Conway's Game of Life in Generative Art
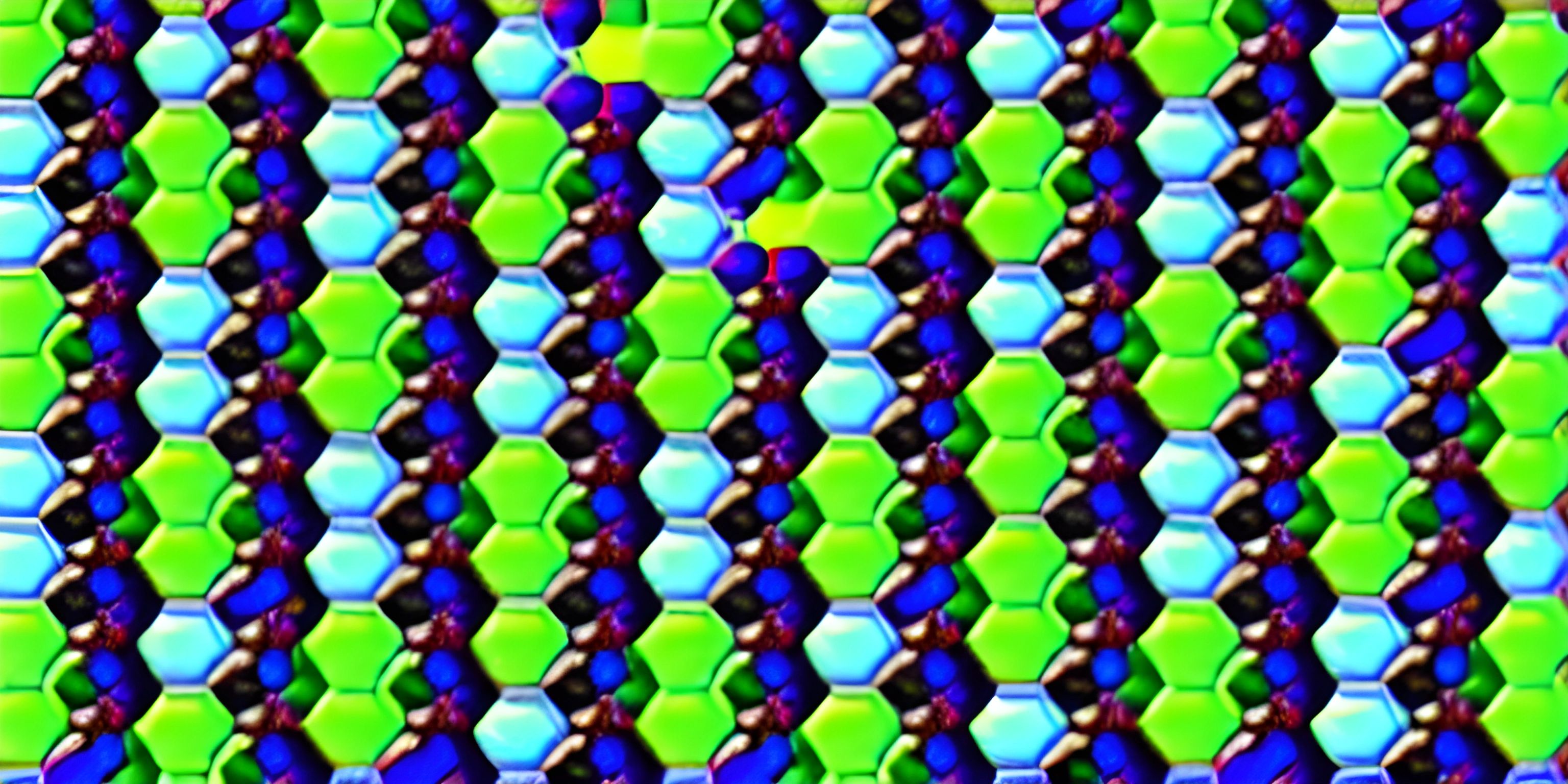
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Imagine a universe as vast as the night sky, yet contained within the confines of a computer screen. Welcome to Conway's Game of Life, a mesmerizing simulation that blends mathematics, art, and a dash of chaos. Invented by the British mathematician John Conway in 1970, this zero-player game demonstrates how simple rules can lead to complex patterns and behaviors.
But wait, there's more! By leveraging these captivating patterns, you can create generative art—artwork created algorithmically that evolves and changes over time. Intrigued? Let's dive in.
The Rules of Life
Conway's Game of Life operates on a grid of cells, each of which can be either alive or dead. The state of each cell changes from one generation to the next based on these rules:
- Underpopulation: A live cell with fewer than two live neighbors dies.
- Survival: A live cell with two or three live neighbors lives on to the next generation.
- Overpopulation: A live cell with more than three live neighbors dies.
- Reproduction: A dead cell with exactly three live neighbors becomes a live cell.
These simple rules can generate incredibly complex patterns. Let's see an example in Python.
import numpy as np import matplotlib.pyplot as plt # Initialize grid width, height = 50, 50 grid = np.random.choice([0, 1], size=(width, height)) def update(grid): new_grid = grid.copy() for i in range(grid.shape[0]): for j in range(grid.shape[1]): # Calculate number of alive neighbors total = int((grid[i, (j - 1) % height] + grid[i, (j + 1) % height] + grid[(i - 1) % width, j] + grid[(i + 1) % width, j] + grid[(i - 1) % width, (j - 1) % height] + grid[(i - 1) % width, (j + 1) % height] + grid[(i + 1) % width, (j - 1) % height] + grid[(i + 1) % width, (j + 1) % height])) # Apply Conway's rules if grid[i, j] == 1: if (total < 2) or (total > 3): new_grid[i, j] = 0 else: if total == 3: new_grid[i, j] = 1 return new_grid # Visualization plt.imshow(grid, cmap='binary') plt.title('Initial State') plt.show() for _ in range(100): grid = update(grid) plt.imshow(grid, cmap='binary') plt.title('Next Generation') plt.show()
From Cellular Automata to Generative Art
Now that we know the basics, let's see how to turn these patterns into stunning generative art. The key idea is to use the evolving grid states to generate visuals, which can be colored, animated, and transformed in various ways.
Colorizing the Grid
Instead of binary (black-and-white) grids, we can use color to add an artistic flair. Here's how to add a splash of color:
import numpy as np import matplotlib.pyplot as plt import matplotlib.colors as mcolors # Initialize grid width, height = 50, 50 grid = np.random.choice([0, 1], size=(width, height)) def update(grid): new_grid = grid.copy() for i in range(grid.shape[0]): for j in range(grid.shape[1]): total = int((grid[i, (j - 1) % height] + grid[i, (j + 1) % height] + grid[(i - 1) % width, j] + grid[(i + 1) % width, j] + grid[(i - 1) % width, (j - 1) % height] + grid[(i - 1) % width, (j + 1) % height] + grid[(i + 1) % width, (j - 1) % height] + grid[(i + 1) % width, (j + 1) % height])) if grid[i, j] == 1: if (total < 2) or (total > 3): new_grid[i, j] = 0 else: if total == 3: new_grid[i, j] = 1 return new_grid def colorize(grid): colors = ["black", "red", "green", "blue", "yellow"] cmap = mcolors.ListedColormap(colors) return cmap(grid) # Visualization plt.imshow(colorize(grid)) plt.title('Initial State') plt.show() for _ in range(100): grid = update(grid) plt.imshow(colorize(grid)) plt.title('Next Generation') plt.show()
Animating the Evolution
We can create an animation to visualize the evolution of the grid over time. This can be done using matplotlib.animation
.
import numpy as np import matplotlib.pyplot as plt import matplotlib.animation as animation # Initialize grid width, height = 50, 50 grid = np.random.choice([0, 1], size=(width, height)) def update(grid): new_grid = grid.copy() for i in range(grid.shape[0]): for j in range(grid.shape[1]): total = int((grid[i, (j - 1) % height] + grid[i, (j + 1) % height] + grid[(i - 1) % width, j] + grid[(i + 1) % width, j] + grid[(i - 1) % width, (j - 1) % height] + grid[(i - 1) % width, (j + 1) % height] + grid[(i + 1) % width, (j - 1) % height] + grid[(i + 1) % width, (j + 1) % height])) if grid[i, j] == 1: if (total < 2) or (total > 3): new_grid[i, j] = 0 else: if total == 3: new_grid[i, j] = 1 return new_grid def colorize(grid): colors = ["black", "red", "green", "blue", "yellow"] cmap = mcolors.ListedColormap(colors) return cmap(grid) fig, ax = plt.subplots() def animate(i): global grid grid = update(grid) ax.clear() ax.imshow(colorize(grid)) ax.set_title(f"Generation {i+1}") ani = animation.FuncAnimation(fig, animate, frames=100, interval=100) plt.show()
Beyond the Basics
Using the fundamental principles of Conway's Game of Life, the possibilities for creative expression are endless. You can experiment with:
- Different Rulesets: Try varying the rules for more diverse patterns.
- Interactive Art: Allow users to draw initial states and see how they evolve.
- Layering: Overlay multiple grids to create complex textures.
By understanding the intersection of algorithms and art, you unlock a world of innovation, turning mathematical principles into visual masterpieces.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Why Program? (psst, it's free!).
FAQ
What is Conway's Game of Life?
Conway's Game of Life is a cellular automaton devised by mathematician John Conway. It consists of a grid of cells that evolve according to simple rules, creating complex patterns over time.
How can I get started with generative art using Conway's Game of Life?
Start by understanding the basic rules and implementation of the game. Experiment with visualizing the grid using colors and animations to bring the patterns to life.
Can I use different rules other than Conway's original rules?
Yes, you can experiment with different rulesets to see how they affect the patterns and complexity of the resulting art.
What tools are necessary for creating generative art with Conway's Game of Life?
You'll need a programming environment (Python is a good choice), libraries like NumPy and Matplotlib for grid manipulation and visualization, and some creativity to experiment with patterns and colors.
Is Conway's Game of Life used in any real-world applications?
While primarily a mathematical curiosity and a tool for creating generative art, concepts from Conway's Game of Life have been used in computer science, theoretical biology, and the study of complex systems.