Concurrency Management
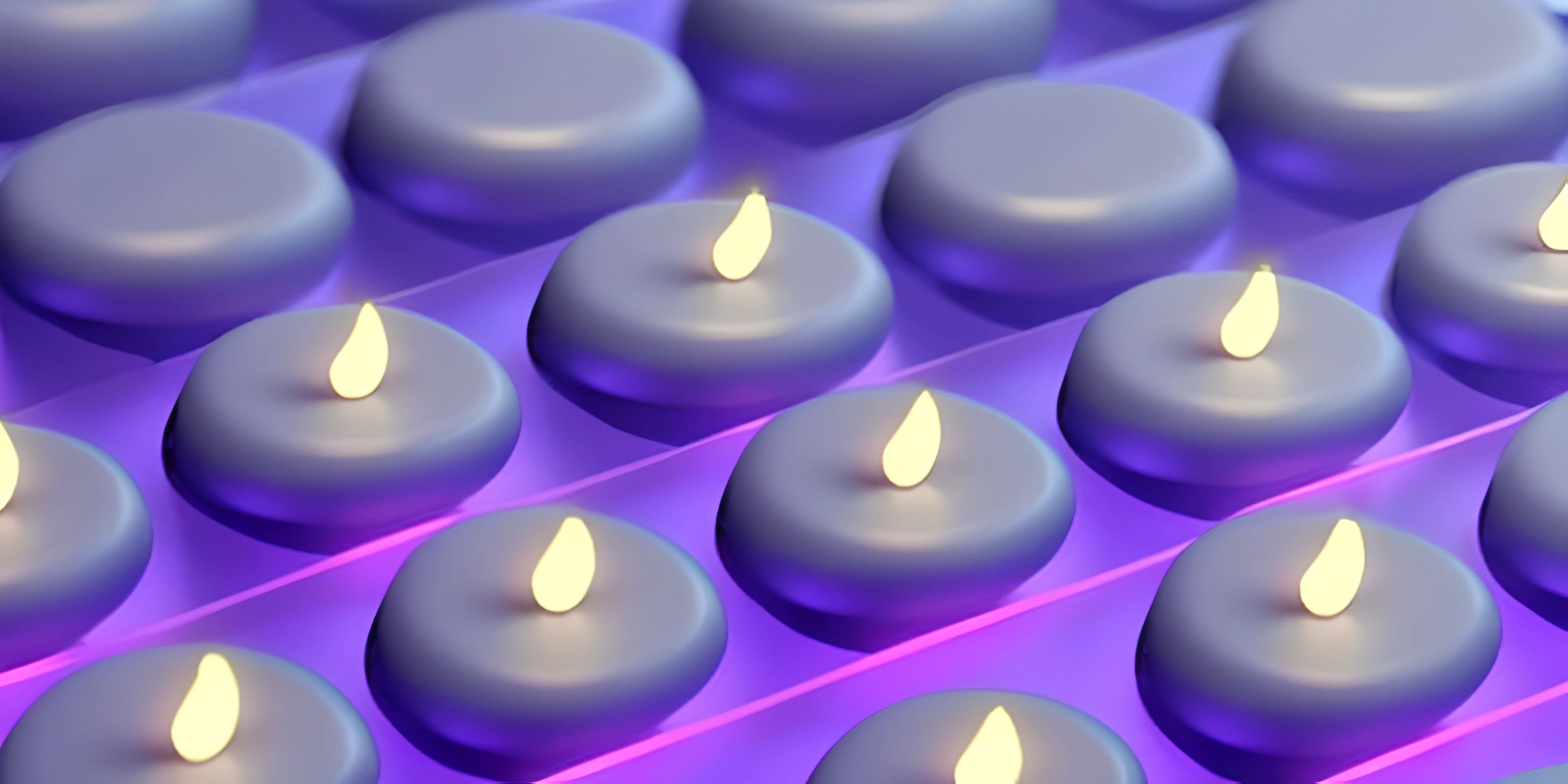
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
When diving into the world of programming, you'll eventually encounter a tricky and exciting concept: concurrency. Imagine a bustling kitchen with multiple chefs working together to prepare a feast. In the realm of software development, concurrency is akin to having several chefs (or threads) working simultaneously to accomplish a task, making everything faster and more efficient.
What is Concurrency?
Concurrency is the simultaneous execution of multiple tasks or threads within a program. It allows your program to perform multiple operations in parallel, utilizing the full potential of modern, multi-core processors.
When dealing with concurrency, it's essential to manage it properly. With great power comes great responsibility, and that's where concurrency management comes into play.
Why Concurrency Management Matters
Just like in our bustling kitchen scenario, if chefs don't coordinate their actions, chaos may ensue. They might end up using the same ingredients, bumping into each other, or even ruining a dish. The same goes for concurrent programming. When multiple threads access shared resources, you need to ensure that they don't interfere with each other, leading to unpredictable results or errors.
Concurrency management helps solve these problems by providing techniques and mechanisms to coordinate and synchronize threads, ensuring that they work harmoniously and avoid conflicts.
Synchronization Techniques
There are several synchronization techniques that can be used to manage concurrent threads, some of which include:
-
Locks: Locks act like an exclusive pass that allows only one thread to access a shared resource at a time. Think of a lock as a key to the kitchen pantry - only the chef with the key can access and use the ingredients inside, ensuring no mix-ups or accidents.
-
Semaphores: Semaphores are more advanced than locks, allowing multiple threads to access a shared resource up to a specified limit. In our kitchen analogy, this would be like having a limited number of cutting boards - multiple chefs can work simultaneously, but once all the cutting boards are in use, the remaining chefs must wait.
-
Atomic operations: Atomic operations are actions that are completed in a single, uninterruptible step. It's like having a chef who performs a task so quickly that no one else can interfere or interrupt - there's no chance of a collision or conflict.
-
Monitors: Monitors are high-level synchronization constructs that wrap around a shared resource, providing synchronized access to it. Picture a kitchen manager who oversees the chefs and ensures they work in harmony, coordinating their actions to avoid accidents.
These techniques play a significant role in concurrency management, helping your program maintain its integrity while reaping the benefits of concurrency.
Languages and Libraries
Many programming languages, such as Java, Python, and C++, provide built-in support for concurrency management. Additionally, there are specialized libraries and frameworks designed to make concurrency management more accessible and efficient, like asyncio for Python or Java's Concurrency API.
In conclusion, concurrency management is an essential aspect of software development that allows you to harness the full power of concurrent programming while avoiding potential pitfalls. By understanding and applying synchronization techniques, you can create efficient and robust applications that keep up with the demands of the modern world.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is concurrency management and why is it important in software development?
Concurrency management is a concept in software development that involves coordinating the simultaneous execution of multiple tasks. It ensures that resources, such as memory and processing power, are utilized efficiently, and that tasks are completed without causing conflicts, data inconsistencies, or deadlocks. Concurrency management is crucial in software development because it helps improve application performance, enables seamless multitasking, and provides a better user experience.
How can developers handle concurrency in their applications?
Developers can handle concurrency in their applications by employing various techniques, such as:
- Using multi-threading or multi-processing to allow multiple tasks to run concurrently
- Implementing synchronization mechanisms like locks, semaphores, or monitors to ensure that shared resources are accessed in a controlled manner
- Applying optimistic or pessimistic concurrency control strategies to manage concurrent access to shared data
- Leveraging parallel programming models and frameworks, such as OpenMP, MPI, or TPL, to simplify concurrent programming
What are some common challenges faced in concurrency management?
Some common challenges faced in concurrency management include:
- Deadlocks: A situation where two or more tasks wait indefinitely for resources held by each other
- Race conditions: Occur when the behavior of a program depends on the relative timing of events, which can lead to unexpected results or data inconsistencies
- Starvation: A situation where a task is unable to proceed because it is perpetually denied access to a shared resource
- Contention: Occurs when multiple tasks compete for access to a shared resource, causing performance bottlenecks
- Complex debugging: Concurrent programs can be more difficult to debug due to the non-deterministic nature of concurrent execution
What is the difference between optimistic and pessimistic concurrency control?
Optimistic and pessimistic concurrency control are two strategies for managing concurrent access to shared data:
- Optimistic concurrency control (OCC) assumes that conflicts between tasks are rare. It allows tasks to access shared data without acquiring locks, and checks for conflicts only when a task tries to commit its changes. If a conflict is detected, the task must retry its operation.
- Pessimistic concurrency control (PCC) assumes that conflicts between tasks are likely. It requires tasks to acquire locks on shared data before accessing it, preventing other tasks from modifying the data until the lock is released. This strategy helps prevent conflicts but can lead to increased contention and decreased performance in scenarios with high concurrency.
Can you provide an example of using locks for concurrency management in a programming language?
Sure! Here's an example in Python using the threading
module to illustrate the use of locks for concurrency management:
import threading # Define a shared counter and a lock counter = 0 counter_lock = threading.Lock() def increment_counter(): global counter with counter_lock: counter += 1 print(f"Counter: {counter}") # Create multiple threads that increment the shared counter threads = [] for _ in range(10): thread = threading.Thread(target=increment_counter) threads.append(thread) thread.start() # Wait for all threads to complete for thread in threads: thread.join() print(f"Final counter value: {counter}")
In this example, the counter_lock
is used to ensure that only one thread can access and modify the counter
at a time, preventing race conditions and ensuring the correct final value of the counter.