Understanding and Implementing Breadth-First Search Algorithm
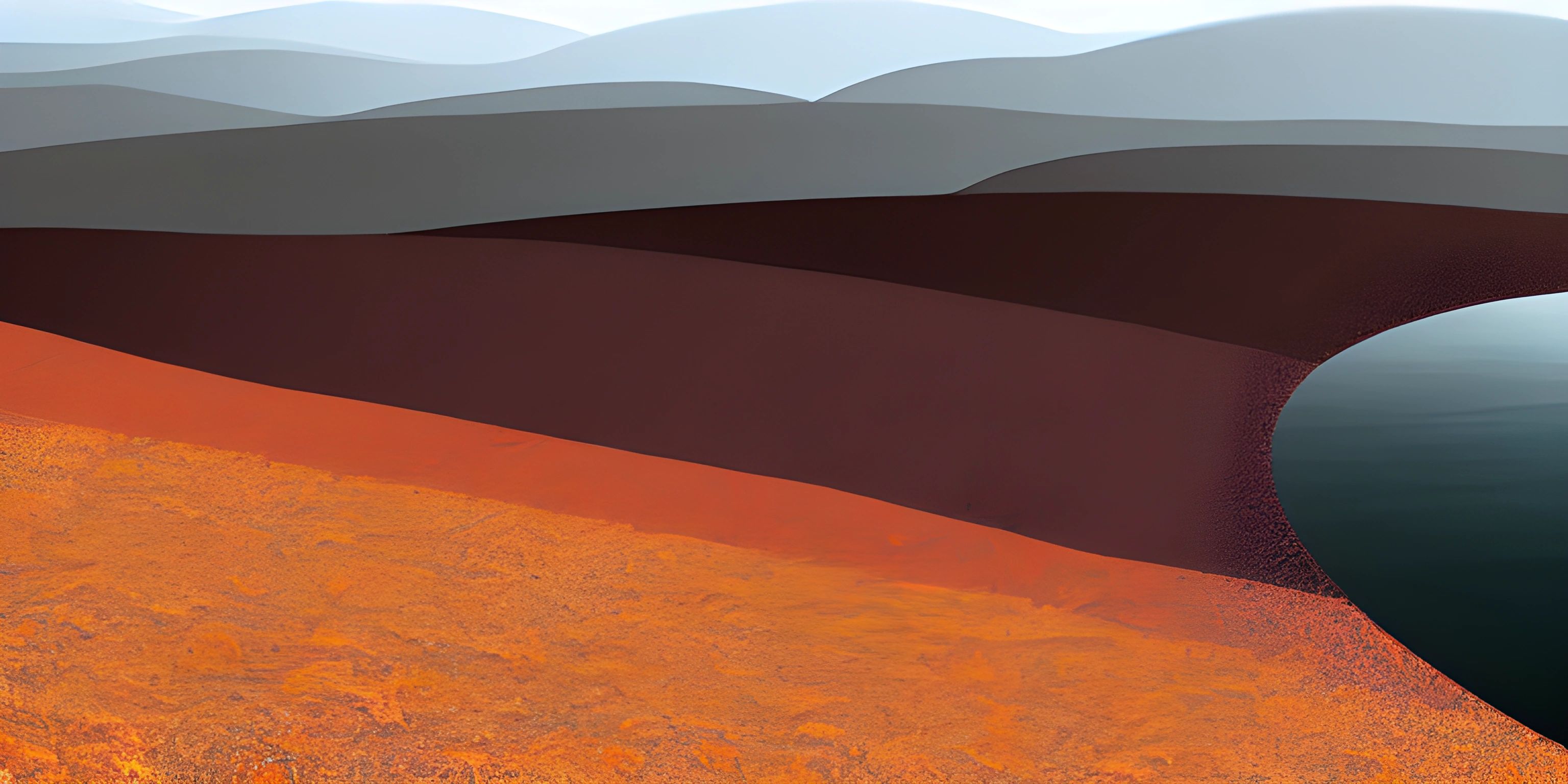
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Imagine you're in a maze. Your goal is to explore every possible path to find the exit. Much like you would check each path level by level, the Breadth-First Search (BFS) algorithm explores all nodes at the present depth level before moving on to nodes at the next level. Let's dive into this fascinating algorithm and learn how to implement it in Python.
Core Concepts of BFS
BFS is a graph traversal algorithm used to explore nodes and edges of a graph. It starts at a given node (often called the "source" or "root" node) and explores all its neighboring nodes at the present depth before moving on to nodes at the next depth level. This systematic approach ensures that the algorithm finds the shortest path from the source node to any other node in an unweighted graph.
Applications of BFS
- Shortest Path in an Unweighted Graph: BFS can find the shortest path between two nodes in an unweighted graph.
- Social Networking Sites: Finding the shortest connection path between two people.
- Web Crawlers: BFS is used to build a web crawler that systematically visits web pages.
- Finding Connected Components: In a graph, BFS can help find all connected components.
How BFS Works
To get a clearer picture, let's consider a simple graph:
1 / \ 2 3 / \ \ 4 5 6
Starting from node 1
, BFS will explore the nodes in the following order:
- Visit node
1
. - Visit all neighbors of node
1
(nodes2
and3
). - Visit all neighbors of nodes
2
and3
(nodes4
,5
, and6
).
BFS uses a queue to keep track of the nodes to be explored next, ensuring that nodes are explored level by level.
Implementing BFS in Python
Let's break down the implementation of BFS in Python. We'll use an adjacency list to represent the graph and a queue to manage the nodes to be explored.
from collections import deque def bfs(graph, start): # Initialize the queue with the start node queue = deque([start]) # Set to keep track of visited nodes visited = set() while queue: # Dequeue a node from the front of the queue node = queue.popleft() if node not in visited: print(f"Visited node: {node}") # Mark the node as visited visited.add(node) # Add all unvisited neighbors to the queue for neighbor in graph[node]: if neighbor not in visited: queue.append(neighbor) # Example graph represented as an adjacency list graph = { 1: [2, 3], 2: [4, 5], 3: [6], 4: [], 5: [], 6: [] } # Perform BFS starting from node 1 bfs(graph, 1)
Explanation of the Code
-
Initialization: We begin by importing
deque
from thecollections
module, which will serve as our queue. We also define thebfs
function that takes thegraph
andstart
node as parameters. -
Queue and Visited Set: Initialize the
queue
with thestart
node and create an emptyvisited
set to keep track of visited nodes. -
Queue Processing: While the queue is not empty:
- Dequeue a node from the front of the queue.
- If the node hasn't been visited, mark it as visited and print it.
- Add all unvisited neighbors of the current node to the queue.
-
Graph Representation: The graph is represented as an adjacency list, where each key is a node, and its value is a list of its neighbors.
Running the Code
When we run the provided code, it will visit the nodes in the following order: 1, 2, 3, 4, 5, 6. This order reflects the level-by-level exploration of BFS.
Optimizations and Variations
- Tracking Levels: If needed, you can track the level of each node by modifying the BFS implementation to keep count of levels.
- Path Reconstruction: To reconstruct the path from the start node to any target node, you can maintain a parent dictionary to backtrack from the target node to the start node.
FAQs
Q: What is the main difference between BFS and Depth-First Search (DFS)? A: The main difference lies in their approach to exploring nodes. BFS explores nodes level by level, using a queue, while DFS dives as deep as possible along a branch before backtracking, using a stack or recursion.
Q: How does BFS handle cycles in a graph? A: BFS handles cycles by keeping track of visited nodes. When a node is visited, it is added to a visited set, ensuring that the algorithm does not revisit the same node and hence avoids infinite loops.
Q: Can BFS be used on weighted graphs? A: BFS is typically used on unweighted graphs for finding the shortest path. For weighted graphs, algorithms like Dijkstra's Algorithm are more appropriate as they take edge weights into account.
Q: Is BFS suitable for finding the shortest path in all types of graphs? A: BFS is suitable for finding the shortest path in unweighted graphs. For weighted graphs, other algorithms like Dijkstra's or A* are more suitable.
Q: What is the time complexity of BFS? A: The time complexity of BFS is O(V + E), where V is the number of vertices and E is the number of edges in the graph. This is because every vertex and edge is explored at most once.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Syntax (psst, it's free!).