Inline Functions
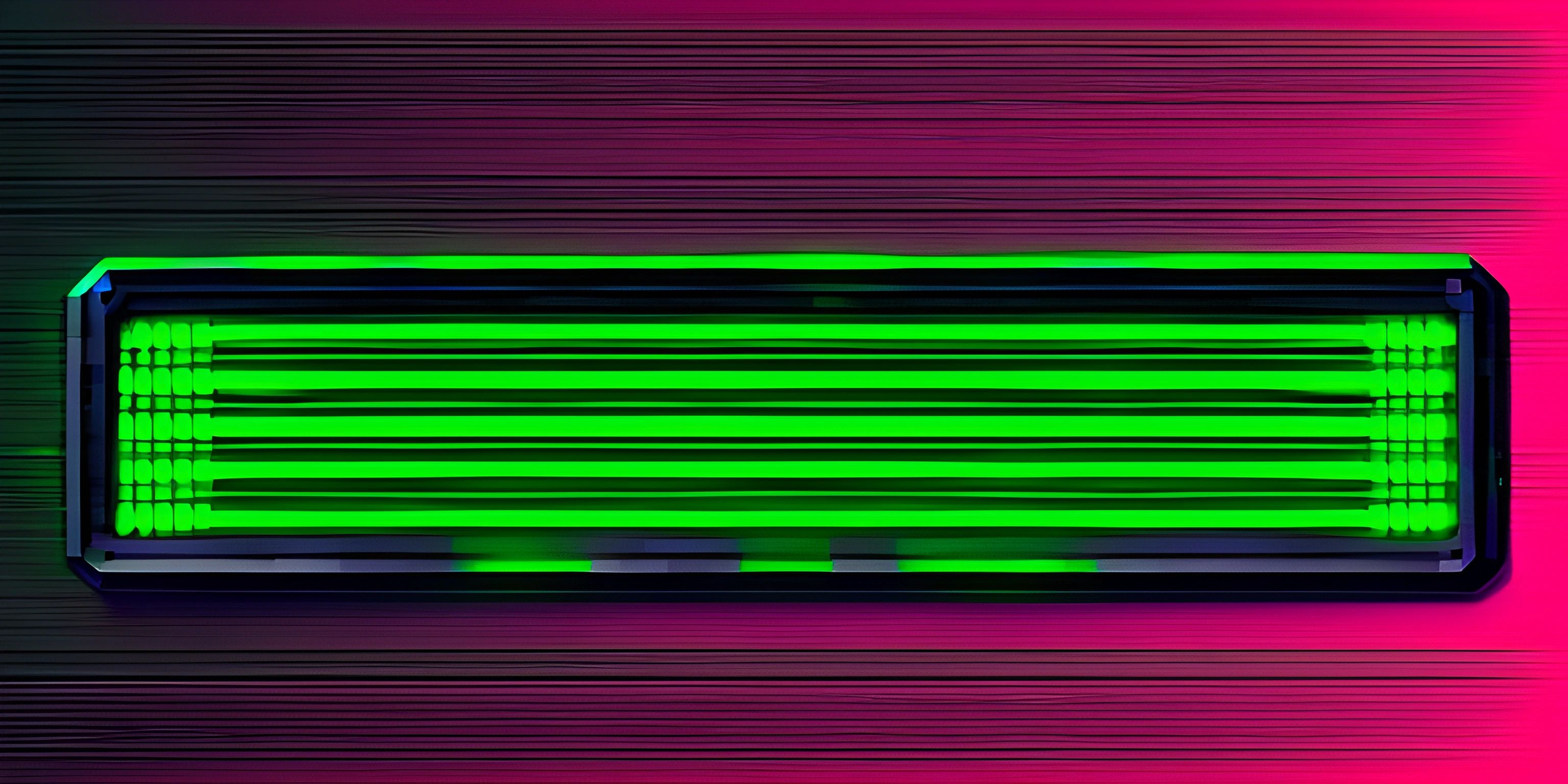
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Programming languages often provide us with ways to improve the performance of our code. One such technique is the use of inline functions. Inline functions are a compiler optimization that can help speed up your program and reduce function call overhead. It's like having the cake and eating it too, all without leaving the kitchen!
What are Inline Functions?
Inline functions are a way to tell the compiler to insert the body of a function directly at the call site instead of performing a regular function call. This can result in faster code execution, as it eliminates the overhead of calling a function, such as setting up the call stack and passing arguments. Imagine it as a way of "pasting" the code of the function wherever it's called, like using a copy-paste shortcut on your keyboard.
Here's a simple example in C++:
inline int add(int a, int b) { return a + b; } int main() { int result = add(3, 4); return 0; }
When the compiler processes the add
function, it sees the inline
keyword and replaces the function call in main()
with the function's body:
int main() { int result = (3 + 4); return 0; }
When to Use Inline Functions
Using inline functions is not always the best choice, as it can lead to increased code size, making your program take up more memory. However, they can be beneficial in certain situations:
-
Small functions: Inline functions work best for small, frequently called functions. These functions often have simple logic that can be executed quickly, making the function call overhead more significant in comparison. Inlining these functions can lead to noticeable performance improvements.
-
Performance-critical code: When you have a section of code that is performance-critical, inlining functions can help reduce the time it takes to execute that code. This is especially useful in real-time applications, such as games or multimedia processing, where every millisecond counts.
-
Template functions: In C++, template functions are good candidates for inlining because they generate specialized code at compile time. Inlining can help avoid code bloat by combining similar instances of template code into one.
When Not to Use Inline Functions
There are situations where inlining functions can do more harm than good:
-
Large functions: Inlining large functions can lead to increased code size, as the function's body is duplicated at every call site. This can result in a slower program, as it may take longer to load into memory and cache.
-
Recursive functions: Inlining recursive functions is generally not recommended, as it can lead to infinite code expansion and significantly increase the program's size. Recursion is typically better suited for regular function calls.
-
Dynamic libraries: Inlining functions in dynamic libraries can create compatibility issues, as the library may need to be recompiled to reflect the changes made by inlining.
It's essential to strike a balance between performance and code size when using inline functions. Choose wisely by analyzing the trade-offs, and let the compiler do the heavy lifting. After all, that's the power of inline functions!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is an inline function?
An inline function is a type of function in programming languages where the compiler replaces the function call with the actual body of the function. This can improve performance by reducing the overhead of calling a function, but it may also increase the code size. Inline functions are typically used for small and frequently called functions.
What are the advantages of using inline functions?
Inline functions offer several benefits, including:
- Improved performance by eliminating the function call overhead, which reduces the time spent on function calls and allows the program to run faster.
- Better code optimization by the compiler, as inlining makes it easier for the compiler to optimize the code.
- Enhanced code readability, as inline functions can make your code more compact and easier to read and understand.
When should I use inline functions in my code?
It's recommended to use inline functions when:
- The function is small and simple, as inlining larger functions may result in increased code size.
- The function is called frequently, as inlining can help reduce the overhead of function calls.
- The performance benefits of inlining outweigh the increased code size, as larger executables can lead to longer load times and increased memory usage.
Can I force a function to be always inlined?
Yes, you can force a function to be always inlined using the inline
keyword in C++ and some other languages. However, the final decision of inlining is still up to the compiler, and it may choose not to inline a function if it determines that doing so would not be beneficial. Here's an example in C++:
inline int add(int a, int b) { return a + b; }
Are there any downsides to using inline functions?
While inline functions can provide performance benefits, they can also have some downsides:
- Increased code size, as the function's code is duplicated at each call site. This can lead to longer load times and increased memory usage.
- Potentially slower compilation times, as the compiler needs to process and optimize the inlined code.
- Reduced code modularity, as changes to the inlined function may require recompiling all the code that uses it, rather than just recompiling the function itself.