A Quick Introduction to x86 Assembly Language
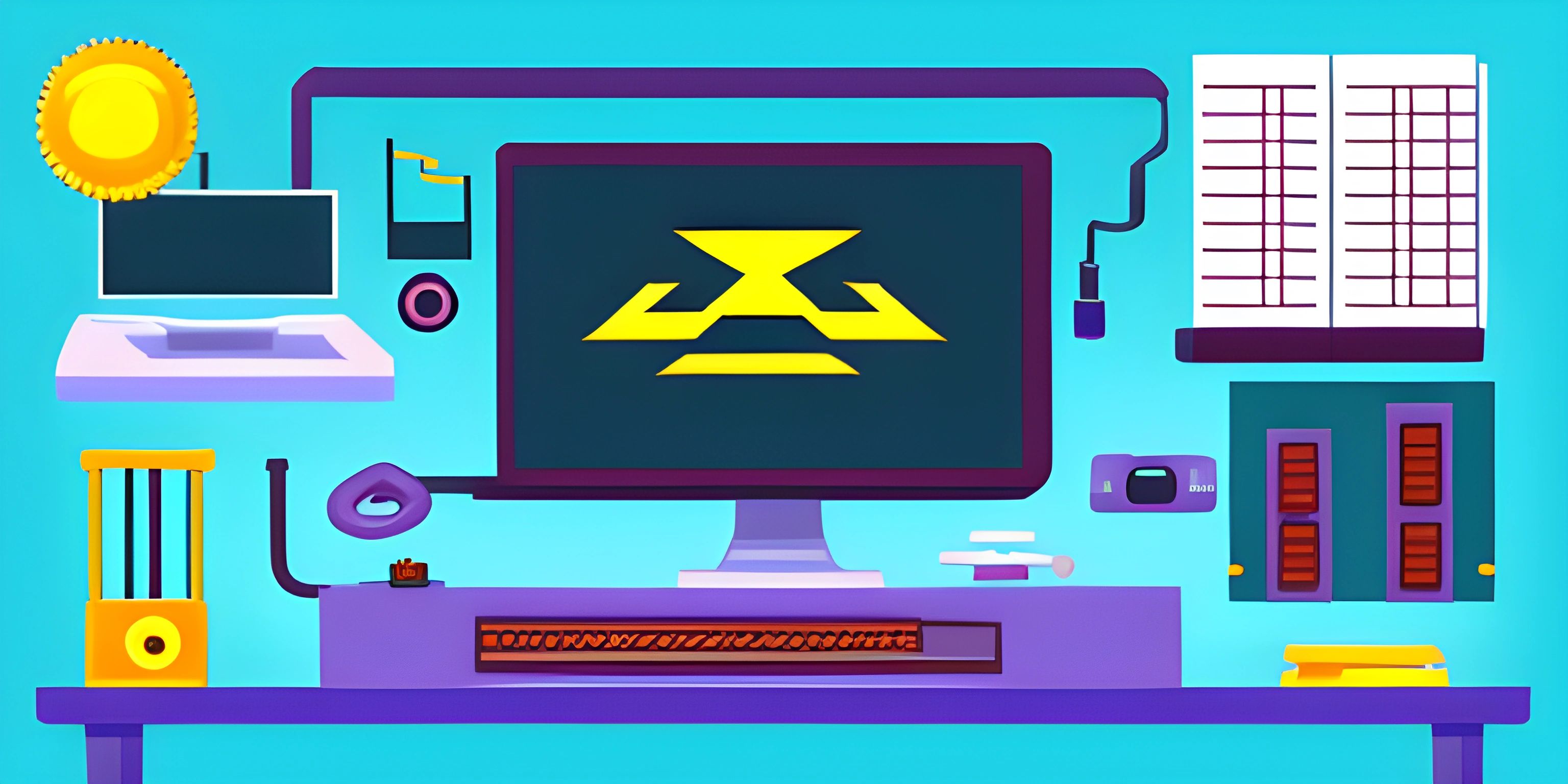
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Have you ever wondered what happens behind the scenes when you hit that "Run" button on your favorite high-level language? Buckle up, because we're about to dive into the nuts and bolts - quite literally - of programming with x86 assembly language. It's the kind of stuff that makes you feel like you’re talking directly to your computer, and in a way, you are!
What is Assembly Language?
Assembly language is like the secret code that your computer's CPU speaks. Unlike high-level languages, assembly is specific to a particular type of CPU architecture. In our case, we're looking at the x86 architecture - the same family of CPUs powering many of our computers today.
Registers: The CPU's Handy Dandy Notepads
Imagine your CPU as a chef in a busy kitchen. Registers are like the chef's notepads, where quick, temporary information is jotted down. x86 has several registers, each with its own special purpose. Here are a few key ones:
- EAX (Accumulator Register): Often used for arithmetic operations.
- EBX (Base Register): Can be used as a pointer to data.
- ECX (Counter Register): Used in loops and string operations.
- EDX (Data Register): Also used in arithmetic operations, often for multiplication and division.
Other important registers include ESI, EDI, EBP, and ESP. Knowing these is crucial for understanding how to manipulate data directly at the hardware level.
Instructions: The CPU's To-Do List
Programming in assembly is all about giving your CPU a to-do list, one instruction at a time. Each instruction is a simple operation, like moving data, performing arithmetic, or jumping to a different part of the program.
MOV Instruction
The MOV
instruction is like the bread and butter of assembly language. It moves data from one place to another:
MOV EAX, 5 ; Move the value 5 into the EAX register MOV EBX, EAX ; Copy the value in EAX to EBX
ADD and SUB Instructions
Want to do some basic math? The ADD
and SUB
instructions are your friends:
ADD EAX, 3 ; Add 3 to the value in EAX SUB EBX, 2 ; Subtract 2 from the value in EBX
JMP Instruction
Control flow is managed using instructions like JMP
, which tells the CPU to jump to a different part of the code. Think of it as a goto statement in other languages:
JMP label ; Jump to the location marked by 'label' label: ; More code here
Putting it All Together: A Simple Program
Let's write a simple x86 assembly program that adds two numbers and stores the result. Here's a step-by-step breakdown:
section .data num1 db 10 ; Define byte with value 10 num2 db 20 ; Define byte with value 20 section .bss result resb 1 ; Reserve a byte for the result section .text global _start _start: ; Load numbers into registers MOV AL, [num1] MOV BL, [num2] ; Add the numbers ADD AL, BL ; Store the result MOV [result], AL ; Exit the program MOV EAX, 1 ; System call for exit XOR EBX, EBX ; Exit code 0 INT 0x80 ; Interrupt to make the kernel execute the system call
In this program, we've defined two numbers in the .data
section, reserved a byte for the result in the .bss
section, and performed the addition in the .text
section. Finally, we used a system call to exit the program gracefully.
Understanding the Example
Why did we use AL
and BL
instead of EAX
and EBX
? AL
and BL
are the lower bytes of EAX
and EBX
, respectively. Since we are dealing with single-byte values, these are the appropriate registers to use.
Conclusion
Congratulations! You've just dipped your toes into the world of x86 assembly language. It's a world where you can control every tiny detail of what your CPU does. While it may seem daunting at first, mastering assembly can give you a deeper understanding of how computers work, making you a better programmer in any language. Remember, every journey begins with a single step - or in this case, a single MOV
instruction.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
Why should I learn x86 assembly language?
Learning x86 assembly language gives you a deeper understanding of how computers work at a low level. It can improve your debugging skills, help you write more efficient code, and make you appreciate the abstractions provided by high-level languages.
Is assembly language still relevant today?
Yes, assembly language is still relevant, especially in systems programming, embedded systems, and performance-critical applications. Knowing assembly can also help in reverse engineering and understanding how malware works.
How hard is it to learn assembly language?
Assembly language has a steep learning curve because it requires detailed knowledge of hardware and low-level operations. However, with practice and persistence, it becomes manageable. Starting with simple programs and gradually increasing complexity is a good approach.
What's the difference between x86 and x64 assembly?
x86 assembly is designed for 32-bit processors, while x64 assembly is for 64-bit processors. x64 assembly has more registers and can handle larger amounts of memory more efficiently. However, the basic principles and many instructions are similar.
Can I write complete programs in assembly language?
Yes, you can write complete programs in assembly language, but it's usually more efficient to use high-level languages for most applications. Assembly is often used for performance-critical code sections or situations where low-level hardware control is required.