Understanding Java Serialization and Its Usage
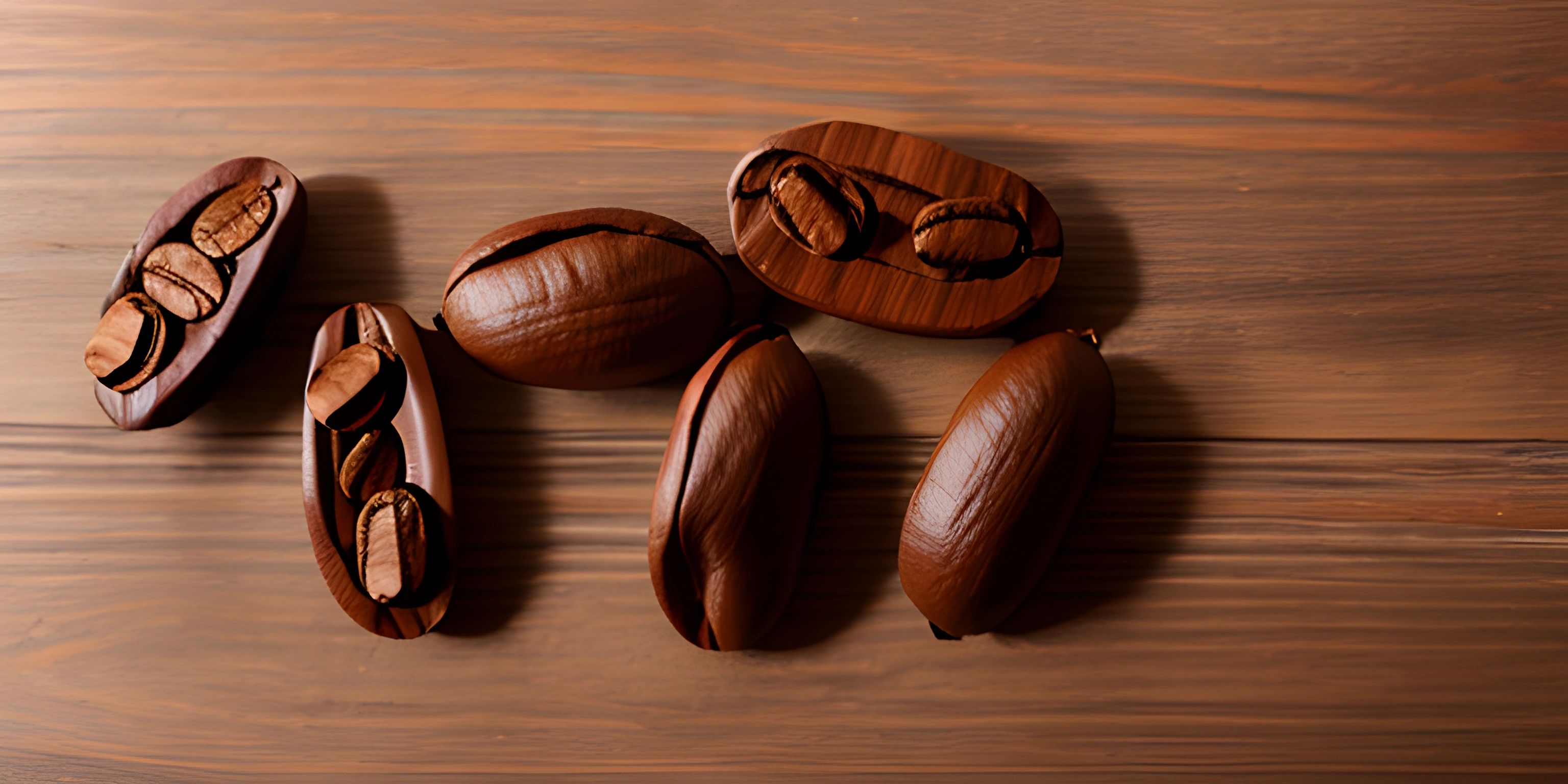
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Java Serialization is a powerful feature that allows you to convert an object's state into a byte stream, which can then be transported or stored. This process of turning an object into a sequence of bytes is known as serialization. On the flip side, you can also retrieve the object's state from the byte stream and reconstruct the object, a process known as deserialization.
Why Serialize?
Java Serialization is particularly useful when you need to:
-
Persist data: Serialization is a fantastic way to save an object's state to a file or a database for later retrieval.
-
Send objects across a network: You can serialize objects to send them over a network to another application, where they can be deserialized, and their state can be reconstructed.
-
Deep copy objects: Serialization can help you create a deep copy of an object, which is an entirely new object with the same state as the original, but independent of it.
How to Serialize and Deserialize Objects in Java
In order to serialize an object in Java, the class of the object must implement the java.io.Serializable
interface. This interface is a marker interface, meaning it doesn't have any methods to implement. Its sole purpose is to indicate that the class can be serialized.
Serialization Example
Let's take a look at an example. Here's a class representing a person:
import java.io.Serializable; public class Person implements Serializable { private String name; private int age; public Person(String name, int age) { this.name = name; this.age = age; } // Getters and setters }
In this example, the Person
class implements the Serializable
interface. Now, we can serialize and deserialize objects of this class.
To serialize an object, you can use ObjectOutputStream
from the java.io
package:
import java.io.FileOutputStream; import java.io.IOException; import java.io.ObjectOutputStream; public class SerializeExample { public static void main(String[] args) { Person person = new Person("John Doe", 30); try (FileOutputStream fileOut = new FileOutputStream("person.ser"); ObjectOutputStream objectOut = new ObjectOutputStream(fileOut)) { objectOut.writeObject(person); System.out.println("Person object serialized successfully."); } catch (IOException e) { e.printStackTrace(); } } }
The above code creates a Person
object and then uses ObjectOutputStream
to write the object to a file named person.ser
.
Deserialization Example
To deserialize an object, you can use ObjectInputStream
from the java.io
package:
import java.io.FileInputStream; import java.io.IOException; import java.io.ObjectInputStream; public class DeserializeExample { public static void main(String[] args) { Person deserializedPerson; try (FileInputStream fileIn = new FileInputStream("person.ser"); ObjectInputStream objectIn = new ObjectInputStream(fileIn)) { deserializedPerson = (Person) objectIn.readObject(); System.out.println("Person object deserialized successfully."); } catch (IOException | ClassNotFoundException e) { e.printStackTrace(); } } }
The above code reads the previously serialized Person
object from the person.ser
file and reconstructs the object using ObjectInputStream
.
Now that you've seen Java Serialization in action, you can use it in your applications to persist, transport, and deep copy objects with ease. Just remember to implement the Serializable
interface, and you're good to go!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).