Assembly Instructions and Addressing Modes
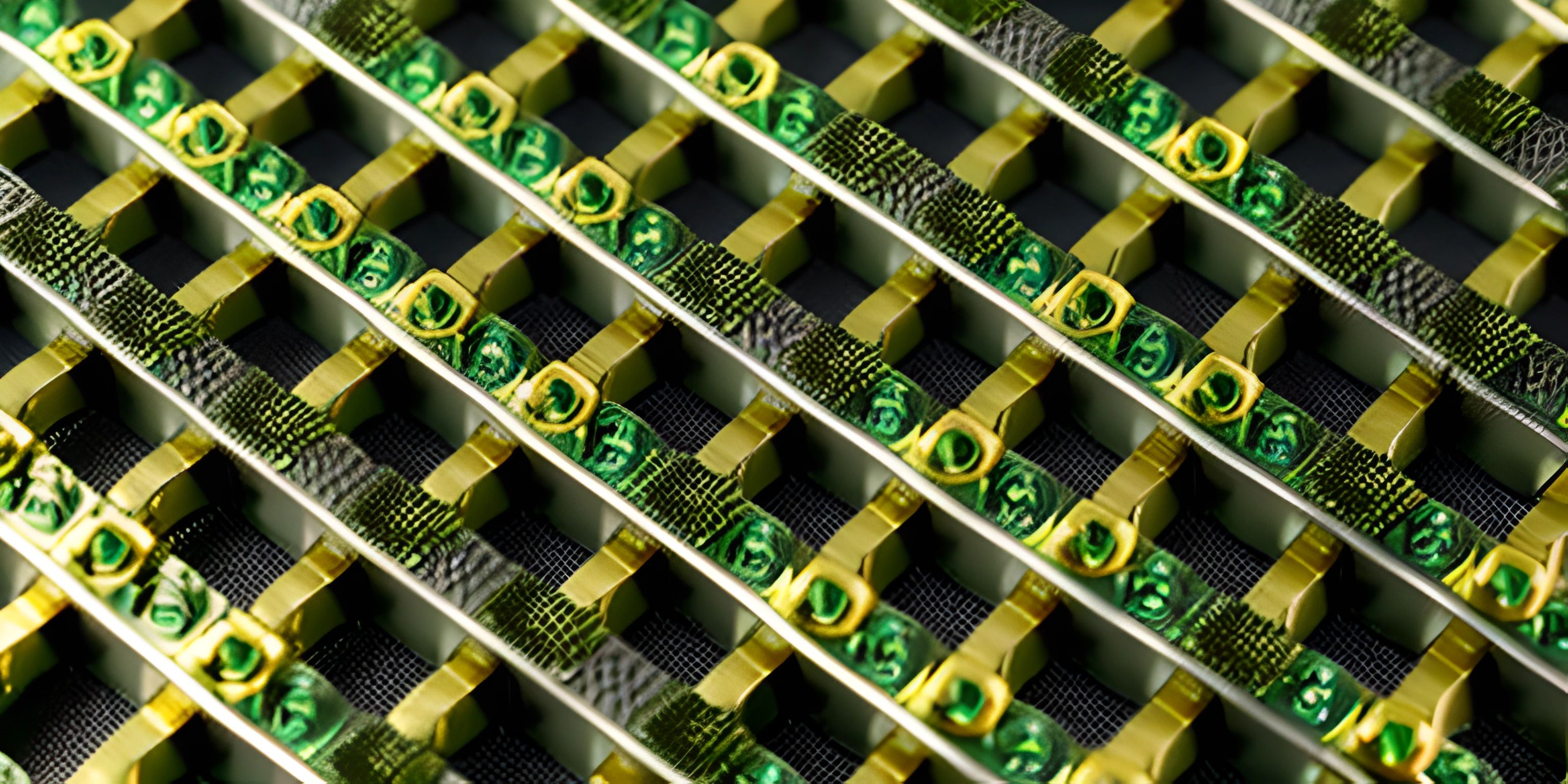
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Assembly Instructions
In the world of assembly programming, instructions are the basic building blocks that make our programs functional. These instructions are the low-level operations that the computer's hardware can understand and execute. Assembly programming is all about connecting these tiny operations to create more complex and useful programs.
Types of Instructions
Although assembly instructions can vary depending on the hardware, there are some common types that you'll encounter in most assembly languages:
- Data Movement - Instructions for moving data between registers or memory locations, such as
MOV
,LOAD
, andSTORE
. - Arithmetic and Logic - Instructions for performing arithmetic and logical operations, like
ADD
,SUB
,AND
, andOR
. - Control Flow - Instructions for altering the flow of the program, including jumps (
JMP
), branches (BNE
,BEQ
), and subroutine calls (CALL
,RET
).
Addressing Modes
Now that we know the basic types of assembly instructions, it's time to talk about how they access data. In assembly programming, addressing modes determine how the data is accessed or manipulated by an instruction.
Different assembly languages can have different addressing modes, but some common ones include:
- Immediate - The operand is a constant value, directly provided in the instruction. For example:
MOV R1, #10
(Move the value 10 into register R1). - Register - The operand is a register, which holds the data. For example:
ADD R2, R1
(Add the values in registers R1 and R2, and store the result in R2). - Direct - The operand is a memory address, specified directly in the instruction. For example:
LOAD R1, 0x1000
(Load the value at memory address 0x1000 into register R1). - Indirect - The operand is a register containing the memory address to access. For example:
STORE R1, [R2]
(Store the value in register R1 at the memory address stored in register R2). - Indexed - The operand is a combination of a base register and an offset value. For example:
LOAD R1, [R2 + 4]
(Load the value at the memory address equal to the value in R2 plus 4 into register R1).
Each of these addressing modes has its own advantages and uses in assembly programming. Understanding when to use each mode is crucial for writing efficient and performant assembly code.
Wrap Up
Combining assembly instructions and addressing modes, we can create powerful programs that directly interact with a computer's hardware. While assembly programming might seem intimidating at first, understanding the basics of assembly instructions and addressing modes is the key to unlocking its potential.
So, the next time you're diving into the world of assembly programming, remember these fundamental concepts to help guide you through the process like a seasoned pro!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: AI Test Playground (psst, it's free!).
FAQ
What are assembly instructions and why are they important in assembly programming?
Assembly instructions are fundamental elements of assembly programming. They are human-readable representations of machine-level operations, such as moving data, performing arithmetic, and controlling program flow. Assembly instructions are essential because they enable programmers to write code that directly interacts with the hardware, providing fine-grained control and optimization opportunities.
What are addressing modes and how do they affect assembly instructions?
Addressing modes are methods used by assembly instructions to access data stored in memory or registers. They define how operands (data values) are fetched and manipulated during the execution of an instruction. Different addressing modes provide various ways of specifying the location of data, offering flexibility and efficiency in assembly programming.
Can you provide examples of common addressing modes used in assembly programming?
Sure! Here are some common addressing modes used in assembly programming:
- Immediate mode: The operand is a constant value or an expression, e.g.,
MOV AX, 10
. - Register mode: The operand is stored in a register, e.g.,
ADD AX, BX
. - Direct mode: The operand is stored in a memory location, specified by its address, e.g.,
MOV AX, [1234]
. - Register indirect mode: The operand's address is stored in a register, e.g.,
MOV AX, [BX]
. - Indexed mode: The operand's address is calculated as the sum of a base address and an index value, e.g.,
MOV AX, [BX + SI]
.
How do I choose the right addressing mode for a specific assembly instruction?
Choosing the right addressing mode depends on the task and hardware constraints. Some factors to consider include:
- The type and location of the data you want to access.
- The available registers and their current values.
- The size and performance requirements of your code. Consider these factors and select the addressing mode that offers the most efficient and convenient way to access the data required for your instruction.
Can you write a simple assembly code example that uses different addressing modes?
Sure! Here's an example in x86 assembly that uses various addressing modes:
section .data array db 0, 1, 2, 3, 4 section .text global _start _start: ; Immediate mode mov eax, 4 ; Register mode mov ebx, eax ; Direct mode mov ecx, [array] ; Register indirect mode lea edi, [array] mov edx, [edi + 2] ; Indexed mode xor esi, esi mov al, [edi + esi] ; Exit the program mov eax, 1 xor ebx, ebx int 0x80
This example demonstrates how different addressing modes can be used in assembly programming to access data stored in memory and registers.