Methods Deep Dive
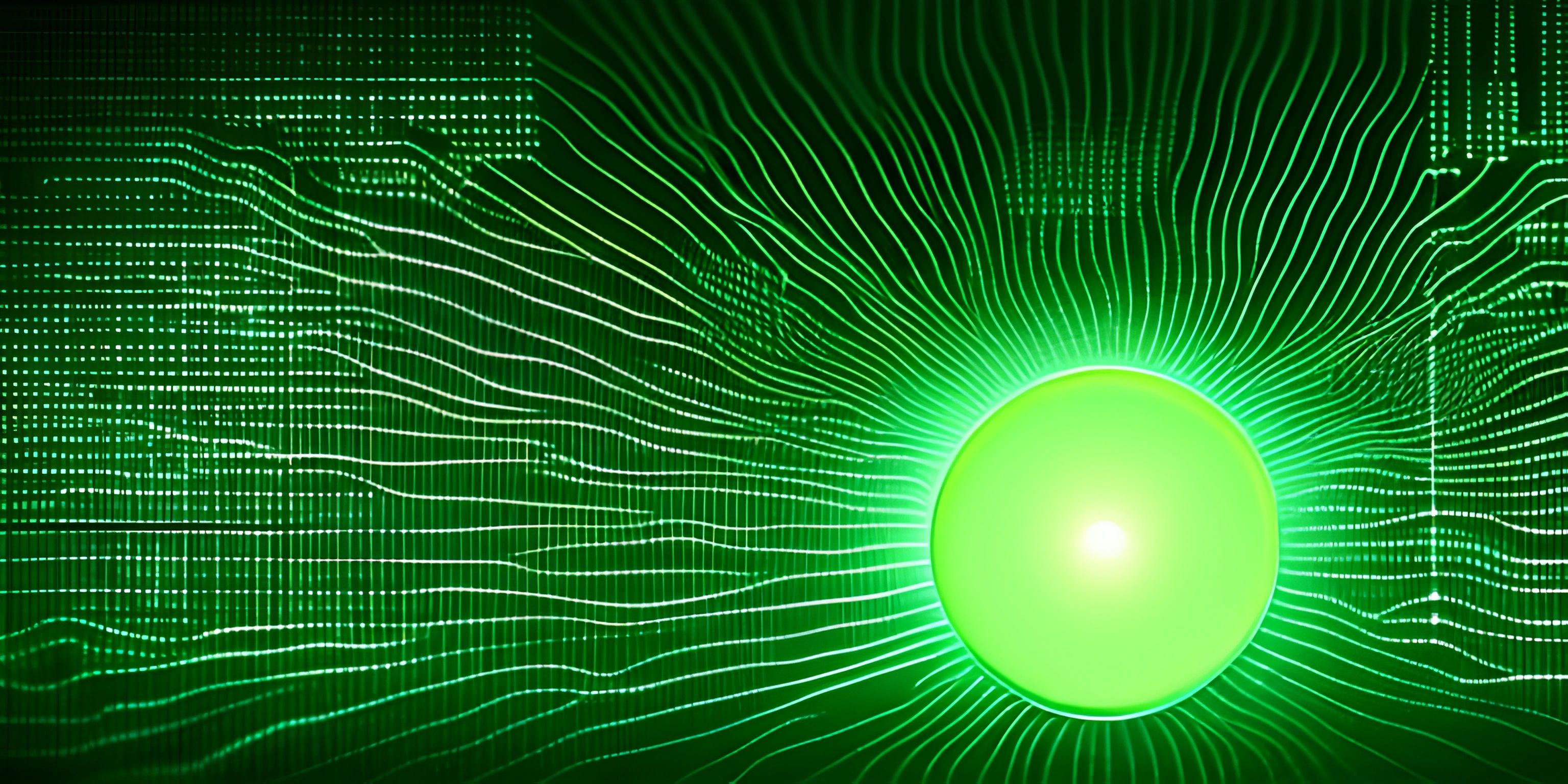
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Methods, sometimes called functions, are a fundamental building block of programming. They help us organize our code, promote reusability, and make it easier to understand the flow of a program. Get ready to dive into the wonderful world of methods!
What are Methods?
Methods are chunks of code that perform a specific task. They are reusable, meaning you can call them multiple times within your program to perform the same action without having to rewrite the same code. This makes your programs more efficient and easier to maintain.
Basic Anatomy of a Method
The basic structure of a method includes a name, a list of input parameters, and a return value. Here's an example of a simple method that takes two numbers, adds them together, and returns the result:
function add(a, b) { return a + b; }
In this example, add
is the method's name, a
and b
are its input parameters, and the return
keyword is used to specify the value that the method produces when called.
Calling a Method
To execute a method, you need to call it by writing its name, followed by a set of parentheses containing the input values (or arguments) you want to pass. For instance, if we want to call our add
method from above, we'd do it like this:
let result = add(5, 3);
This will call the add
method with the arguments 5
and 3
, and the result (8
) will be stored in the variable result
.
Why Methods are Helpful
Methods provide numerous benefits in programming, and here are some of the most noteworthy ones:
Code Reusability
One of the primary advantages of methods is code reusability. Instead of duplicating the same code in multiple places, you can write a method once and call it as many times as needed. This not only makes your code shorter and easier to manage but also reduces the chances of introducing errors.
Code Organization
Methods help you organize your code into logical, manageable units. By breaking a large program into smaller, more focused methods, you can make it easier to understand and maintain. It also allows you to concentrate on one aspect of your program at a time, letting you tackle complex problems one piece at a time.
Modularity
Methods promote modularity, allowing you to build programs by combining smaller, independent pieces. This makes it easier to test, debug, and modify your code. Plus, you can reuse these modular methods across multiple projects, saving time and effort in the long run.
Tips for Creating and Using Methods Effectively
To get the most out of methods, follow these best practices:
-
Choose meaningful names: Give your methods clear, descriptive names that indicate their purpose. This makes your code more readable and easier to understand.
-
Keep methods focused: Methods should perform a single, well-defined task. Avoid writing overly complex methods that try to do too much, as they can be hard to understand and maintain.
-
Don't Repeat Yourself (DRY): If you find yourself writing the same code in multiple places, consider creating a method to encapsulate that functionality, then call the method instead of duplicating the code.
-
Use parameters and return values wisely: Make good use of input parameters and return values to create flexible, reusable methods. Avoid using global variables or other side effects, as they can lead to hard-to-find bugs and make your code harder to understand.
So there you have it – a deep dive into the world of methods! By understanding how methods work and following best practices when creating and using them, you'll be well on your way to writing more efficient, organized, and maintainable code. Happy programming!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Basic Concepts (psst, it's free!).
FAQ
What are methods in programming and why are they helpful?
Methods, also known as functions or procedures, are blocks of code that perform a specific task. They are helpful in programming for several reasons:
- Reusability: Methods can be called multiple times, reducing code duplication.
- Modularity: Breaking down a complex task into smaller, manageable methods makes the code easier to understand and maintain.
- Abstraction: Encapsulating the implementation details within methods allows the rest of the code to focus on high-level logic.
How do you create a method in a programming language?
To create a method, you generally follow these steps:
- Define the method using a keyword (e.g., "def" in Python, "function" in JavaScript, or "Sub" in VBA).
- Give the method a descriptive name.
- Specify any input parameters or arguments the method requires.
- Write the code block that performs the method's task.
- Optionally, return a value from the method. Here's a simple example in Python:
def greet(name): return f"Hello, {name}!"
Can you provide an example of how to call a method in a programming language?
Sure! Here's an example using the greet
method we defined earlier:
# Call the greet method with the argument "Alice" greeting = greet("Alice") # Print the returned greeting print(greeting) # Output: "Hello, Alice!"
What are the differences between arguments and parameters in methods?
Parameters are the variables listed within the method definition's parentheses, while arguments are the actual values passed to the method when it's called. In the greet
method example, name
is a parameter, and in the method call greet("Alice")
, "Alice" is an argument.
How can I handle variable numbers of arguments in a method?
Many programming languages allow you to handle a variable number of arguments using special syntax. In Python, you can use the *args (for positional arguments) and **kwargs (for keyword arguments) conventions:
def example_method(*args, **kwargs): for arg in args: print(f"Positional argument: {arg}") for key, value in kwargs.items(): print(f"Keyword argument: {key} = {value}")