Cellular Automata in Generative Art
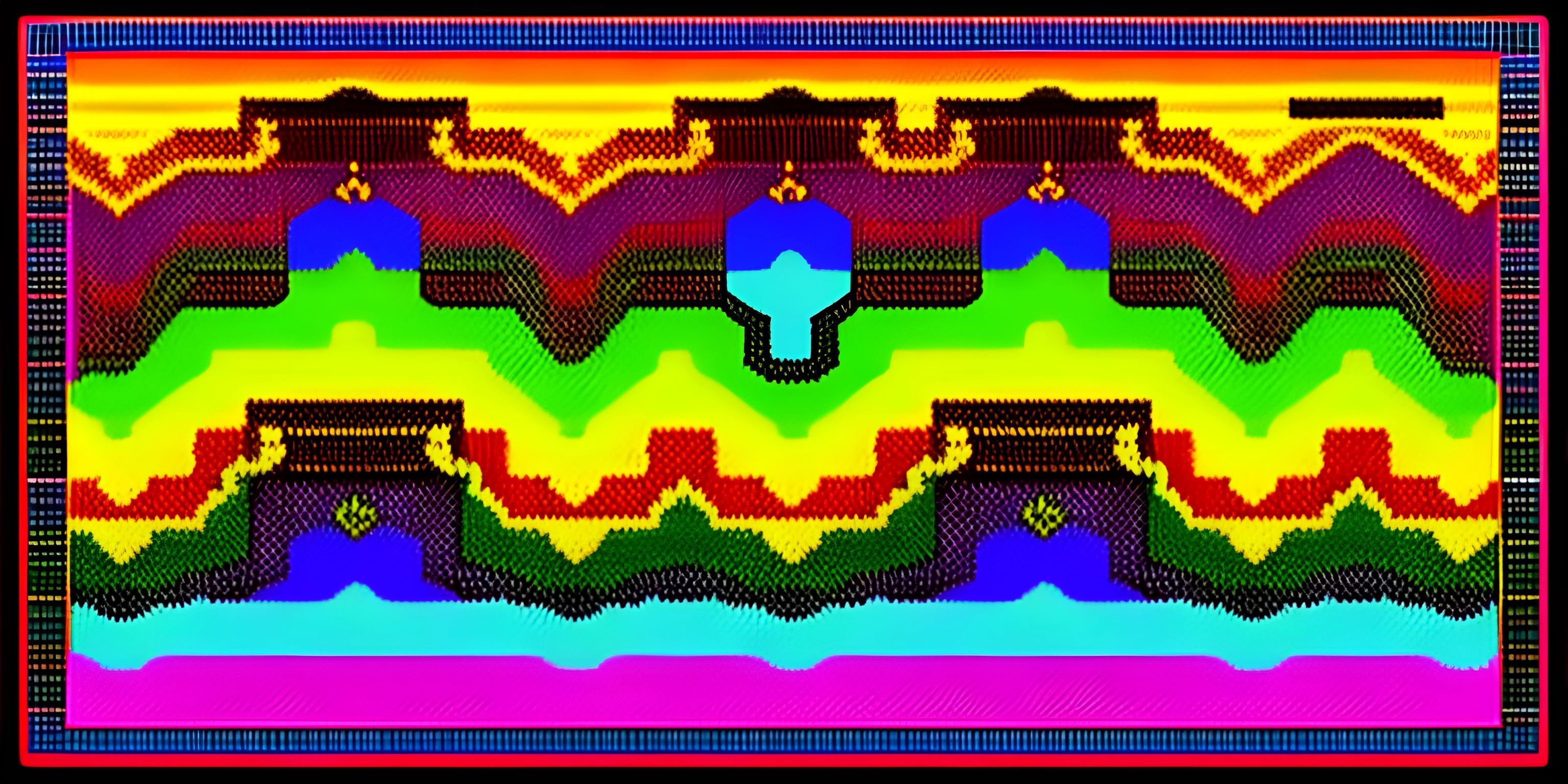
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Imagine a world where simple rules give rise to intricate patterns, complex structures, and stunning visuals. Welcome to the enchanting realm of cellular automata! These tiny computation units can create mesmerizing generative art, bridging the worlds of mathematics, programming, and art. Buckle up as we dive into this fascinating intersection.
What Are Cellular Automata?
At their core, cellular automata (CA) are computational models that consist of a grid of cells. Each cell can be in one of a finite number of states, and the state of a cell at the next time step is determined by its current state and the states of its neighbors. This simple concept can lead to remarkably complex behavior.
The Basic Elements
- Grid: The universe of cellular automata is a grid of cells, which can be one-dimensional, two-dimensional, or even higher-dimensional.
- Cells: Each cell in the grid can be in one of a finite number of states. For instance, a cell might be "alive" or "dead" in the famous Game of Life.
- Neighborhood: The neighborhood of a cell consists of the cells surrounding it. The most common neighborhoods are the von Neumann neighborhood (up, down, left, right) and the Moore neighborhood (all eight surrounding cells in a 2D grid).
- Rule: The rule defines how the state of each cell is updated based on its current state and the states of its neighbors.
The Rules of the Game
The heart of cellular automata lies in their rules. One of the simplest and most famous examples is Conway's Game of Life. The rules are:
- Any live cell with fewer than two live neighbors dies (underpopulation).
- Any live cell with two or three live neighbors lives on to the next generation.
- Any live cell with more than three live neighbors dies (overpopulation).
- Any dead cell with exactly three live neighbors becomes a live cell (reproduction).
These rules, though simple, can generate a wide range of complex patterns, from stable structures to oscillators and even patterns that replicate.
Generative Art with Cellular Automata
Now, let's explore how these simple rules can be harnessed to create stunning generative art.
Setting Up the Canvas
Before we dive into coding, let’s imagine our grid as a canvas where each cell can be a different color. The state of a cell could determine its color, allowing us to create beautiful, evolving patterns.
Let's start with a basic setup in Python using the Pygame library:
import pygame import numpy as np # Initialize Pygame pygame.init() # Screen dimensions width, height = 800, 800 screen = pygame.display.set_mode((width, height)) pygame.display.set_caption("Cellular Automata Art") # Grid dimensions cols, rows = 100, 100 cell_size = width // cols # Initialize grid with random states grid = np.random.randint(2, size=(cols, rows)) # Colors alive_color = (255, 255, 255) # White dead_color = (0, 0, 0) # Black def draw_grid(): for x in range(cols): for y in range(rows): color = alive_color if grid[x, y] == 1 else dead_color rect = pygame.Rect(x * cell_size, y * cell_size, cell_size, cell_size) pygame.draw.rect(screen, color, rect) # Main loop running = True while running: for event in pygame.event.get(): if event.type == pygame.QUIT: running = False draw_grid() pygame.display.flip() pygame.quit()
This code sets up a basic grid where each cell is either alive (white) or dead (black), and it displays the initial random configuration. Next, we'll implement the rules of cellular automata to evolve the grid over time.
Implementing the Evolution Rules
We’ll update each cell based on the states of its neighbors. Here's how you can implement Conway's Game of Life:
def update_grid(): global grid new_grid = np.copy(grid) for x in range(cols): for y in range(rows): # Get the number of alive neighbors alive_neighbors = np.sum(grid[(x-1):(x+2), (y-1):(y+2)]) - grid[x, y] if grid[x, y] == 1: # Apply the rules of the Game of Life if alive_neighbors < 2 or alive_neighbors > 3: new_grid[x, y] = 0 else: if alive_neighbors == 3: new_grid[x, y] = 1 grid = new_grid # Main loop with grid update running = True while running: for event in pygame.event.get(): if event.type == pygame.QUIT: running = False update_grid() draw_grid() pygame.display.flip() pygame.time.delay(100) pygame.quit()
Experimenting with Colors and Patterns
To add more artistic flair, we can experiment with different colors and states. For example, we could use a gradient of colors instead of just black and white, or we could introduce more states to create intricate patterns.
# Colors for different states colors = [(0, 0, 0), (255, 0, 0), (0, 255, 0), (0, 0, 255)] def draw_grid(): for x in range(cols): for y in range(rows): color = colors[grid[x, y]] rect = pygame.Rect(x * cell_size, y * cell_size, cell_size, cell_size) pygame.draw.rect(screen, color, rect) # Initialize grid with more states grid = np.random.randint(4, size=(cols, rows))
Cellular Automata Beyond Conway's Game of Life
There are countless variations of cellular automata, each with its unique rules and behaviors. Some famous examples include:
- Elementary Cellular Automata: These are one-dimensional CAs with the simplest possible neighborhood. Rule 30 and Rule 110 are well-known examples.
- Langton's Ant: A two-dimensional Turing machine with a very simple set of rules that can produce complex, chaotic patterns.
- Brian’s Brain: A cellular automaton that mimics the behavior of neurons.
Exploring these variations can lead to endless possibilities for generative art.
Conclusion
Cellular automata offer a fascinating way to create generative art through simple rules that lead to complex behaviors. By experimenting with different rules, colors, and patterns, you can create your own mesmerizing artworks. So, grab your code editor and start exploring the beautiful world of cellular automata!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Making Art with Code (psst, it's free!).
FAQ
What is cellular automata?
Cellular automata are computational models consisting of a grid of cells, where each cell changes state based on a set of rules and the states of its neighbors. These models can create complex patterns from simple rules.
How does Conway's Game of Life work?
Conway's Game of Life is a cellular automaton where each cell can be alive or dead. The state of each cell is updated based on the number of alive neighbors it has, following specific rules for survival, death, and reproduction.
Can cellular automata be used for art?
Yes, cellular automata can be used to create stunning generative art by visualizing the states of cells with different colors and patterns. The evolving behavior of the cells can produce intricate and beautiful designs.
What are some variations of cellular automata?
Some variations include Elementary Cellular Automata, Langton's Ant, and Brian’s Brain. Each variation has its unique set of rules and can produce different patterns and behaviors.
What tools can I use to create cellular automata art?
You can use programming languages like Python with libraries such as Pygame for visualizing cellular automata. Other tools include Processing and JavaScript with libraries like p5.js for creating generative art.