MATLAB File I/O
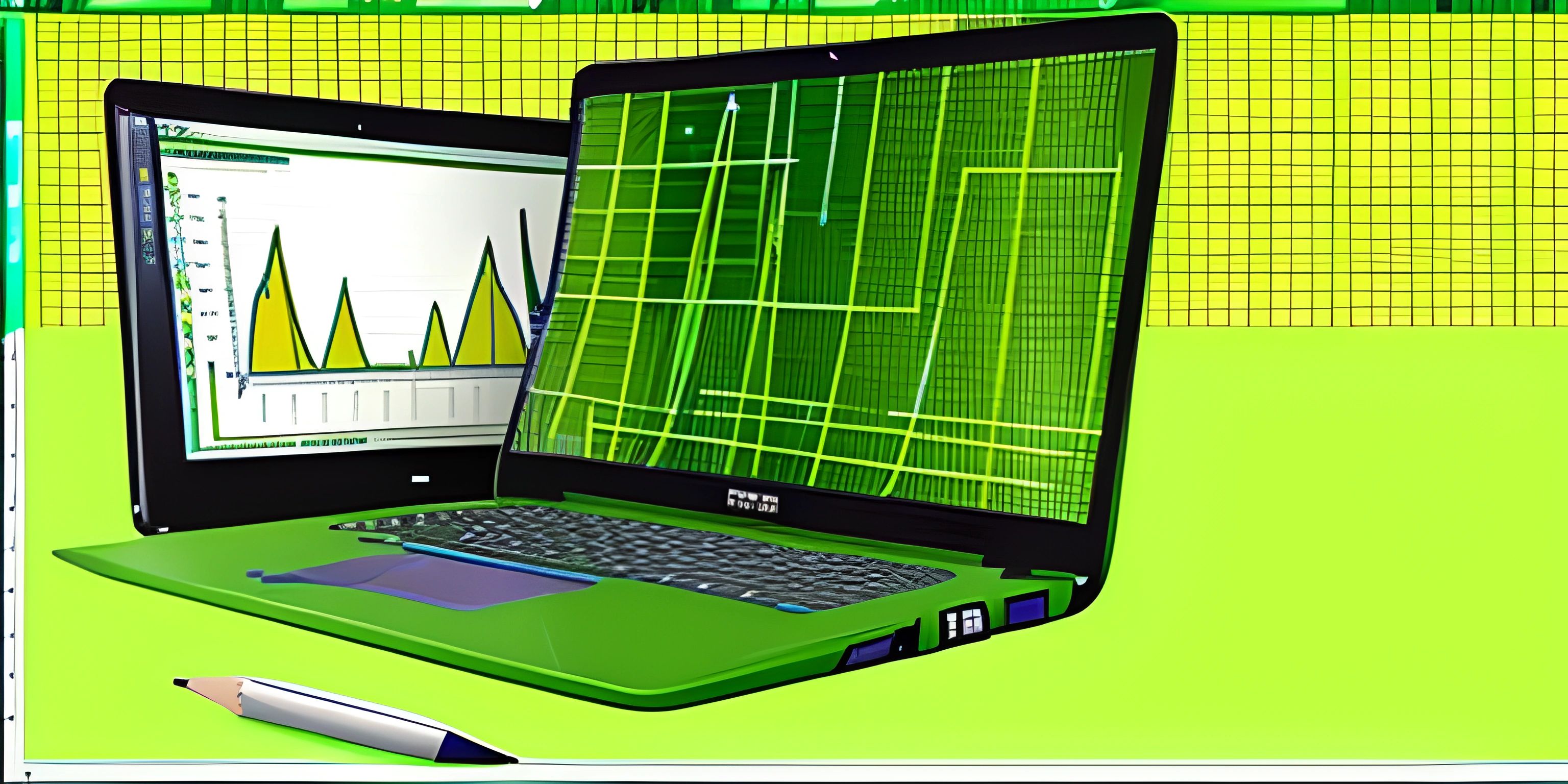
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
MATLAB provides a variety of functions for file input and output (I/O), making it easy to read and write data to and from files. We will cover both text and binary files, as well as best practices and tips for efficient file handling.
Text Files
Reading and writing data from text files is a common task in MATLAB. Let's dive into the main functions used for this purpose.
Opening and Closing Files
Before reading from or writing to a text file, you must open it using the fopen
function. fopen
returns a file identifier, which you'll use to reference the file in subsequent operations.
fileID = fopen('example.txt', 'r');
The first argument is the filename, and the second argument is the file access mode. In this case, 'r'
indicates that we want to open the file for reading. Other common modes include 'w'
for writing (overwriting any existing content) and 'a'
for appending (adding data to the end of the file).
After you're done with a file, it's important to close it using the fclose
function:
fclose(fileID);
Reading and Writing Text Files
To read data from a text file, you can use the fscanf
function. Here's an example of how to read a matrix of numbers from a file:
fileID = fopen('data.txt', 'r'); data = fscanf(fileID, '%f', [3, Inf]); fclose(fileID);
In this example, %f
is a format specifier that tells fscanf
to read floating-point numbers. The [3, Inf]
argument specifies that we want to read a matrix with three rows and as many columns as there are numbers in the file.
To write data to a text file, you can use the fprintf
function. Here's an example of how to write a matrix of numbers to a file:
fileID = fopen('output.txt', 'w'); fprintf(fileID, '%f\t', data); fclose(fileID);
This code writes the matrix data
to the file output.txt
, using tab characters (\t
) to separate the numbers.
Binary Files
While text files are human-readable, binary files store data more compactly and can be read and written more efficiently. MATLAB provides fread
and fwrite
functions for reading and writing binary files.
Reading and Writing Binary Files
To read data from a binary file, use the fread
function:
fileID = fopen('data.bin', 'r'); data = fread(fileID, [3, Inf], 'double'); fclose(fileID);
In this example, 'double'
is the data type specifier, indicating that we want to read 8-byte (64-bit) floating-point numbers.
To write data to a binary file, use the fwrite
function:
fileID = fopen('output.bin', 'w'); fwrite(fileID, data, 'double'); fclose(fileID);
This code writes the matrix data
to the file output.bin
using 8-byte floating-point numbers.
FAQ
When should I use text files vs. binary files in MATLAB?
Text files are human-readable and are easier to inspect and debug. However, binary files are more compact and efficient for storing large amounts of data. Use text files when you need to share data with other programs or users, and use binary files when you want to store large datasets more efficiently.
How do I handle errors when reading or writing files in MATLAB?
MATLAB provides error handling functions, such as try
, catch
, and finally
, which can be used to handle errors during file I/O. For example, you can use a try-catch
block to handle file opening errors and provide a helpful error message.
Can I read and write non-numeric data from text files?
Yes, MATLAB's fscanf
and fprintf
functions support various format specifiers for reading and writing non-numeric data, such as strings and characters. Check the MATLAB documentation for a full list of supported format specifiers.