Rust I/O Operations
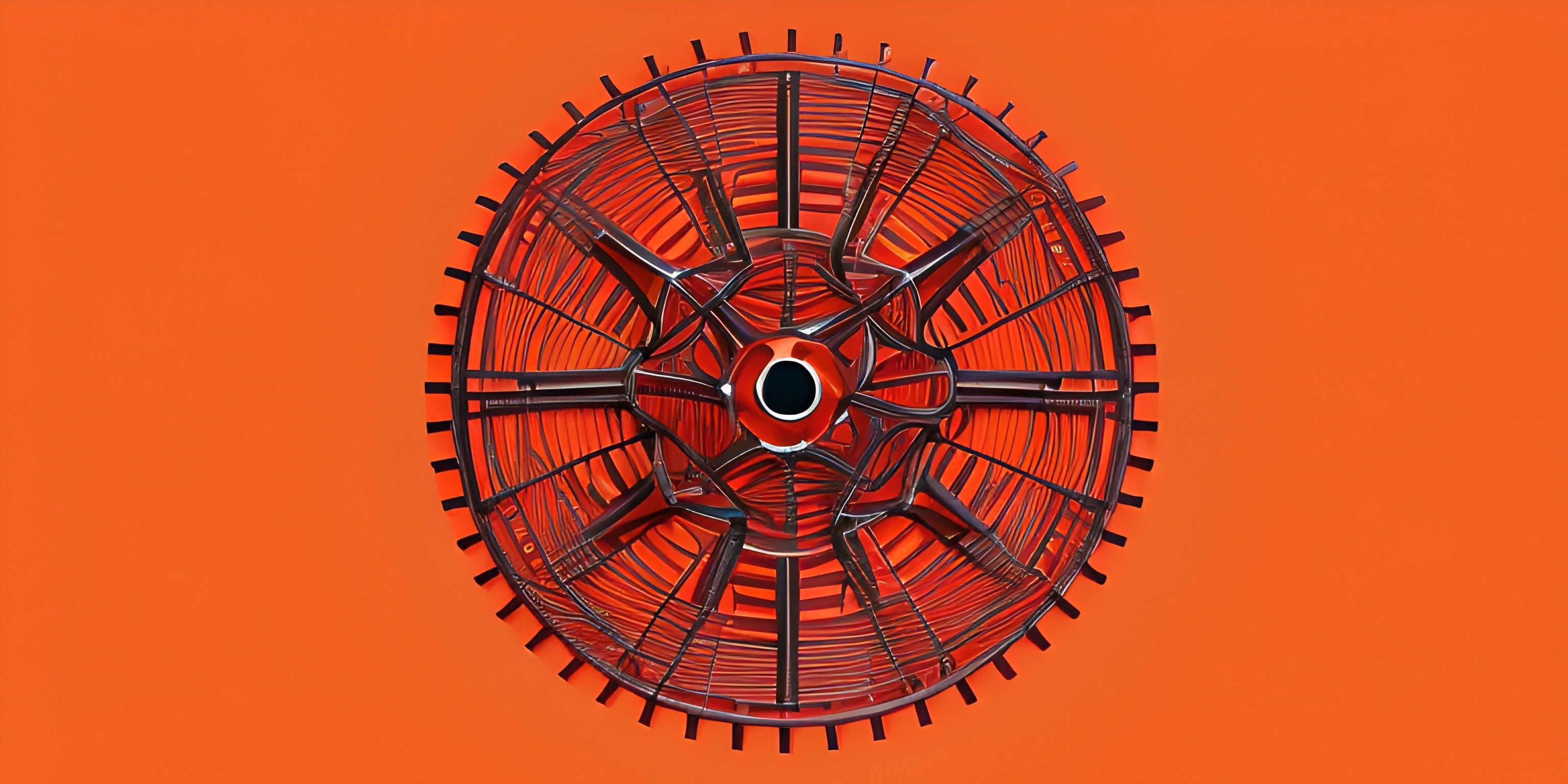
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Input and output (I/O) operations are essential in any programming language, including Rust. They allow your program to interact with the outside world, whether it's reading from or writing to files, or communicating with users through the command line.
User Input and Output
Let's start with the basics: getting user input and printing output to the command line. Rust provides a standard library, std
, which includes modules for handling I/O operations.
Printing Output
To print output to the console, we use the println!
macro. Here's a simple example:
fn main() { println!("Hello, Cratecode!"); }
In this example, the println!
macro prints the "Hello, Cratecode!" message, followed by a newline character.
Reading User Input
Now let's get some input from the user. To do this, we'll use the std::io
module, specifically the stdin()
function and the read_line()
method. Here's an example:
use std::io; fn main() { let mut input = String::new(); println!("What's your name?"); io::stdin() .read_line(&mut input) .expect("Failed to read line"); println!("Hello, {}!", input.trim()); }
In this example, we first create a mutable String
to store the user input. We then print a prompt asking for the user's name. Next, we use the stdin()
function to access the standard input and read the line using read_line()
. After that, we handle potential errors using the expect()
method. Finally, we print a personalized greeting using the input, trimming any leading or trailing whitespace.
File I/O
Reading from and writing to files is another common I/O operation. Rust provides the std::fs
module to interact with the file system.
Reading a File
To read the contents of a file, use the std::fs::read_to_string
function. Here's an example:
use std::fs; fn main() { let content = fs::read_to_string("input.txt") .expect("Failed to read file"); println!("File content:\n{}", content); }
This code reads the content of the "input.txt" file and prints it to the console. If there's an error reading the file, the expect()
method will handle it.
Writing to a File
To write data to a file, use the std::fs::write
function. Here's an example:
use std::fs; fn main() { let content = "This is some text to write to the file."; fs::write("output.txt", content) .expect("Failed to write to file"); println!("Text written to output.txt"); }
This code writes the content of the content
variable to a file named "output.txt". If the file doesn't exist, it will be created. If there's an error writing the file, the expect()
method will handle it.
Conclusion
Rust provides powerful I/O capabilities through its standard library, allowing for seamless interaction with users and the file system. With the std::io
and std::fs
modules, you can perform essential I/O operations such as reading and writing files and handling user input and output. Happy coding!
FAQ
What are the basic I/O operations in Rust?
The basic I/O operations in Rust include:
- Reading files
- Writing files
- Reading user input from the console
- Displaying output to the console
How can I read a file in Rust?
To read a file in Rust, you can use the std::fs::read_to_string
function. Here's an example:
use std::fs; fn main() { let content = fs::read_to_string("example.txt") .expect("Error reading the file"); println!("File content:\n{}", content); }
How can I write to a file in Rust?
To write to a file in Rust, you can utilize the std::fs::write
function. Here's a sample code:
use std::fs; fn main() { let content = "Hello, Rust!"; fs::write("output.txt", content) .expect("Error writing to the file"); println!("Content has been written to output.txt"); }
How do I read user input from the console in Rust?
You can read user input from the console using the std::io::stdin
function. Check out this example:
use std::io; fn main() { let mut input = String::new(); println!("Enter some text:"); io::stdin() .read_line(&mut input) .expect("Error reading user input"); println!("You entered: {}", input); }
How do I display output to the console in Rust?
To display output to the console in Rust, you can use the println!
macro. Here's a simple example:
fn main() { let message = "Hello, Rust!"; println!("{}", message); }