Understanding Packages in Java
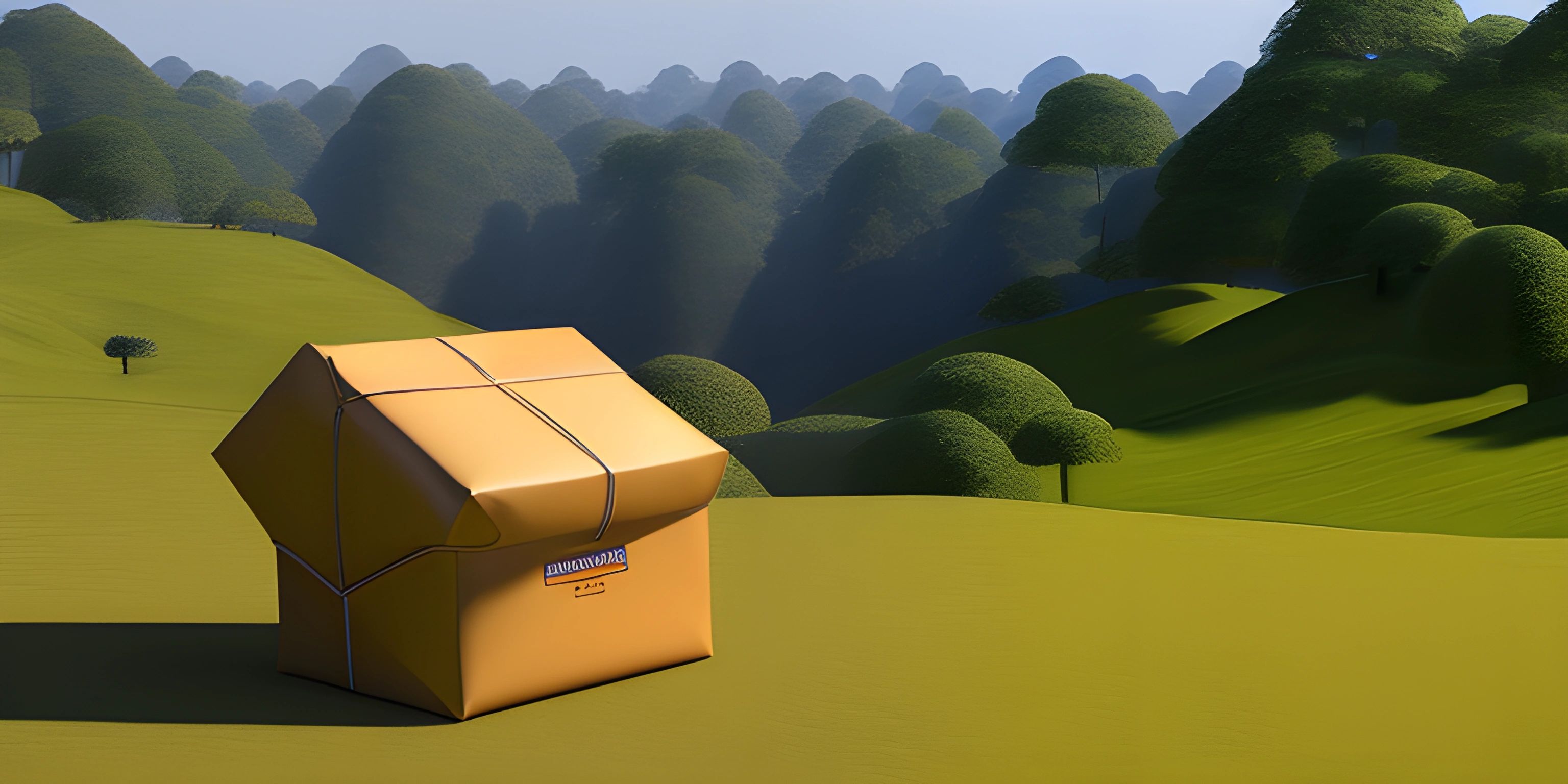
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Java packages are a fundamental aspect of Java programming, providing a way to organize and manage code. They are essential for maintaining a clean and understandable codebase, especially as a project grows in size and complexity. In this article, we'll dive into the world of Java packages, exploring their importance, structure, and how to use them effectively.
What are Java Packages?
Packages in Java are essentially namespaces that serve as containers for classes and interfaces. They provide a hierarchical structure for organizing code, allowing developers to group related classes and interfaces together. This organization helps to avoid naming conflicts, promote code reusability, and makes it easier to locate and maintain code.
Package Naming Conventions
When creating packages, it's crucial to follow the Java package naming conventions. These conventions are in place to ensure consistency and avoid naming conflicts. Java package names are typically all lowercase and use a reverse domain name as their prefix. For example, if your domain name is example.com
, your package name should start with com.example
. This helps to ensure package names are unique and easy to understand.
Additionally, package names should be meaningful and reflect the purpose or functionality of the classes and interfaces they contain. For example, com.example.database
would be an appropriate package name for classes related to database management.
Creating and Using Packages
To create a package in Java, you simply need to define it at the beginning of your Java file using the package
keyword, followed by the package name. For example:
package com.example.database; public class DatabaseManager { // Class implementation goes here }
This code snippet defines a class named DatabaseManager
inside the com.example.database
package.
To use a class or interface from another package, you need to import
it. The import
keyword is followed by the fully qualified name of the class or interface you wish to use. Here's an example:
package com.example.application; import com.example.database.DatabaseManager; public class Application { public static void main(String[] args) { DatabaseManager dbManager = new DatabaseManager(); // Use the DatabaseManager class here } }
In this example, we import the DatabaseManager
class from the com.example.database
package and use it in our Application
class.
Organizing Large Projects
As your Java project grows, it's essential to keep your codebase organized. By structuring your packages hierarchically, you can create a logical organization that's easy to understand and maintain. For example, you might have packages like com.example.database
, com.example.ui
, and com.example.networking
that each contain code related to specific functionalities.
By effectively using packages, you can keep your codebase organized, maintainable, and easy to navigate. They are an essential tool for Java developers and should be used consistently and thoughtfully.
FAQ
What are Java packages and why are they important?
Java packages are namespaces that serve as containers for classes and interfaces. They help to organize code, avoid naming conflicts, and promote code reusability. Packages are important in maintaining a clean and understandable codebase, especially as a project grows in size and complexity.
What are some best practices when it comes to naming Java packages?
Java package names should follow naming conventions, such as using all lowercase letters and a reverse domain name as a prefix (e.g., com.example
). Additionally, package names should be meaningful and reflect the purpose or functionality of the classes and interfaces they contain.
How do I create a package and use classes from other packages?
To create a package, define it at the beginning of your Java file using the package
keyword, followed by the package name. To use a class or interface from another package, import it using the import
keyword, followed by the fully qualified name of the class or interface.