Java Introduction
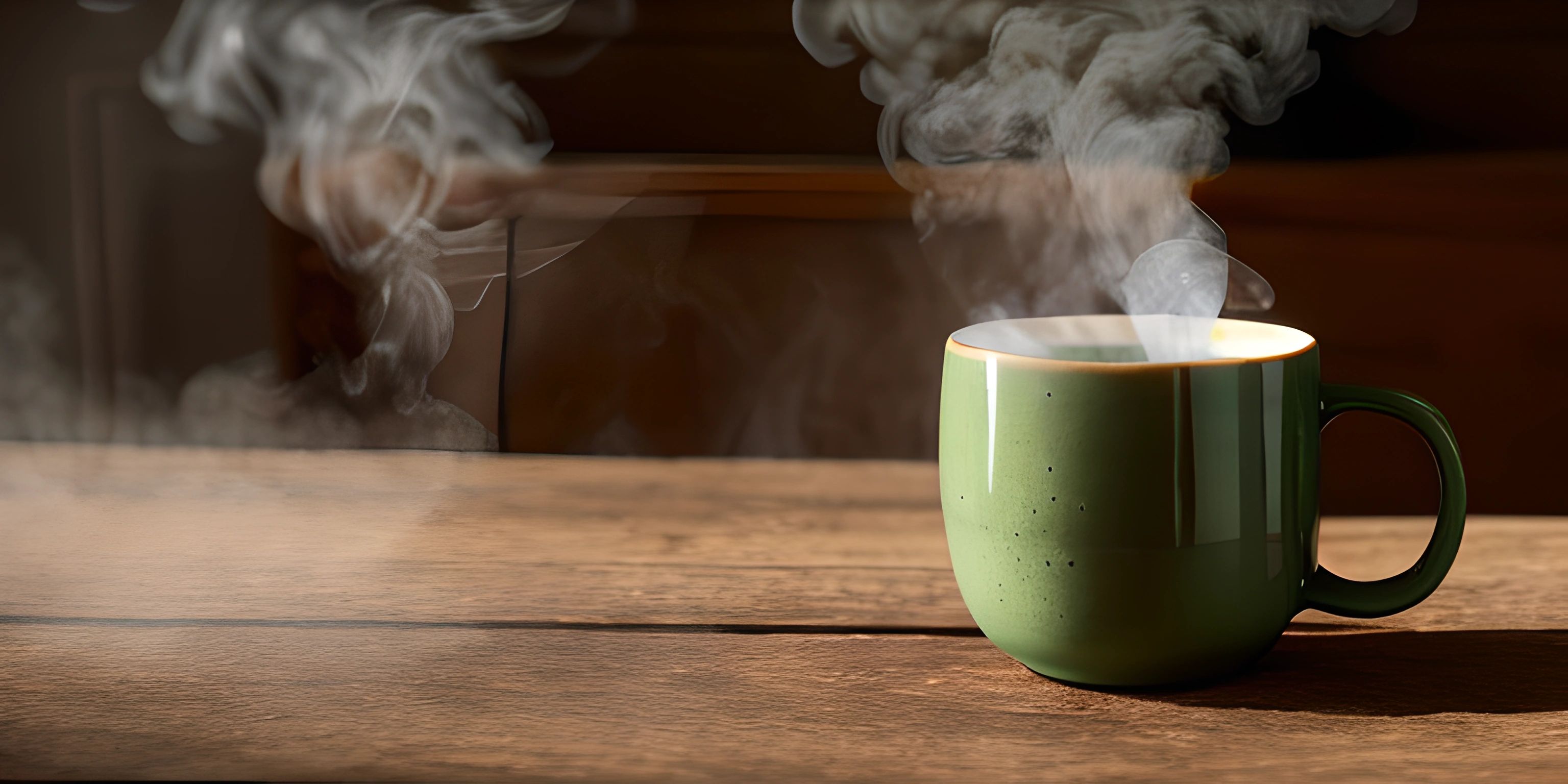
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Dive into the world of Java, one of the most popular and versatile programming languages out there. Java is the Swiss Army knife of programming, used for everything from web applications to mobile apps and even powering some of our favorite appliances. But enough with the introductions, let's unravel the Java magic!
Java Basics
Java is an object-oriented programming (OOP) language, which means it revolves around the concept of objects. Objects are instances of classes, and classes act as blueprints for creating these objects. We can think of a class as a recipe, and objects as the delicious cookies we bake using that recipe.
Syntax
To get started with Java, you'll need to understand its syntax. Java code is written within classes, and each class typically resides in its own file. Here's a simple "Hello, World!" example:
public class HelloWorld { public static void main(String[] args) { System.out.println("Hello, World!"); } }
Let's break it down:
public class HelloWorld
declares a new public class calledHelloWorld
.public static void main(String[] args)
is the main method, where the execution of the program begins.System.out.println("Hello, World!");
prints "Hello, World!" to the console.
Variables and Data Types
Java has several data types that store different kinds of values. There are two main categories: primitives (e.g., int
, float
, char
, boolean
) and reference types (e.g., objects and arrays). Variables are used to store these values:
int age = 25; float salary = 50000.0f; char initial = 'A'; boolean isActive = true;
Control Structures
Java has control structures that help you control the flow of your program:
- Conditionals (e.g.,
if
,else
,switch
) allow you to execute different blocks of code based on certain conditions. - Loops (e.g.,
for
,while
,do-while
) help you repeat a block of code multiple times.
Functions
Functions (also known as methods in Java) are blocks of code that perform specific tasks. Functions can receive input (parameters) and return output (return values). They help make your code reusable, modular, and efficient.
Here's an example of a simple function that adds two numbers:
public int add(int a, int b) { return a + b; }
OOP Concepts in Java
In Java, everything is built around the concept of objects. Some key OOP concepts include:
- Encapsulation: Combining data (variables) and methods within a class, while hiding the internal workings of the class from the outside world.
- Inheritance: Creating new classes from existing ones, reusing their properties and methods.
- Polymorphism: Allowing objects of different classes to be treated as objects of a common superclass.
- Abstraction: Simplifying complex systems by focusing on essential features and hiding unnecessary details.
Java Libraries
Java comes packed with a vast standard library known as the Java API (Application Programming Interface). The Java API provides pre-built functionality that makes it easier and faster to build applications.
Conclusion
Java is a powerful, versatile, and widely-used programming language. By understanding its essential concepts, you'll be well on your way to creating your own amazing applications. Now, grab a cup of coffee (pun intended) and start exploring the world of Java!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is Java and why is it popular?
Java is a versatile, object-oriented programming language designed for flexibility and ease of use. It is one of the most popular languages because it allows developers to create applications that can run on multiple platforms without the need for recompilation. Its widespread adoption is attributed to its reliability, performance, and vast ecosystem of libraries and frameworks.
Can you provide a simple example of a Java program?
Absolutely! Here's a basic example of a Java program that prints "Hello, World!" to the console:
public class HelloWorld { public static void main(String[] args) { System.out.println("Hello, World!"); } }
What are some essential concepts I should know in Java?
As a beginner, you should familiarize yourself with these essential Java concepts:
- Variables and data types
- Control structures (e.g., if statements, loops)
- Object-oriented programming principles (classes, objects, inheritance, polymorphism)
- Exception handling
- Collections and generics
- File I/O and serialization
How do I compile and run a Java program?
To compile a Java program, use the javac
command followed by the file name. For example, to compile a file named MyProgram.java
, you'd type javac MyProgram.java
. This will generate a bytecode file with the extension .class
.
To run the compiled program, use the java
command followed by the class name (without the .class extension). In our example, you'd type java MyProgram
to execute the program.
What are some popular Java development tools and frameworks?
There are numerous tools and frameworks available for Java development. Some popular choices include:
- Integrated Development Environments (IDEs): IntelliJ IDEA, Eclipse, and NetBeans
- Build tools: Maven and Gradle
- Testing frameworks: JUnit and TestNG
- Web development frameworks: Spring, JavaServer Faces (JSF), and Apache Struts
- Android development tools: Android Studio and the Android Software Development Kit (SDK)