Complex Numbers in JavaScript
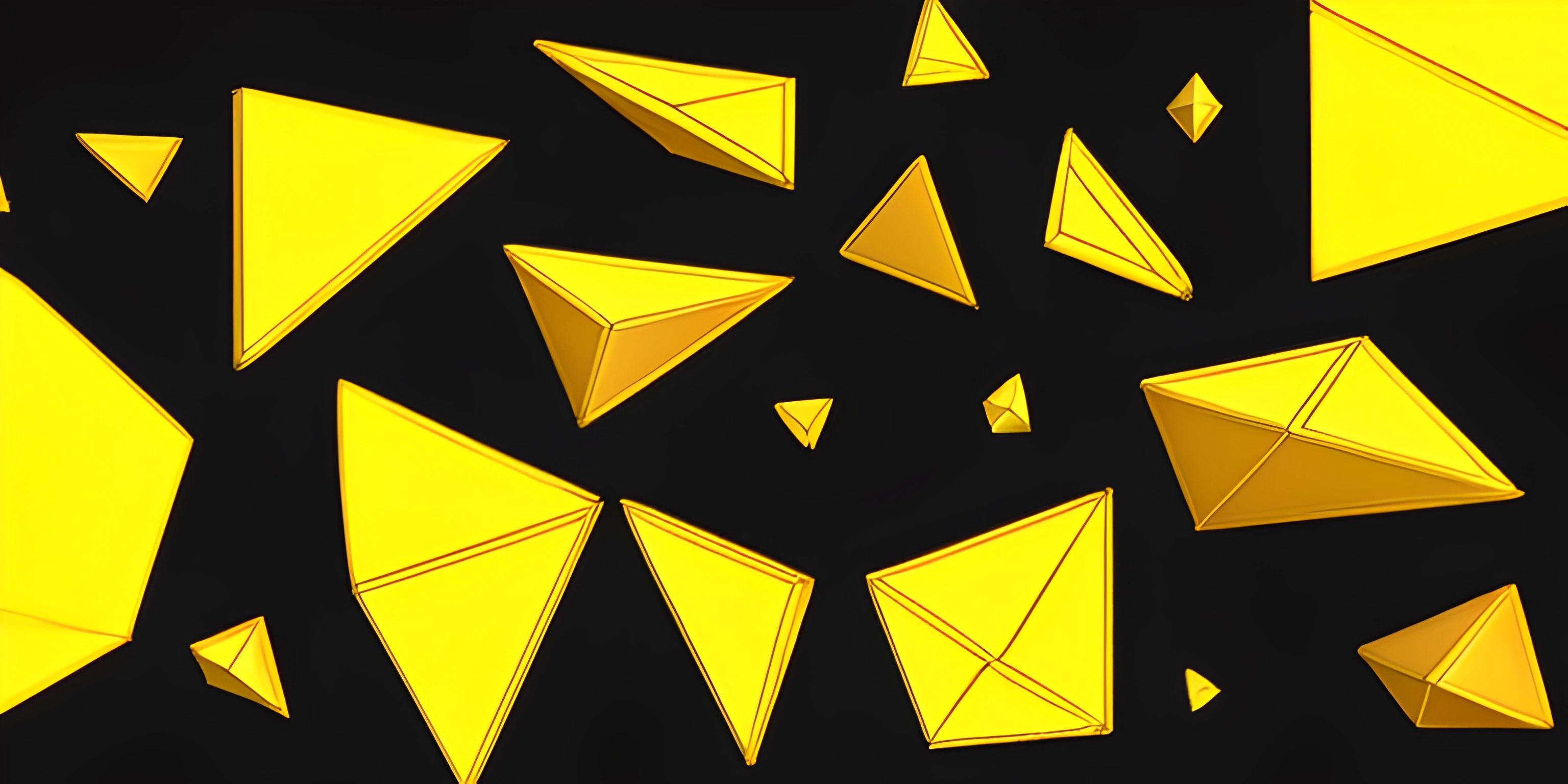
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Complex numbers are often used in mathematics, engineering, and physics. But, JavaScript doesn't have built-in support for complex numbers. Don't let that discourage you, though! In this article, we'll explore how to create a custom Complex
class to represent complex numbers and perform mathematical operations with them.
Complex Number Basics
A complex number is a number with a real part and an imaginary part. It's usually written in the form a + bi
, where a
is the real part, b
is the imaginary part, and i
is the imaginary unit, which is the square root of -1.
To get started, let's create a Complex
class to represent complex numbers in JavaScript.
class Complex { constructor(real, imaginary) { this.real = real; this.imaginary = imaginary; } }
Now, we've got a basic Complex
class that can hold a complex number's real and imaginary parts. But that's just the beginning! Let's add some methods to perform mathematical operations on our complex numbers.
Addition
To add two complex numbers, simply add their corresponding real parts and imaginary parts. Here's how to do it:
class Complex { // ... constructor and other code add(complex) { return new Complex(this.real + complex.real, this.imaginary + complex.imaginary); } }
Subtraction
Subtracting complex numbers is similar to addition. Just subtract the corresponding real and imaginary parts:
class Complex { // ... constructor and other code subtract(complex) { return new Complex(this.real - complex.real, this.imaginary - complex.imaginary); } }
Multiplication
Multiplying complex numbers involves some more arithmetic. To multiply two complex numbers (a + bi)
and (c + di)
, you can use the following formula:
(a + bi)(c + di) = (ac - bd) + (ad + bc)i
Here's how to implement multiplication in the Complex
class:
class Complex { // ... constructor and other code multiply(complex) { const real = this.real * complex.real - this.imaginary * complex.imaginary; const imaginary = this.real * complex.imaginary + this.imaginary * complex.real; return new Complex(real, imaginary); } }
Division
Division is a bit more complicated. To divide two complex numbers (a + bi)
and (c + di)
, you can use the following formula:
(a + bi) / (c + di) = [(ac + bd) / (c² + d²)] + [(bc - ad) / (c² + d²)]i
Here's the implementation for the Complex
class:
class Complex { // ... constructor and other code divide(complex) { const denominator = complex.real ** 2 + complex.imaginary ** 2; const real = (this.real * complex.real + this.imaginary * complex.imaginary) / denominator; const imaginary = (this.imaginary * complex.real - this.real * complex.imaginary) / denominator; return new Complex(real, imaginary); } }
Putting It All Together
Now, we've implemented a Complex
class that can handle basic arithmetic operations with complex numbers. Here's the complete class for reference:
class Complex { constructor(real, imaginary) { this.real = real; this.imaginary = imaginary; } add(complex) { return new Complex(this.real + complex.real, this.imaginary + complex.imaginary); } subtract(complex) { return new Complex(this.real - complex.real, this.imaginary - complex.imaginary); } multiply(complex) { const real = this.real * complex.real - this.imaginary * complex.imaginary; const imaginary = this.real * complex.imaginary + this.imaginary * complex.real; return new Complex(real, imaginary); } divide(complex) { const denominator = complex.real ** 2 + complex.imaginary ** 2; const real = (this.real * complex.real + this.imaginary * complex.imaginary) / denominator; const imaginary = (this.imaginary * complex.real - this.real * complex.imaginary) / denominator; return new Complex(real, imaginary); } }
With this custom Complex
class, you can now work with complex numbers in JavaScript with ease. Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Mandelbrot Set (psst, it's free!).
FAQ
What are complex numbers and why are they important in JavaScript?
Complex numbers are numbers that consist of two parts: a real part and an imaginary part. They are usually written in the form a + bi
, where a
is the real part, b
is the imaginary part, and i
is the imaginary unit. Complex numbers play a significant role in various fields such as engineering, physics, and mathematics. In JavaScript, they can be useful for solving problems that require working with complex numbers or for implementing algorithms that involve complex arithmetic.
How can I create a custom Complex class in JavaScript?
To create a custom Complex class in JavaScript, you can define a class with a constructor that takes two arguments: the real and imaginary parts of the complex number. Here's an example:
class Complex { constructor(real, imaginary) { this.real = real; this.imaginary = imaginary; } }
You can then create instances of this class by providing the real and imaginary parts as arguments:
let complexNumber = new Complex(3, 4);
How can I perform basic arithmetic operations with complex numbers in JavaScript?
You can add methods to the Complex class to perform basic arithmetic operations such as addition, subtraction, multiplication, and division. For example, you can implement the add()
method as follows:
class Complex { // ...constructor... add(other) { return new Complex(this.real + other.real, this.imaginary + other.imaginary); } }
Similarly, you can implement other arithmetic operations by following the rules of complex number arithmetic.
How can I calculate the magnitude and argument of a complex number in JavaScript?
To calculate the magnitude and argument of a complex number, you can add methods to the Complex class that utilize the Math library. For example, the magnitude can be calculated using the Pythagorean theorem, and the argument can be calculated using the Math.atan2()
function:
class Complex { // ...constructor and other methods... magnitude() { return Math.sqrt(this.real * this.real + this.imaginary * this.imaginary); } argument() { return Math.atan2(this.imaginary, this.real); } }
How can I perform complex conjugation in JavaScript?
Complex conjugation is the operation of changing the sign of the imaginary part of a complex number. You can add a method to the Complex class for complex conjugation as follows:
class Complex { // ...constructor and other methods... conjugate() { return new Complex(this.real, -this.imaginary); } }
With this method, you can easily create the complex conjugate of a complex number:
let complexNumber = new Complex(3, 4); let conjugateNumber = complexNumber.conjugate();