Drawing Shapes with Java's Graphics2D Library
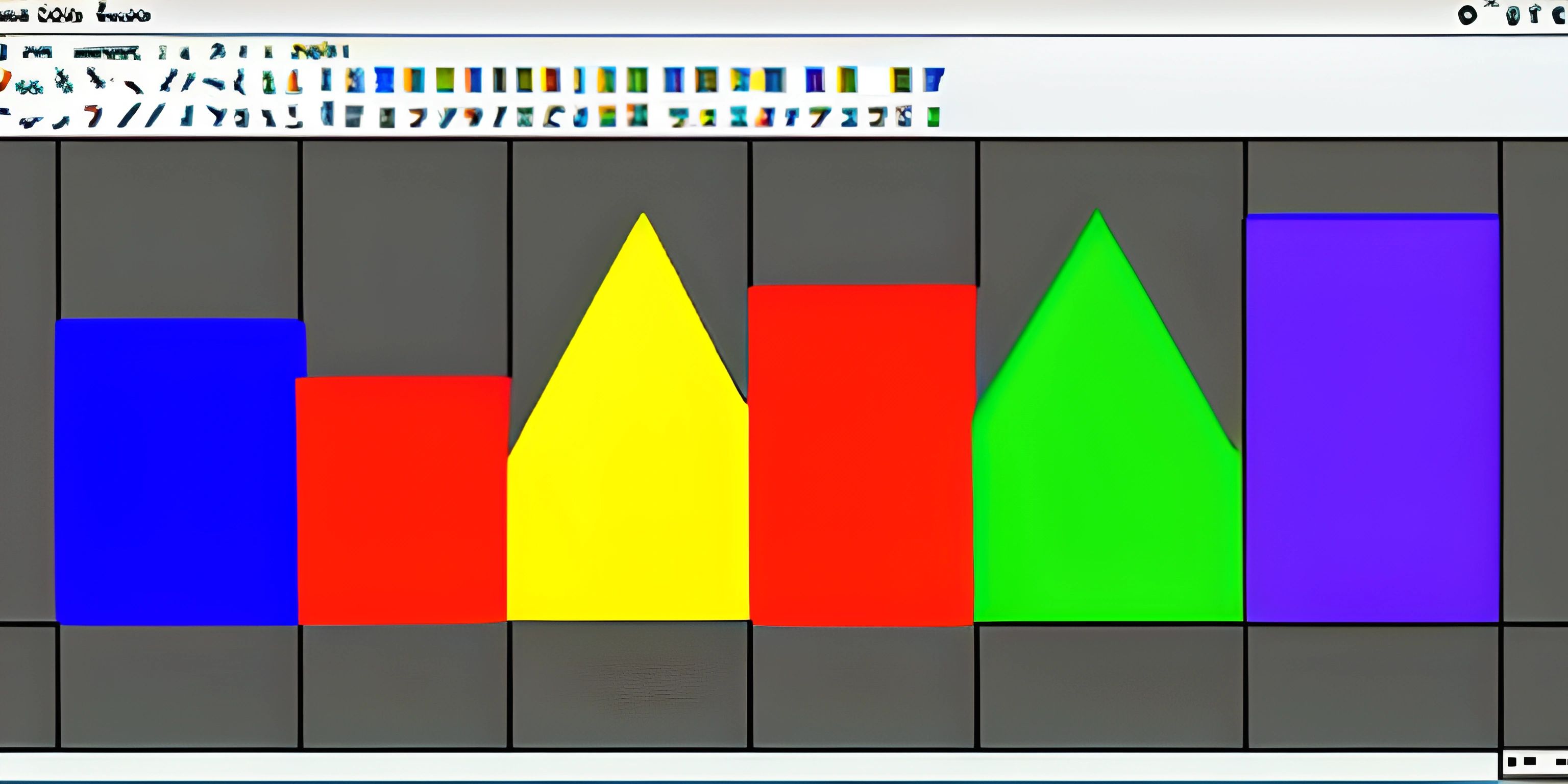
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Step into the vibrant world of digital art with Java's Graphics2D library! Graphics2D is a powerful library for creating two-dimensional graphics and animations in Java applications. With a wide array of tools at your disposal, you'll be well on your way to creating captivating visuals and mesmerizing animations.
Getting Started with Graphics2D
To begin, you'll need to set up a Java project and import the essential classes. Start by importing java.awt.Graphics
and java.awt.Graphics2D
, which provide the core functionality for drawing with Graphics2D. Additionally, import javax.swing.JFrame
and javax.swing.JPanel
to create a window and canvas for your artwork.
import java.awt.Graphics; import java.awt.Graphics2D; import javax.swing.JFrame; import javax.swing.JPanel;
Now, create a class that extends JPanel
and overrides the paintComponent
method. The paintComponent
method is where all the magic happens—it's the canvas where you'll be painting your shapes.
public class MyArtwork extends JPanel { @Override protected void paintComponent(Graphics g) { super.paintComponent(g); Graphics2D g2d = (Graphics2D) g; // Drawing code goes here } }
To display your artwork, create a JFrame
and add an instance of your custom JPanel
class to it.
public static void main(String[] args) { JFrame frame = new JFrame("My Artwork"); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setSize(800, 600); frame.add(new MyArtwork()); frame.setVisible(true); }
Now that you have your canvas set up, let's dive into the exciting world of shapes and colors!
Drawing Basic Shapes
The Graphics2D library provides various methods for drawing simple shapes such as lines, rectangles, circles, and more. Let's explore some of them!
Drawing a Line
To draw a line, use the drawLine
method, which takes four parameters: the starting x and y coordinates, and the ending x and y coordinates.
g2d.drawLine(50, 50, 150, 150);
Drawing a Rectangle
To draw a rectangle, use the drawRect
method. This method takes four parameters: the x and y coordinates of the top-left corner, and the width and height of the rectangle.
g2d.drawRect(100, 100, 200, 100);
Drawing an Oval
To draw an oval, use the drawOval
method. Like drawRect
, this method takes four parameters: the x and y coordinates of the bounding rectangle's top-left corner, and the width and height of the bounding rectangle.
g2d.drawOval(300, 300, 100, 50);
Filling Shapes with Color
To fill shapes with color, first set the color using the setColor
method, and then use the corresponding "fill" method for the desired shape.
g2d.setColor(Color.BLUE); g2d.fillRect(100, 100, 200, 100);
Transformations
Graphics2D also supports various transformations such as translation, rotation, and scaling. To apply a transformation, use the translate
, rotate
, or scale
methods before drawing your shapes.
g2d.translate(300, 300); g2d.rotate(Math.toRadians(45)); g2d.scale(2, 1); g2d.drawRect(-50, -50, 100, 100);
Remember to reset the transformations after drawing your shapes to avoid unwanted effects on subsequent drawings.
g2d.setTransform(new AffineTransform());
With these tools in your arsenal, you're ready to unleash your creativity and explore the endless possibilities of Java's Graphics2D library. Happy drawing!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Drawing Basic Shapes (psst, it's free!).