Complex Numbers and Their Operations
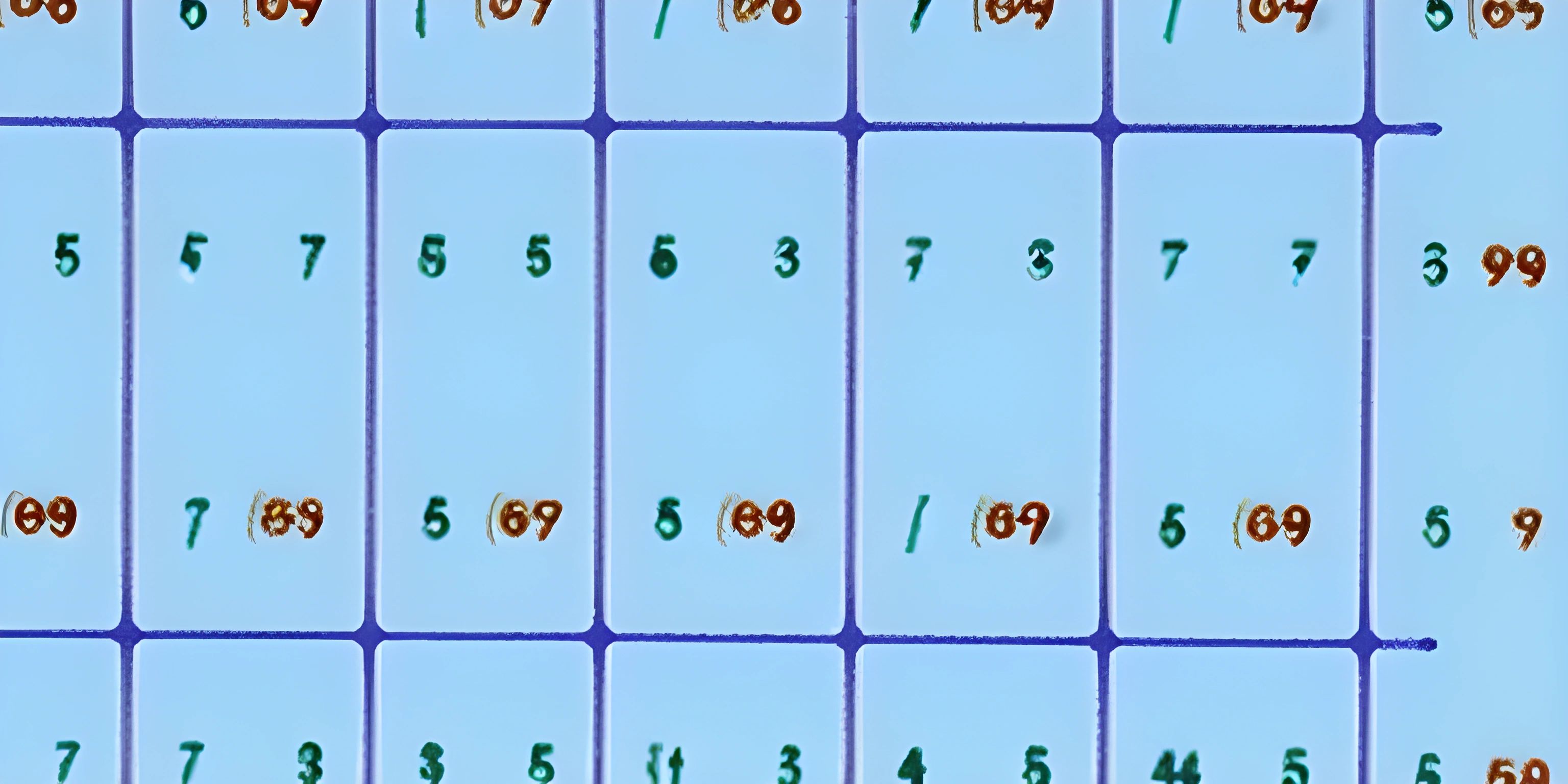
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Complex numbers may sound daunting, but they are, in fact, quite 'real' in the world of mathematics and programming. With a pinch of imagination and a dollop of Python, we'll venture into this intriguing realm!
What are Complex Numbers anyway?
A complex number is a number that can be expressed in the form a + bi
, where a
and b
are real numbers, and i
is a solution of the equation x² = -1
. Because no real number satisfies this equation, i
is called an imaginary unit, and b
is the imaginary part.
Creating Complex Numbers in Python
Python provides a built-in complex
data type to represent complex numbers. You can form a complex number using the complex(a, b)
function, which returns a complex number a + bi
.
# Creating complex numbers z1 = complex(2, 3) z2 = complex(4, -1) print("z1 =", z1) print("z2 =", z2)
This code will output: z1 = (2+3j)
and z2 = (4-1j)
. Notice the 'j' notation Python uses for the imaginary unit!
Operations on Complex Numbers
Now let's see how to perform basic mathematical operations on these mysterious entities. Fear not, your Python interpreter is a powerful wizard who can handle this.
Addition
Adding two complex numbers is as easy as pie. You just have to add the real parts and the imaginary parts separately.
# Adding complex numbers z3 = z1 + z2 print("z3 =", z3)
This code will output: z3 = (6+2j)
.
Multiplication
Multiplying complex numbers might seem like a nightmare straight out of an algebra book, but Python takes care of that, too.
# Multiplying complex numbers z4 = z1 * z2 print("z4 =", z4)
This code will output: z4 = (11+10j)
.
And there you have it, folks! A whirlwind tour of complex numbers and their operations. But wait, there's more! With libraries like NumPy, you can perform even more complex operations. That's a tale for another day, though!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Mandelbrot Set (psst, it's free!).
FAQ
What's a complex number?
A complex number is a number that can be expressed in the form a + bi
, where a
and b
are real numbers, and i
is a solution of the equation x² = -1
. Because no real number satisfies this equation, i
is called an imaginary unit. In Python, complex numbers are represented using the j
notation instead of i
.
How do you add complex numbers?
Adding complex numbers is simple. You just have to add the real parts and the imaginary parts separately. In Python, you can just use the +
operator to add two complex numbers.
How do you multiply complex numbers in Python?
Multiplying complex numbers in Python is as simple as using the *
operator. Python takes care of the multiplication of the real and imaginary parts.
Can Python handle mathematical operations on complex numbers?
Yes, Python has built-in support for complex numbers and their operations. The complex
data type in Python helps handle complex numbers and their arithmetic straight out of the box.