Data Structures in Java
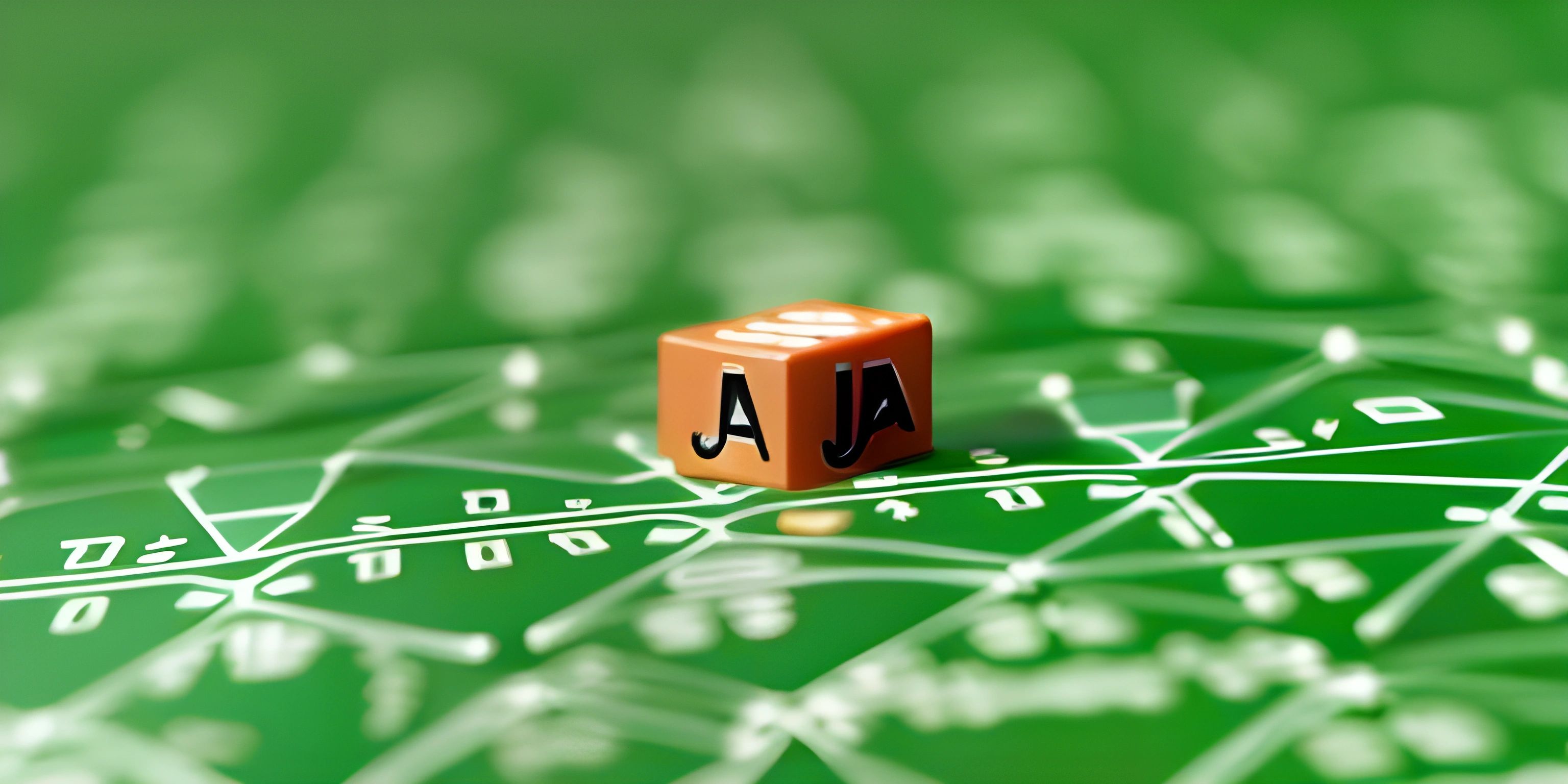
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Dive into the world of Java data structures and discover the tools that help you store and manage data in your programs. Let's unravel the mystery of arrays, lists, sets, and maps, and see how they can make your life easier as a Java programmer.
Arrays
In Java, an array is a fixed-size container that can hold elements of a single data type. It's an efficient way to store data that has a known quantity. Here's how you can create a simple integer array:
int[] myArray = new int[5]; myArray[0] = 42; myArray[1] = 7; // ... and so on
You can also initialize an array with values like this:
int[] myArray = {42, 7, 13, 29, 64};
Though arrays are handy, their fixed size can be limiting. Luckily, Java offers more flexible data structures, such as lists.
Lists
A list is a dynamic data structure that can grow or shrink in size as needed. Java has two main types of lists: ArrayList and LinkedList.
ArrayList
An ArrayList
is a resizable array that provides fast access to elements by their index. It's a great choice when you need to access elements frequently but don't need to insert or remove elements often. Here's how to create an ArrayList:
import java.util.ArrayList; ArrayList<Integer> myList = new ArrayList<>(); myList.add(42); myList.add(7); myList.add(13);
LinkedList
A LinkedList
is a doubly-linked list that offers fast insertion and removal of elements. It's ideal when you need to add or remove elements frequently but don't need to access them by index often. Here's how to create a LinkedList:
import java.util.LinkedList; LinkedList<Integer> myList = new LinkedList<>(); myList.add(42); myList.add(7); myList.add(13);
Sets
A set is a collection of unique elements that have no particular order. It's perfect for situations where you need to store a group of distinct items. Java provides two primary types of sets: HashSet and TreeSet.
HashSet
A HashSet
stores elements using a hash table, which offers constant-time performance for basic operations like adding, removing, and searching elements. Here's how to create a HashSet:
import java.util.HashSet; HashSet<Integer> mySet = new HashSet<>(); mySet.add(42); mySet.add(7); mySet.add(13);
TreeSet
A TreeSet
is a sorted set implemented as a balanced binary search tree. It stores elements in a sorted order, which can be useful when you need to keep your data structured. Here's how to create a TreeSet:
import java.util.TreeSet; TreeSet<Integer> mySet = new TreeSet<>(); mySet.add(42); mySet.add(7); mySet.add(13);
Maps
A map is a data structure that stores key-value pairs, allowing you to associate a value with a unique key. Java offers two main types of maps: HashMap and TreeMap.
HashMap
A HashMap
is an efficient map implementation that uses a hash table to store key-value pairs. It offers constant-time performance for basic operations. Here's how to create a HashMap:
import java.util.HashMap; HashMap<String, Integer> myMap = new HashMap<>(); myMap.put("one", 1); myMap.put("two", 2); myMap.put("three", 3);
TreeMap
A TreeMap
is a sorted map implemented as a balanced binary search tree. It stores key-value pairs in a sorted order based on their keys. Here's how to create a TreeMap:
import java.util.TreeMap; TreeMap<String, Integer> myMap = new TreeMap<>(); myMap.put("one", 1); myMap.put("two", 2); myMap.put("three", 3);
Now that you've explored various Java data structures and their use cases, you're equipped to tackle problems with the right tools at your disposal. Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Structs and Traits (psst, it's free!).
FAQ
What are the primary data structures in Java?
The primary data structures in Java include arrays, lists (ArrayList and LinkedList), sets (HashSet and TreeSet), and maps (HashMap and TreeMap).
When should I use an ArrayList?
Use an ArrayList when you need to access elements frequently by their index but don't need to insert or remove elements often, as it provides fast access to elements. It's a dynamic data structure that can grow or shrink in size as needed.
What is the difference between a HashMap and a TreeMap?
The main difference between a HashMap and a TreeMap is their underlying implementation and order. A HashMap is an efficient map implementation that uses a hash table to store key-value pairs and offers constant-time performance for basic operations. A TreeMap is a sorted map implemented as a balanced binary search tree, storing key-value pairs in a sorted order based on their keys.