Consistent Hashing Explained
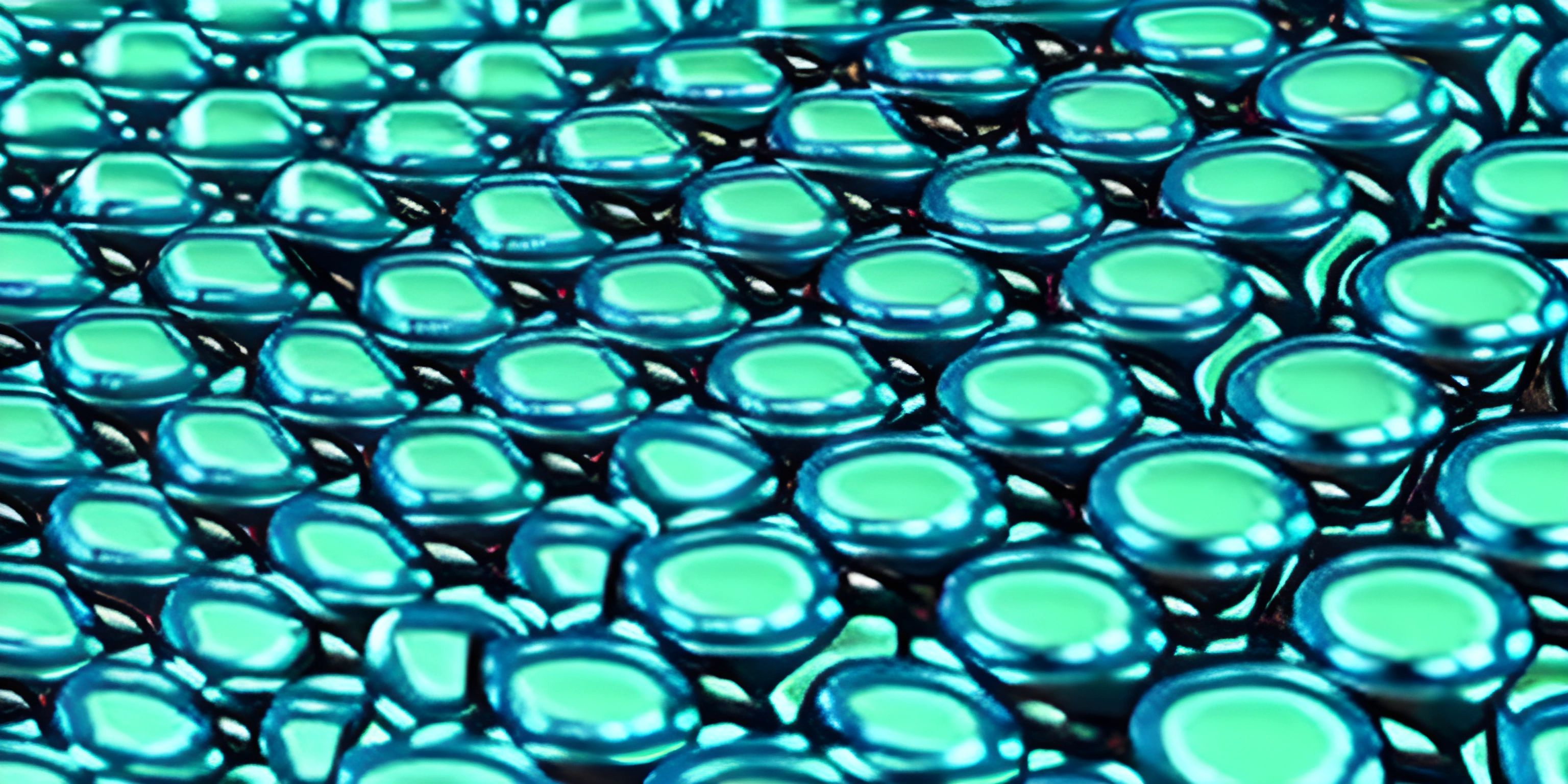
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Ever wondered how large-scale distributed systems like Amazon, Google, and Facebook manage to distribute data so evenly and handle re-distribution when a node goes down or a new one joins? Well, the secret lies in a concept called "consistent hashing". It's like that old game of musical chairs, but with a mathematical twist that ensures nobody crashes into each other when the music stops. Let's dive into this intriguing concept!
Consistent Hashing: The Game of Musical Chairs for Data
Imagine a game of musical chairs where the chairs are arranged in a circle and the players walk around until the music stops. The idea is to find a chair to sit in. This is essentially how consistent hashing works.
In consistent hashing, data items (think of them as the players in our game) are distributed across available nodes (the chairs) arranged in a circular space, often visualized as a hash ring.
Let's use a simple pseudocode example:
hash_ring = ConsistentHashRing(nodes) data_location = hash_ring.get_node("data_item")
In this code, ConsistentHashRing
is a representation of the hash ring, and get_node
maps the data_item
to a node on the ring. But what if a new node joins or an existing one leaves? How does our game of musical chairs adapt? That's where the consistent part comes in.
Consistent: Keep the Moves to a Minimum
In a traditional hash table, if the number of slots (nodes) changes, nearly all keys are remapped, turning our musical chairs into a chaotic scramble. Consistent hashing does something smarter.
When a node is added or removed, consistent hashing only remaps keys that were assigned to that node. This minimizes the number of keys that need to be relocated, crucial for large-scale systems that handle massive amounts of data.
hash_ring.add_node(new_node) data_location = hash_ring.get_node("data_item")
In the above code, when we add a new node to the hash ring, only a minimal number of data items will be reassigned to the new node. Other data items continue to sit comfortably on their original nodes, oblivious to the change. This "minimal disruption" feature is why consistent hashing is the MVP in distributed systems.
Applications: Distributed Systems, Load Balancing, and More
Consistent hashing is the secret sauce behind many distributed data storage systems. It's used in distributed caches like Memcached, DHTs like Apache Cassandra, and even web servers for session management and load balancing.
It's like having a super-efficient traffic management system that ensures a smooth flow of data, even during the digital equivalent of rush hour, or when a road (node) is temporarily blocked or a new one is opened.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is consistent hashing?
Consistent hashing is a hashing technique used in distributed systems to evenly distribute data across multiple nodes. Unlike traditional hashing, where a change in the number of slots causes a rehash of all items, consistent hashing ensures minimal remapping when a node is added or removed.
How does consistent hashing work?
Consistent hashing arranges nodes in a circular hash ring. Each data item is hashed to a position on the ring, and the item is assigned to the nearest node in the clockwise direction. When a node is added or removed, only items mapped to that node are remapped, causing minimal disruption.
Why is consistent hashing important in distributed systems?
Consistent hashing is crucial in distributed systems as it minimizes the amount of data that needs to be moved around when nodes join or leave the system. This is vital for performance and availability in large-scale systems that handle massive amounts of data.
Can you give an example of where consistent hashing is used?
Consistent hashing is used in many distributed storage systems. For example, it's used in distributed caches like Memcached, distributed hash tables (DHTs) like Apache Cassandra, and in web servers for session management and load balancing.
What's the downside of consistent hashing?
One common issue with consistent hashing is that it can lead to an uneven distribution of data if there is a "clustering" of keys around certain nodes. This is often addressed using "virtual nodes", where each physical node is associated with multiple points on the hash ring, smoothing out the distribution of keys.