Understanding and Implementing the Bellman-Ford Algorithm
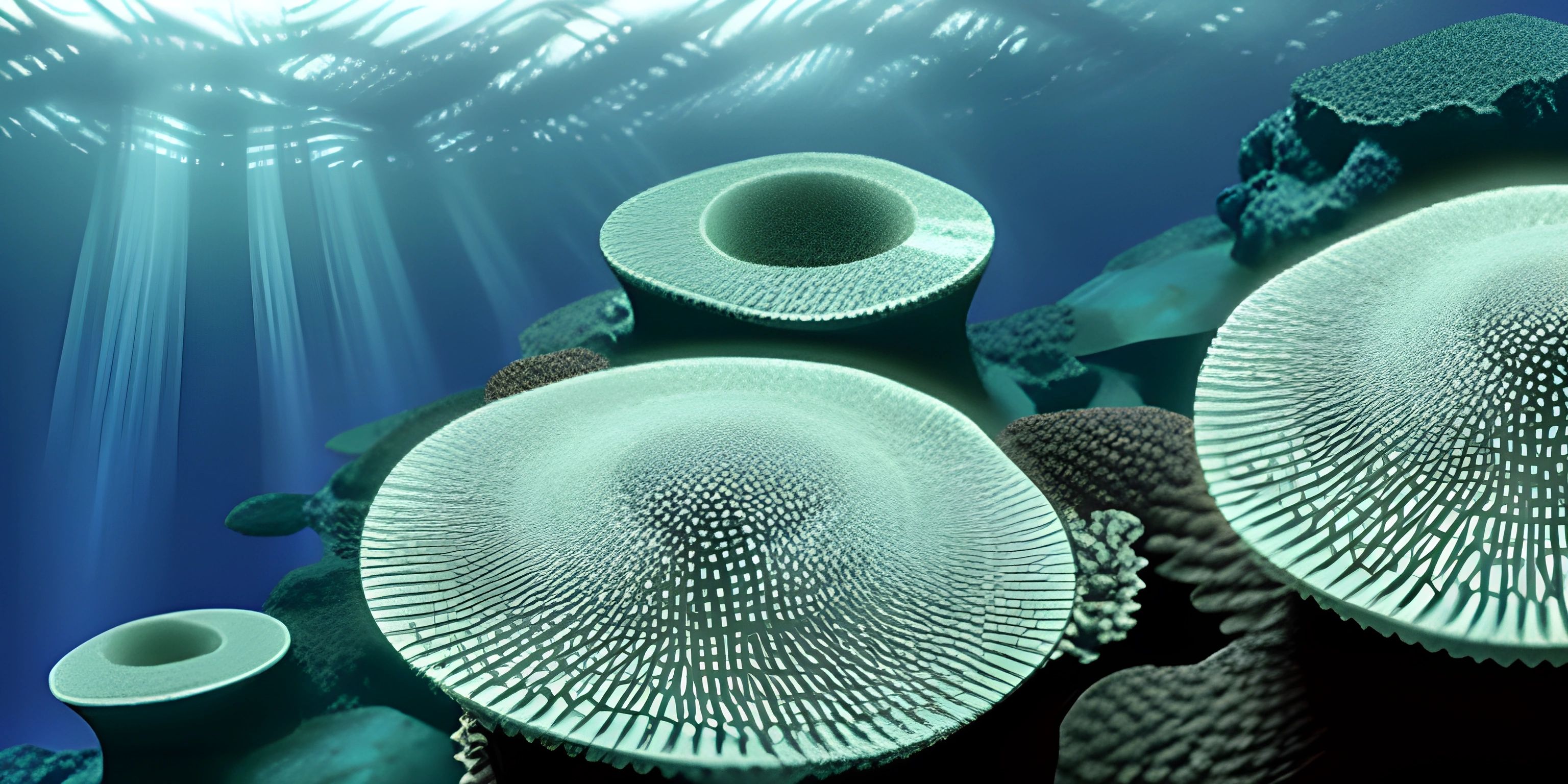
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
If you've ever been stuck in traffic and wished for the fastest route, then you've delved into the realm of shortest path algorithms, even if just in spirit. Today, we'll take a scenic drive through the Bellman-Ford algorithm, which, unlike some of its peers, can handle roads (or edges) with negative lengths (weights)! Buckle up, and let's hit the road.
Introduction to Bellman-Ford
The Bellman-Ford algorithm is a fundamental algorithm in graph theory used to find the shortest paths from a single source vertex to all other vertices in a weighted graph. Unlike Dijkstra's algorithm, Bellman-Ford can handle graphs with negative weights, making it a versatile tool in your algorithm toolkit.
Key Features:
- Handles Negative Weights: Unlike Dijkstra's algorithm, Bellman-Ford can manage edges with negative weights.
- Detects Negative Weight Cycles: If a graph contains a cycle with a negative total weight, Bellman-Ford can detect it.
- Complexity: It runs in O(V * E) time, where V is the number of vertices and E is the number of edges.
The Algorithm
Here’s a high-level overview of the steps involved in the Bellman-Ford algorithm:
- Initialization: Start by setting the distance to the source node as 0 and to all other nodes as infinity.
- Relaxation: Repeat V-1 times, where V is the number of vertices:
- For each edge (u, v) with weight w, if the distance to u plus w is less than the distance to v, update the distance to v.
- Check for Negative Weight Cycles: For each edge (u, v) with weight w, if the distance to u plus w is still less than the distance to v, a negative weight cycle exists.
Python Implementation
Let's dive into some code! Here's a Python implementation of the Bellman-Ford algorithm.
class Graph: def __init__(self, vertices): self.V = vertices self.edges = [] def add_edge(self, u, v, w): self.edges.append((u, v, w)) def bellman_ford(self, src): # Step 1: Initialize distances from src to all other vertices as INFINITE dist = [float("inf")] * self.V dist[src] = 0 # Step 2: Relax all edges |V| - 1 times. for _ in range(self.V - 1): for u, v, w in self.edges: if dist[u] != float("inf") and dist[u] + w < dist[v]: dist[v] = dist[u] + w # Step 3: Check for negative-weight cycles. for u, v, w in self.edges: if dist[u] != float("inf") and dist[u] + w < dist[v]: print("Graph contains negative weight cycle") return self.print_solution(dist) def print_solution(self, dist): print("Vertex Distance from Source") for i in range(self.V): print(f"{i}\t\t{dist[i]}") # Create a graph and add edges g = Graph(5) g.add_edge(0, 1, -1) g.add_edge(0, 2, 4) g.add_edge(1, 2, 3) g.add_edge(1, 3, 2) g.add_edge(1, 4, 2) g.add_edge(3, 2, 5) g.add_edge(3, 1, 1) g.add_edge(4, 3, -3) # Run Bellman-Ford algorithm from vertex 0 g.bellman_ford(0)
Explanation
- Graph Class: We define a
Graph
class to represent the graph. It initializes with the number of vertices and an empty list of edges. - Adding Edges: The
add_edge
method adds edges to the graph. - Bellman-Ford Method: The heart of the implementation. Initializes distances, performs relaxation, and checks for negative weight cycles.
- Print Solution: Outputs the shortest distances from the source to all vertices.
Running the Code
When you run this code, it will output the shortest distances from the source vertex (in this case, vertex 0) to all other vertices. If a negative weight cycle is detected, it will inform you.
Advantages and Disadvantages
Advantages:
- Handles Negative Weights: One of the few shortest path algorithms that can handle negative weights.
- Cycle Detection: Can detect negative weight cycles, which can be very useful in certain applications.
Disadvantages:
- Slower: Has a higher time complexity (O(V * E)) compared to Dijkstra's algorithm (O(V + E log V)).
- Inefficiency with Positive Weights: If your graph does not contain negative weights, Dijkstra's algorithm is more efficient.
Practical Uses
The Bellman-Ford algorithm is useful in various practical scenarios, such as:
- Routing Algorithms: In computer networks to find the shortest path.
- Financial Modeling: To detect arbitrage opportunities in currency exchange.
- Pathfinding: In games or simulations where negative weights might represent penalties.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Structs and Traits (psst, it's free!).
FAQ
What makes Bellman-Ford different from Dijkstra's algorithm?
The Bellman-Ford algorithm can handle negative weights and detect negative weight cycles, whereas Dijkstra's algorithm cannot. However, Bellman-Ford is generally slower.
Can the Bellman-Ford algorithm be used for undirected graphs?
Yes, but each edge should be added twice (once in each direction) because undirected edges can be traversed in both directions.
What happens if a graph contains a negative weight cycle?
The algorithm will detect it and report that the graph contains a negative weight cycle. In such cases, shortest paths are undefined.
How can I optimize the Bellman-Ford algorithm for better performance?
You can use techniques like early stopping if no distance update occurs during an iteration, indicating that all shortest paths have already been found.
What are some real-world applications of the Bellman-Ford algorithm?
The Bellman-Ford algorithm is used in network routing protocols, financial arbitrage detection, game development for pathfinding, and various optimization problems in operations research.