Functions in Programming
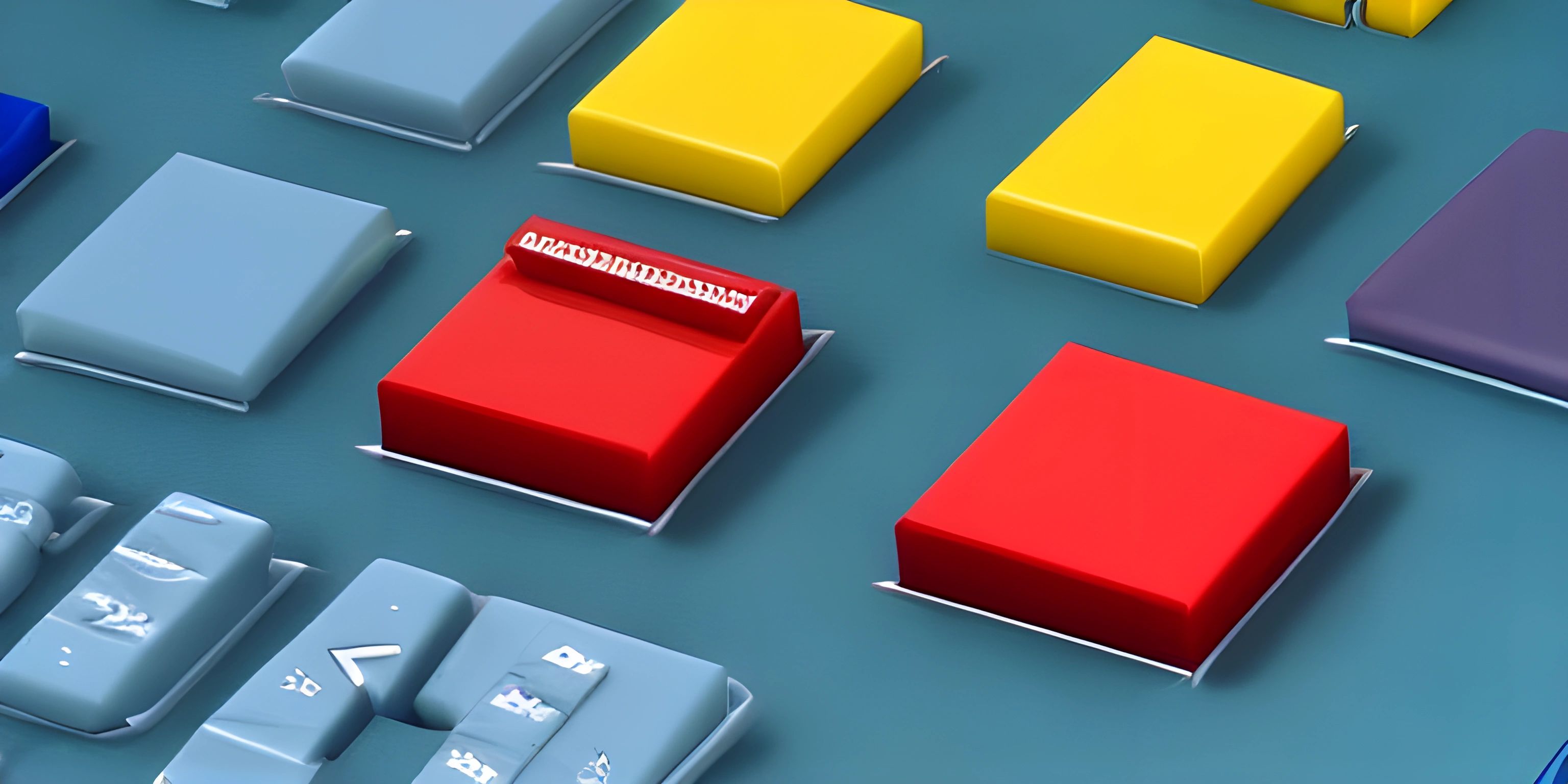
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Functions, sometimes called methods, are fundamental building blocks of programming. They allow you to package a set of instructions into a reusable unit that can be called multiple times throughout your program. Functions help to simplify code, promote modularity, and enable reusability. In other words, functions are like the Swiss Army knife of programming: versatile, compact, and indispensable.
Anatomy of a Function
A typical function consists of a few key components: a name, a list of parameters, a body, and a return value.
-
Name: The name is used to call the function in your code, and it should be descriptive of what the function does.
-
Parameters: Parameters are the input values that the function takes in when it's called. They act as placeholders for the actual values, called arguments, that will be used during the function call.
-
Body: The body of the function contains the code that will be executed when the function is called. This is where the magic happens.
-
Return Value: A function may optionally return a value as the result of its execution. This value can then be used elsewhere in your program.
Here's a simple example of a function in Python:
def greet(name): message = "Hello, " + name + "!" return message
In this example, greet
is the name of the function, and it takes a single parameter name
. The body of the function constructs a greeting message, and the return
statement sends the message back to the caller.
Calling Functions
To use a function, you need to call it by its name and pass the appropriate arguments for its parameters. Using the greet
function from above, we can call it as follows:
greeting = greet("Alice") print(greeting) # Output: "Hello, Alice!"
We pass the string "Alice" as an argument for the name
parameter, and the function returns the greeting message, which we then print out.
Functions Across Languages
The concept of functions is universal in programming, but their syntax and usage may differ between languages. Here's the same greet
function in JavaScript:
function greet(name) { const message = "Hello, " + name + "!"; return message; }
Despite minor syntactical differences, the core principles of functions remain the same across various programming languages.
Functions as First-Class Citizens
In some languages, like Python and JavaScript, functions are considered first-class citizens. This means that they can be assigned to variables, passed as arguments, and even returned from other functions. This opens up a world of possibilities for more advanced programming techniques, such as higher-order functions and closures.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Structs and Traits (psst, it's free!).
FAQ
What is a function in programming?
A function, also known as a method, is a reusable unit of code that packages a set of instructions. Functions simplify code, promote modularity, and enable reusability. They consist of a name, parameters, a body, and an optional return value.
What are the components of a function?
A typical function consists of the following components: a name, a list of parameters, a body, and a return value. The name is used to call the function, parameters are the input values, the body contains the code to be executed, and the return value is the result of the function's execution.
How do you call a function?
You call a function by using its name and passing the appropriate arguments for its parameters. The function then executes its body with the given arguments and, if applicable, returns a value.
Are functions used in all programming languages?
While the concept of functions is universal in programming, their syntax and usage may vary between languages. However, the core principles of functions remain consistent across different programming languages.
What does it mean for a function to be a first-class citizen?
In some programming languages, functions are considered first-class citizens, meaning they can be assigned to variables, passed as arguments, and returned from other functions. This allows for advanced programming techniques such as higher-order functions and closures.