Exploring GNU Assembler (GAS) and Its Usage
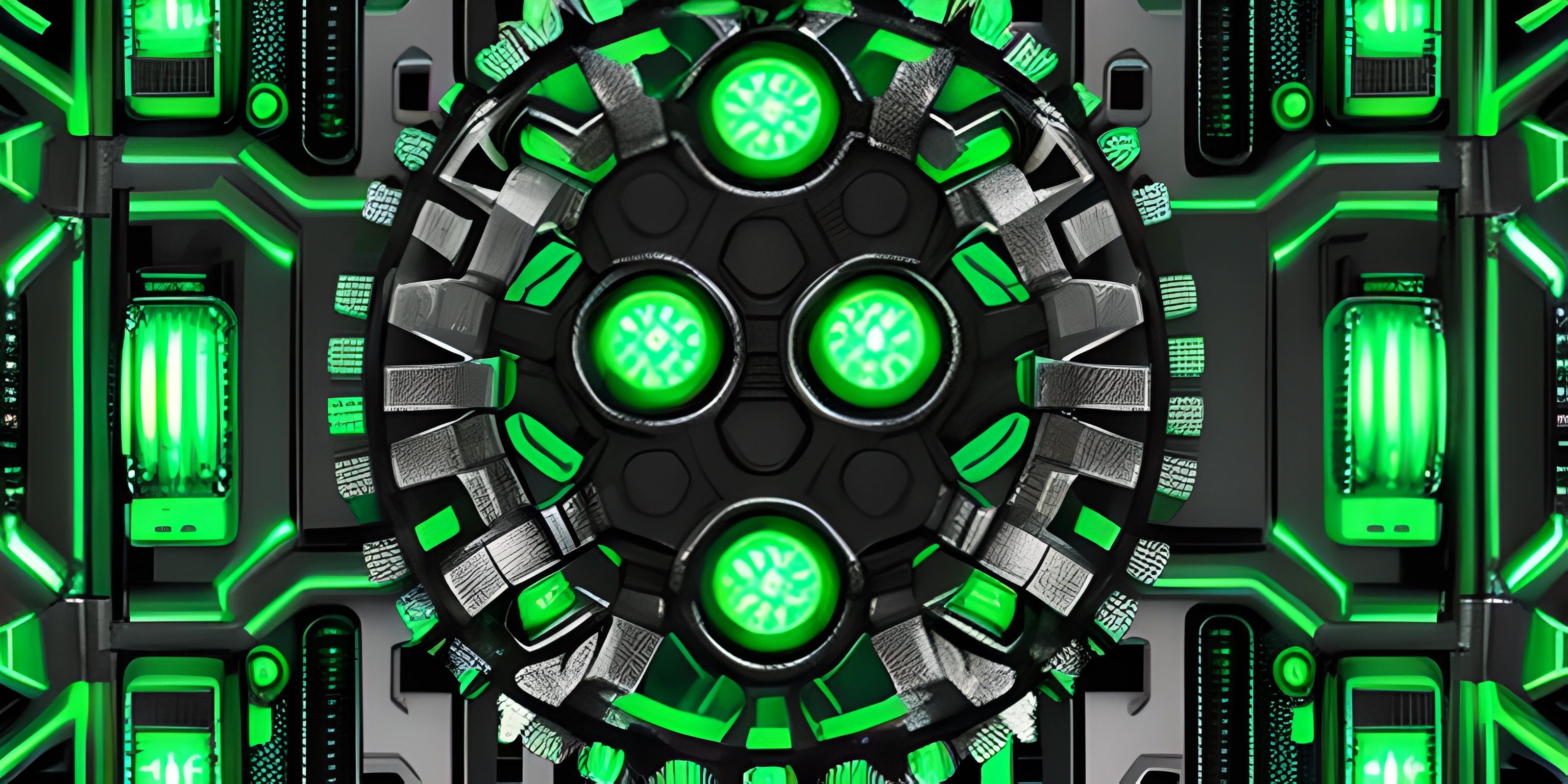
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
GNU Assembler, commonly known as GAS, is a powerful assembler that forms part of the GNU Compiler Collection (GCC). GAS is primarily used to convert low-level assembly language code into machine-readable binary code. This conversion is essential, as computers only understand binary instructions.
Writing Assembly Code for GAS
When writing assembly code for GAS, you'll use a syntax known as "AT&T syntax." This syntax is different from the "Intel syntax" used by other assemblers. The key differences lie in the way instructions, registers, and operands are written.
Here's a simple example of assembly code in AT&T syntax that utilizes GAS:
.section .data msg: .asciz "Hello, World!" .section .text .globl _start _start: # Write the message to stdout movl $4, %eax movl $1, %ebx leal msg, %ecx movl $13, %edx int $0x80 # Terminate the program movl $1, %eax xorl %ebx, %ebx int $0x80
This code prints "Hello, World!" to the standard output and then terminates the program.
Compiling and Linking with GAS
To compile and link your assembly code using GAS, follow these steps:
-
Save your assembly code in a file with a
.s
extension, such ashello.s
. -
Run the following command to assemble the code into an object file:
as -o hello.o hello.s
-
Link the object file using the following command:
ld -o hello hello.o
-
Execute the compiled program:
./hello
Your program should now print "Hello, World!" to the terminal.
Debugging Assembly Code
To debug your assembly code, you can use a debugger like gdb
. Here's a brief example of how to use gdb
with GAS:
-
Assemble and link the code as explained above.
-
Start the
gdb
debugger with your compiled program:gdb hello
-
Set a breakpoint at the
_start
label:break _start
-
Run the program within the debugger:
run
-
Use the
stepi
command to step through the assembly instructions one by one. -
Examine the register values using the
info registers
command. -
Continue stepping through the code until the program exits or you have debugged the issue.
Remember that debugging assembly code might be more challenging than debugging high-level languages, as you'll be working with raw instructions and registers.
Conclusion
GAS is a widely-used assembler that plays a crucial role in the GCC toolchain. By understanding the basics of GAS and how to use it to write, compile, and debug assembly code, you'll gain valuable insights into low-level programming and the inner workings of computer systems. Happy assembling!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Basic Concepts (psst, it's free!).
FAQ
What is the GNU Assembler (GAS)?
The GNU Assembler, also known as GAS, is a part of the GNU Toolchain and is responsible for converting assembly language code into machine code. It is a powerful and flexible assembler that supports a wide range of hardware architectures and is widely used in the development of operating systems, firmware, and low-level software.
How do I write assembly code for GAS?
Writing assembly code for GAS requires knowledge of the target hardware's instruction set and register usage. The basic format of GAS assembly code includes labels, directives, and instructions. Here's a simple example of a program that adds two numbers using x86-64 assembly:
.globl _start _start: mov $2, %rax # Load the value 2 into the RAX register add $3, %rax # Add the value 3 to the RAX register # The result, 5, is now stored in the RAX register
How do I compile and run my GAS assembly code?
To compile and run the GAS assembly code, follow these steps:
- Save your assembly code to a file, e.g.,
example.s
. - Assemble the code using the
as
command:as -o example.o example.s
- Link the object file using the
ld
command:ld -o example example.o
- Run the compiled program:
./example
Can I use GAS with other programming languages?
Yes, you can use GAS in combination with other programming languages, such as C or C++. This is useful when you need to optimize a specific portion of your code or when you need to access specific hardware features. To use GAS with other programming languages, you can use inline assembly or write separate assembly files and link them during the build process.
Which hardware architectures are supported by GAS?
GAS supports a wide range of hardware architectures, including x86, x86-64, ARM, MIPS, PowerPC, RISC-V, and many more. This versatility makes it a popular choice for cross-platform development and allows developers to create efficient, low-level code for various devices and systems.