x86 NASM Assembly Recursion Example
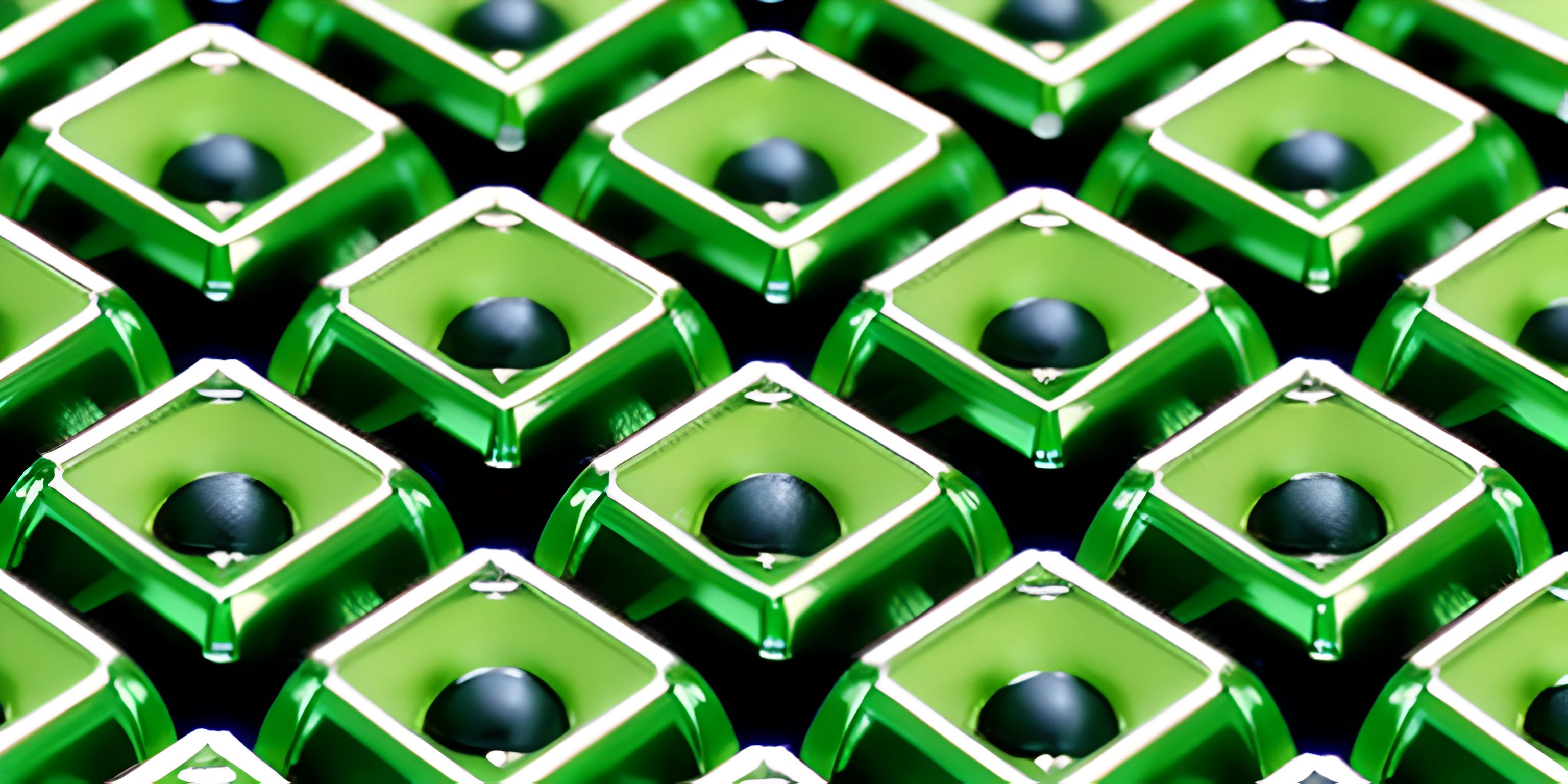
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Recursion is an important programming concept that can be quite powerful when used correctly. It's basically the idea of a function calling itself until it reaches a base condition. Today, we're going to dive into a simple example of recursion in x86 NASM Assembly language by creating a factorial function.
Factorial Function
Factorial, represented by n!
, is the product of all positive integers less than or equal to n
. For example, 5! = 5 × 4 × 3 × 2 × 1 = 120
. The base condition for a factorial function is 0! = 1
.
Let's see how we can create a factorial function using recursion in x86 NASM Assembly language.
section .data section .text global _start _start: ; Calculate the factorial of 5 (5! = 120) mov eax, 5 call factorial ; The result (120) is now stored in eax ; Exit the program mov eax, 1 xor ebx, ebx int 0x80 ; Factorial function using recursion factorial: ; Check if eax is 0 (base condition) cmp eax, 0 jz end_recursion ; Save the current value of eax push eax ; Decrement eax and call factorial function recursively dec eax call factorial ; Multiply the result returned in eax with the saved value of eax pop ebx imul eax, ebx ret end_recursion: ; Return 1 when eax is 0 (base condition) mov eax, 1 ret
This code calculates the factorial of 5
, and the result (120
) is stored in the eax
register. Let's break this code down step by step:
- We start by initializing the
eax
register with the value5
, which is the number we want to calculate the factorial of. - We call the
factorial
function. - Inside the
factorial
function, we first check ifeax
is0
. If it is, we jump to theend_recursion
label, seteax
to1
, and return. - If
eax
is not0
, we save the current value ofeax
by pushing it onto the stack. - We decrement
eax
and call thefactorial
function recursively. - After the recursion is complete, we pop the saved value of
eax
into theebx
register. - We multiply the result returned in
eax
with the saved value inebx
. - Finally, we return the result.
And there you have it, a simple example of recursion in x86 NASM Assembly language! Now you can impress your friends with your assembly-level knowledge of recursion and factorials!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Recursion Intro (psst, it's free!).
FAQ
What is recursion in x86 NASM Assembly language?
Recursion is a programming technique in which a function calls itself, typically to solve a smaller instance of the original problem. In x86 NASM Assembly language, recursion is implemented by creating a function that uses the call instruction to invoke itself.
Can you give a simple example of a recursive factorial function in x86 NASM Assembly?
Sure! Here's an example of a recursive factorial function in x86 NASM Assembly language:
section .data n db 5 ; The input number for the factorial section .text global _start _start: ; Load n into EAX mov eax, [n] ; Calculate factorial call factorial ; Exit mov eax, 1 int 0x80 factorial: ; Base case: if n == 1, return 1 cmp eax, 1 jne recursive_case ret recursive_case: ; Save current value of n push eax ; n = n - 1 dec eax ; Call factorial(n - 1) call factorial ; Multiply the result by the saved n value pop ebx mul ebx ; Return the result ret
How does the recursive function manage multiple calls without losing previous values?
In x86 NASM Assembly, the stack is used to store previous values of the function call. When a function calls itself, it pushes the current values of its arguments or local variables onto the stack. After the function call returns, it pops the saved values from the stack to restore the previous state, ensuring that the previous values are not lost.
Are there any limitations or drawbacks of using recursion in x86 NASM Assembly language?
Yes, recursion in x86 NASM Assembly language can have some drawbacks, such as:
- Increased memory usage: Since recursion uses the stack to store the previous values of function calls, it can consume a significant amount of memory for deep recursion.
- Limited stack size: The stack size is limited, and if the recursion depth exceeds the available stack space, it can lead to a stack overflow error.
- Performance: Recursion can be slower than iterative solutions, especially for large input values, due to the overhead of function calls and stack operations. To overcome these limitations, you can use techniques like memoization or convert the recursive solution into an iterative one.