Understanding Data Types in JavaScript
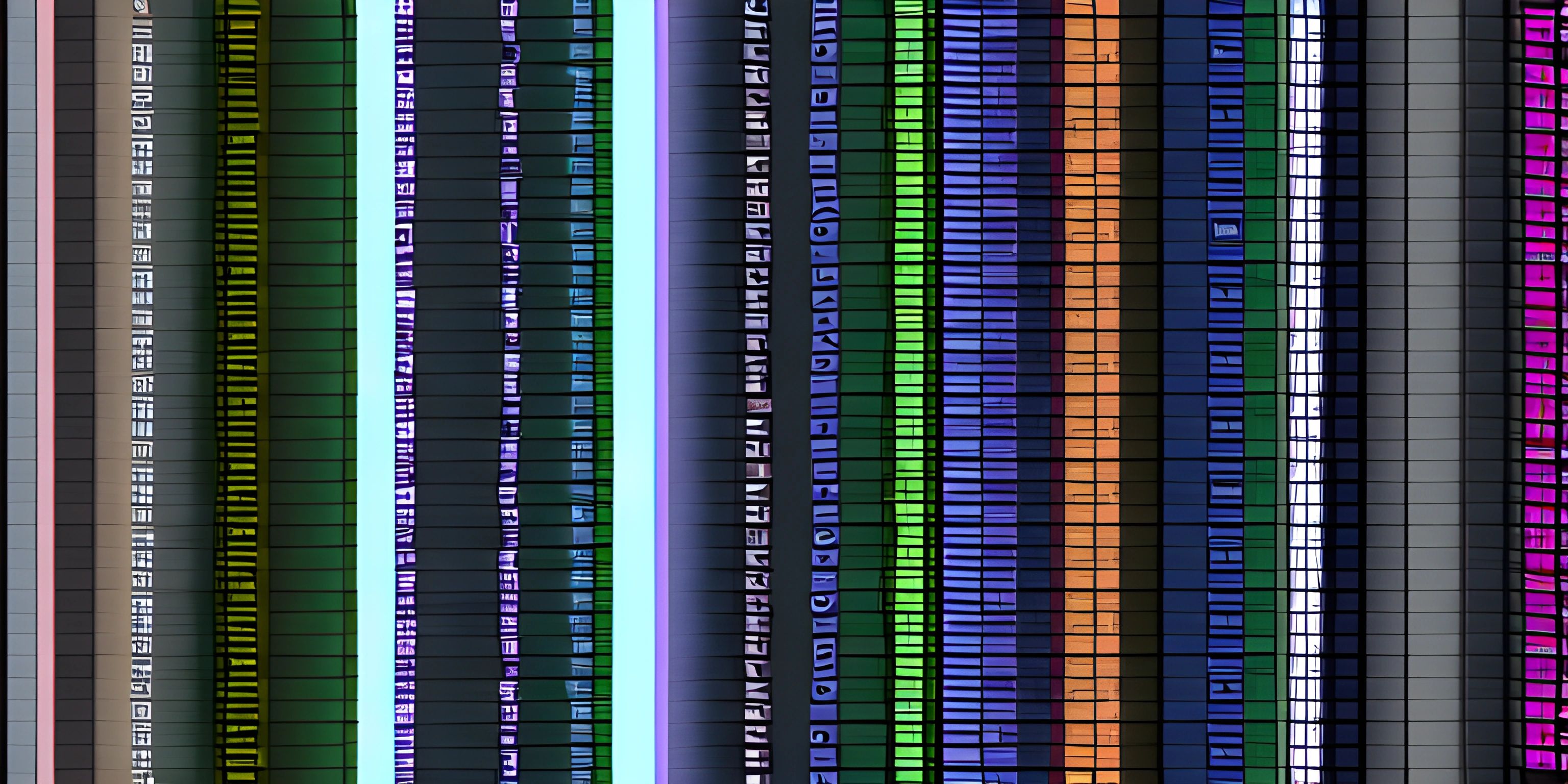
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Imagine you have a massive toolbox, but each tool looks almost identical. Without knowing which tool is used for what, you might end up using a wrench to hammer a nail. That's the chaos we'd face in programming without understanding data types! Data types are the building blocks of any programming language, and in JavaScript, they play a crucial role in how your code operates and performs.
What are Data Types?
In JavaScript, data types are essentially categories of data that tell the compiler or interpreter how the programmer intends to use the data. It's like a label that tells you whether you can eat something, drive it, or use it to build a treehouse.
Primitive Data Types
Primitive data types are the most basic types of data. They're like the fundamental particles in the world of programming. Here are the primitive data types in JavaScript:
- Number: Represents both integer and floating-point numbers.
- String: Represents a sequence of characters (text).
- Boolean: Represents true or false.
- Null: Represents an intentional absence of any object value.
- Undefined: Represents a variable that has been declared but not assigned a value.
- Symbol: Represents a unique and immutable value (ES6 and later).
Let's dive into each one with examples!
Number
The Number data type is pretty straightforward. It includes all integer and floating-point numbers.
let integerNumber = 42; // Integer let floatingNumber = 3.14; // Floating-point number
JavaScript also provides some special numerical values such as Infinity
, -Infinity
, and NaN
(Not-a-Number).
console.log(1 / 0); // Infinity console.log(-1 / 0); // -Infinity console.log("Hello" * 2); // NaN
String
A String is a sequence of characters used to represent text. You can use double quotes, single quotes, or backticks for template literals.
let doubleQuoteString = "Hello, World!"; let singleQuoteString = 'JavaScript is fun!'; let templateLiteralString = `The answer is ${integerNumber}`;
Strings in JavaScript come with a plethora of methods, like toUpperCase()
, toLowerCase()
, and substring()
, to manipulate and interact with text.
Boolean
Boolean data types are quite binary in nature, representing only two values: true
or false
.
let isJavaScriptFun = true; let isHomeworkDone = false;
Booleans are often used in conditional statements for control flow.
Null and Undefined
At first glance, null
and undefined
might seem similar, but they serve different purposes.
- Undefined: This means a variable has been declared but not yet assigned a value.
let notAssigned; console.log(notAssigned); // undefined
- Null: This represents the intentional absence of any object value.
let emptyValue = null; console.log(emptyValue); // null
Symbol
Symbols were introduced in ECMAScript 6 (ES6) and represent a unique and immutable value. They're often used to identify object properties uniquely, avoiding name collisions.
let uniqueId = Symbol('id'); console.log(uniqueId); // Symbol(id)
BigInt
Introduced in ES2020, BigInt is used for arbitrary-precision integers. They're useful when dealing with numbers larger than the Number.MAX_SAFE_INTEGER
.
let bigIntValue = 1234567890123456789012345678901234567890n; console.log(bigIntValue); // 1234567890123456789012345678901234567890n
Reference Data Types
Unlike primitive data types, reference data types can store collections of data and more complex entities. The most common reference data types in JavaScript are Objects and Arrays.
Objects
An Object is a collection of properties, where each property is a key-value pair. Think of it as a mini-database.
let person = { firstName: "John", lastName: "Doe", age: 30, isEmployed: true }; console.log(person.firstName); // John
Objects can be nested and can store functions, making them very flexible.
Arrays
An Array is an ordered collection of items that can store multiple values in a single variable. Arrays can hold different data types, including other arrays.
let colors = ["red", "green", "blue"]; console.log(colors[0]); // red let mixedArray = [42, "hello", true, null]; console.log(mixedArray[1]); // hello
Functions
Though technically not a data type, functions can be treated as values in JavaScript. They can be assigned to variables, passed as arguments, and returned from other functions.
function greet(name) { return `Hello, ${name}!`; } let greeting = greet("Alice"); console.log(greeting); // Hello, Alice!
Type Coercion
JavaScript is a loosely typed language, meaning it can automatically convert data types when needed. This is known as type coercion, and it can lead to some unexpected results.
console.log('5' + 5); // 55 (String concatenation) console.log('5' - 2); // 3 (String converted to Number)
Understanding how type coercion works ensures you write code that behaves as expected.
Type Checking
Knowing the type of data you're working with can prevent bugs. JavaScript provides the typeof
operator for this purpose.
console.log(typeof 42); // number console.log(typeof 'Hello'); // string console.log(typeof true); // boolean console.log(typeof undefined); // undefined console.log(typeof null); // object (this is a historical quirk) console.log(typeof Symbol('id')); // symbol console.log(typeof {name: "Alice"}); // object
For arrays and null, which both return object
, you might use additional checks.
console.log(Array.isArray([1, 2, 3])); // true console.log(null === null); // true
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Data Types (psst, it's free!).
FAQ
What are the primitive data types in JavaScript?
Primitive data types in JavaScript include Number, String, Boolean, Null, Undefined, Symbol, and BigInt. These are the basic building blocks of data in the language.
What is the difference between null and undefined?
Undefined
indicates a variable that has been declared but not assigned a value, whereas null
represents an intentional absence of any object value.
How does type coercion work in JavaScript?
JavaScript automatically converts data types when required, which can lead to unexpected results. For instance, adding a string and a number concatenates them as a string, while subtracting converts the string to a number.
What is a Symbol in JavaScript?
A Symbol is a unique and immutable data type introduced in ES6, often used to create unique object property keys to avoid name collisions.
How can you check the type of a variable in JavaScript?
You can use the typeof
operator to check the type of a variable. For arrays and null, additional checks like Array.isArray()
and strict comparison (===
) are often used.