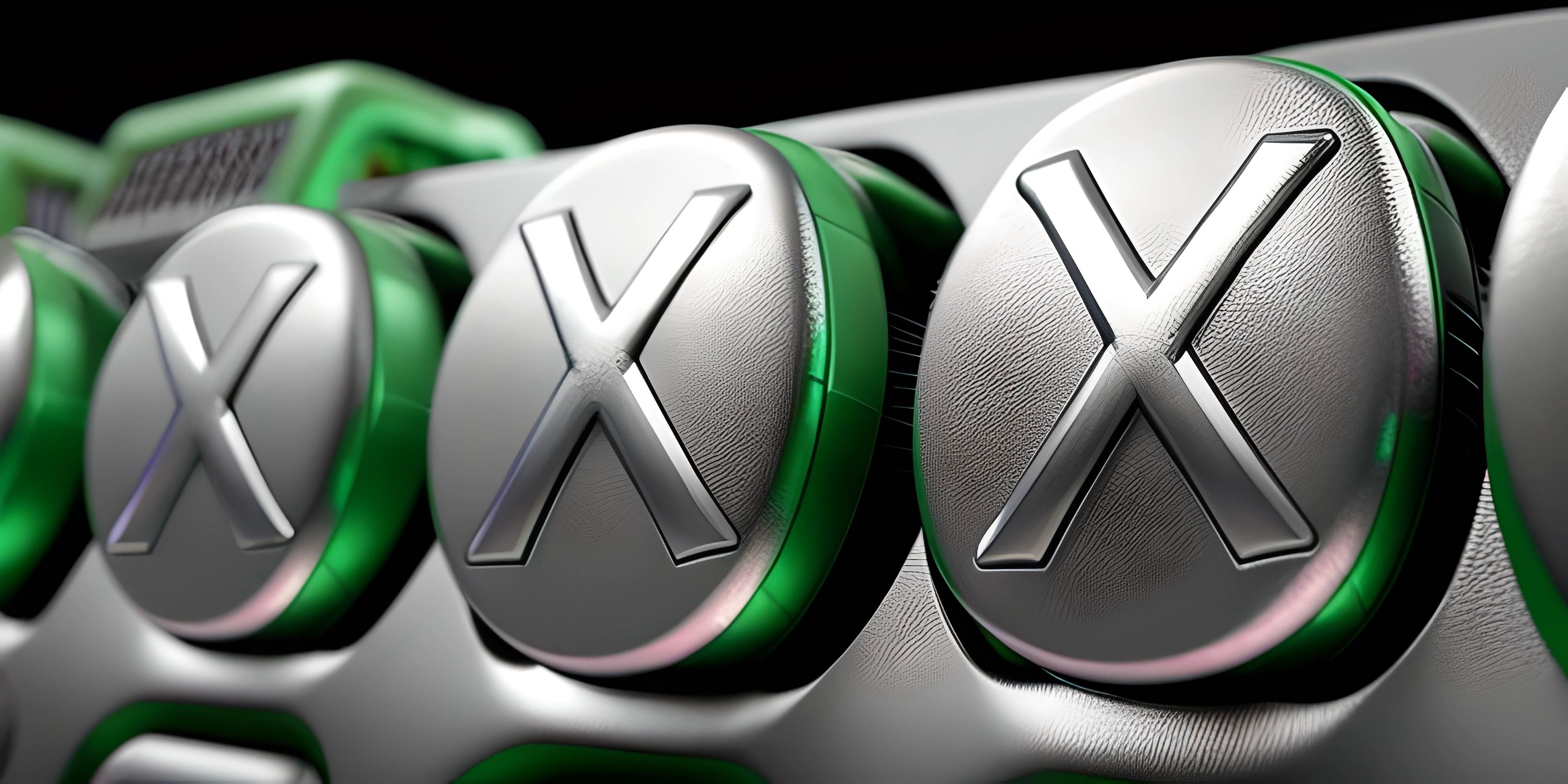
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
In x86 NASM assembly, function calls and argument passing are essential for creating modular, reusable code. In this article, we'll dive into the process of calling functions and passing arguments, making your x86 NASM assembly code more efficient and organized.
Function Call Basics
Functions, also called procedures or subroutines in assembly, are blocks of code that perform a specific task. Functions can be called from other parts of the code, allowing you to reuse code without duplicating it.
In x86 NASM assembly, the call
instruction is used to call a function. The function is typically labeled with a descriptive name indicating its purpose. After the function is executed, the ret
instruction returns to the instruction immediately following the call
.
Here's a simple example:
section .text global _start _start: call my_function ; rest of the code my_function: ; function body ret
Passing Arguments
When calling a function, you often need to pass arguments (also called parameters) to it. In x86 NASM assembly, arguments are typically passed using registers. The most common registers used for argument passing are eax
, ebx
, ecx
, and edx
. Here's an example:
section .text global _start _start: mov eax, 5 ; Argument 1 mov ebx, 10 ; Argument 2 call add_numbers ; eax now contains the sum of the two arguments add_numbers: add eax, ebx ; Add the two arguments ret
In this example, we pass two arguments (5 and 10) to the add_numbers
function, which adds them and stores the result in the eax
register.
Stack-based Argument Passing
Another way to pass arguments to a function is by using the stack. This is especially useful when you have more arguments than available registers or when you want to preserve register values. Here's an example of passing arguments using the stack:
section .text global _start _start: push 10 ; Argument 2 push 5 ; Argument 1 call add_numbers_stack add esp, 8 ; Clean up the stack ; eax now contains the sum of the two arguments add_numbers_stack: ; Get the arguments from the stack mov eax, [esp+4] ; Argument 1 mov ebx, [esp+8] ; Argument 2 add eax, ebx ; Add the two arguments ret
In this example, we push the arguments onto the stack before calling the add_numbers_stack
function. Inside the function, we use the esp
register to access the arguments on the stack and perform the addition. After the function call, we clean up the stack by adjusting the esp
register.
Remember that when using the stack for argument passing, you need to ensure the function call and the stack cleanup are balanced, or you may encounter issues with stack corruption or incorrect results.
Now that you've learned how to call functions and pass arguments in x86 NASM assembly, your code will be more modular, reusable, and easier to manage. Keep practicing these concepts to develop a deeper understanding of function calls and argument passing in assembly programming.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Recursion Intro (psst, it's free!).
FAQ
What is a function call in x86 NASM assembly?
A function call in x86 NASM assembly is a process of transferring control from one part of the code to another, usually to execute a specific subroutine or function. Functions are reusable code snippets that can be called multiple times throughout the program. In x86 NASM assembly, function calls are typically performed using the call
instruction, which pushes the return address onto the stack and jumps to the specified function label.
How do I pass arguments to a function in x86 NASM assembly?
To pass arguments to a function in x86 NASM assembly, you can use registers or the stack:
- Registers: You can pass arguments using general-purpose registers, like
eax
,ebx
,ecx
, andedx
. The caller function places the argument values into these registers before issuing thecall
instruction. The called function then retrieves the values from the registers.
mov eax, 5 mov ebx, 10 call my_function
- Stack: You can pass arguments by pushing them onto the stack before calling the function. The called function can access the values by referring to the appropriate stack offsets.
push 10 push 5 call my_function
Remember to clean up the stack after the function call if necessary.
How do I return a value from a function in x86 NASM assembly?
In x86 NASM assembly, you typically return a value from a function using the eax
register. The called function places the return value in the eax
register before executing the ret
instruction to return control to the caller. The caller can then access the return value in the eax
register.
my_function: ; ... perform calculations ... mov eax, result ret
How can I preserve register values while calling a function?
To preserve register values while calling a function in x86 NASM assembly, you can use the stack to store the contents of the registers before the function call, then restore the values after the function call. This process is known as "saving" and "restoring" registers.
; Save registers push eax push ebx ; Call function and pass arguments mov eax, 5 mov ebx, 10 call my_function ; Restore registers pop ebx pop eax
Can I use recursion in x86 NASM assembly functions?
Yes, you can use recursion in x86 NASM assembly functions, which involves a function calling itself. However, you must take care of saving and restoring the necessary registers and maintaining the stack properly to avoid overwriting data or causing a stack overflow. When using recursion, ensure that you have a base case to terminate the recursion, and make sure each recursive call is working towards that base case to avoid infinite loops.