ARM Assembly Introduction
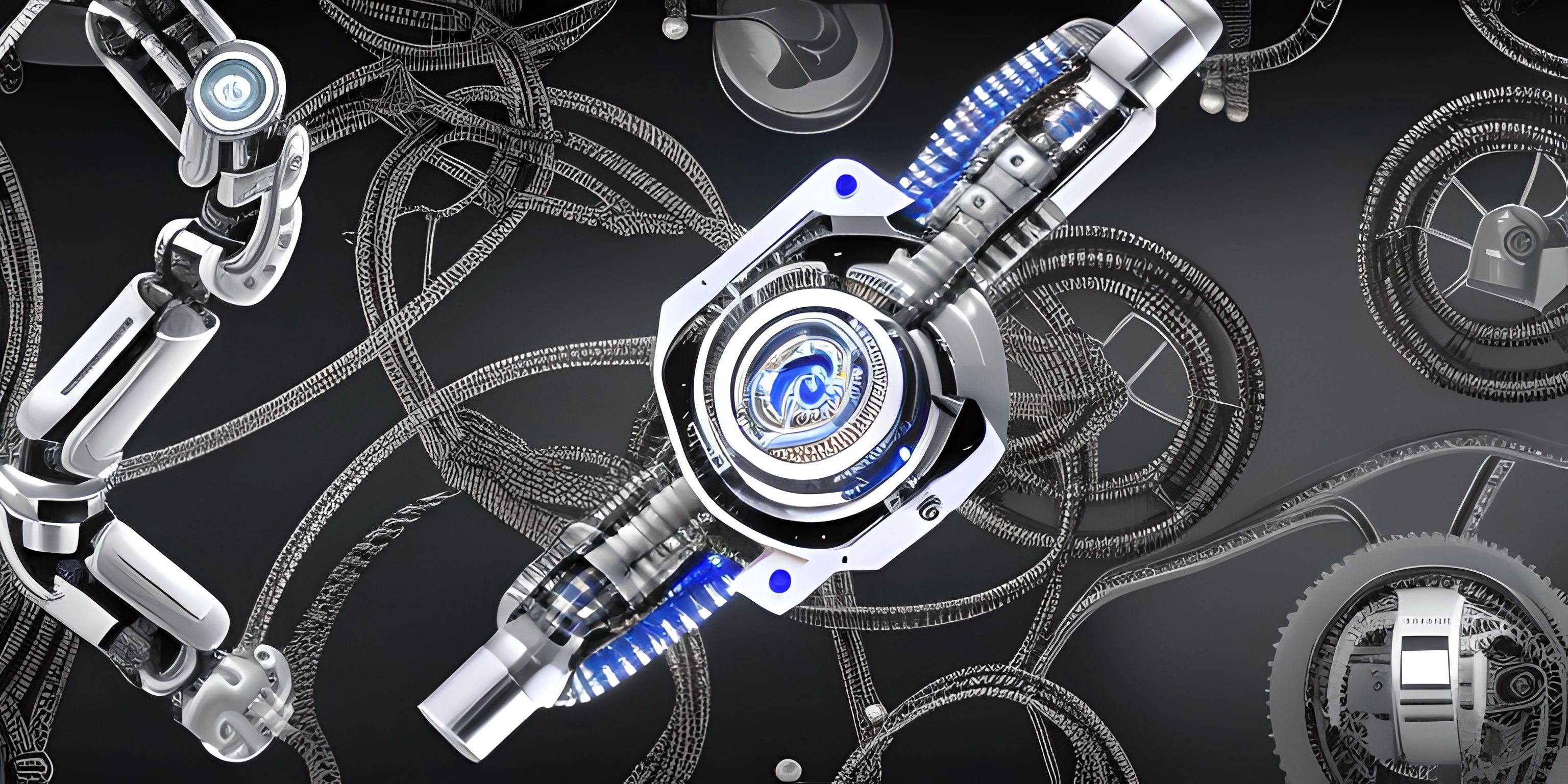
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
ARM Assembly is a low-level programming language specifically designed for ARM processors. ARM processors are widely used in mobile devices, embedded systems, and Internet of Things devices. Getting started with ARM Assembly can be an exciting journey into the inner workings of a computer, as it allows you to understand and manipulate the hardware directly.
Registers
In ARM Assembly, we work directly with the processor's registers. Registers are small, fast storage locations inside the processor that hold data and addresses. ARM processors typically have 16 registers, labeled R0 to R15. Some of these registers have specific purposes, such as the Program Counter (PC, R15) and Stack Pointer (SP, R13). The other registers can be used for general-purpose operations.
Instructions
ARM Assembly consists of a set of instructions that the processor can execute. These instructions are used to perform operations such as moving data between registers, performing arithmetic operations, and controlling the flow of the program. Some examples of ARM Assembly instructions include:
MOV
: Move data between registersADD
: Add two registers and store the result in a third registerSUB
: Subtract one register from another and store the result in a third registerLDR
: Load data from memory into a registerSTR
: Store data from a register into memory
Here's a simple example of ARM Assembly code that adds two numbers and stores the result in another register:
MOV R0, #5 ; Load the value 5 into register R0 MOV R1, #7 ; Load the value 7 into register R1 ADD R2, R0, R1; Add the values in R0 and R1, and store the result in R2
Addressing Modes
ARM Assembly provides various addressing modes for working with memory. Addressing modes determine how the processor accesses memory and how the address is calculated. Some common addressing modes include:
- Immediate: The value is directly specified in the instruction, such as
MOV R0, #5
. - Register: The address is stored in a register, such as
LDR R0, [R1]
. - Register Offset: The address is calculated by adding or subtracting an offset value from a register, such as
LDR R0, [R1, #4]
.
Conclusion
ARM Assembly language is a powerful way to interact directly with ARM processors, giving you a better understanding of how the hardware works. By learning the basics of registers, instructions, and addressing modes, you're well on your way to mastering this arcane art. Happy coding, and may your programs run swiftly and efficiently on the silicon steeds of the ARM realm!
FAQ
What is ARM Assembly language?
ARM Assembly language is a low-level programming language used to program ARM processors directly. It provides a human-readable way to interact with the processor's individual instructions and registers, allowing developers to write highly efficient and tailored code for specific hardware.
What are the basic concepts of ARM Assembly language?
The basic concepts of ARM Assembly language include:
- Registers: These are small, fast storage locations within the processor that hold data and address values.
- Instructions: These are the fundamental commands that tell the processor what to do, such as arithmetic operations, data movement, and branching.
- Addressing modes: These determine how the processor accesses data and instructions, either through registers or memory addresses.
What are some common ARM Assembly instructions?
Some common ARM Assembly instructions include:
MOV
: Move data from one register to anotherADD
: Add two values and store the result in a registerSUB
: Subtract one value from another and store the result in a registerLDR
: Load data from memory into a registerSTR
: Store data from a register to memoryB
: Branch to a specified address
How do I write a simple ARM Assembly program?
Here's an example of a simple ARM Assembly program that adds two numbers together and stores the result in a register:
MOV R0, #5 ; Move the value 5 into register R0 MOV R1, #3 ; Move the value 3 into register R1 ADD R2, R0, R1 ; Add the values in R0 and R1, and store the result in R2
This program moves the values 5 and 3 into registers R0 and R1, respectively, and then adds them together, storing the result (8) in register R2.
How can I learn more about ARM Assembly language?
To learn more about ARM Assembly language, you can refer to official ARM documentation, online tutorials, and textbooks dedicated to ARM Assembly programming. Additionally, practice and experimentation are key to mastering any programming language, so writing and analyzing existing ARM Assembly code can be incredibly helpful.