Memoization Deep Dive
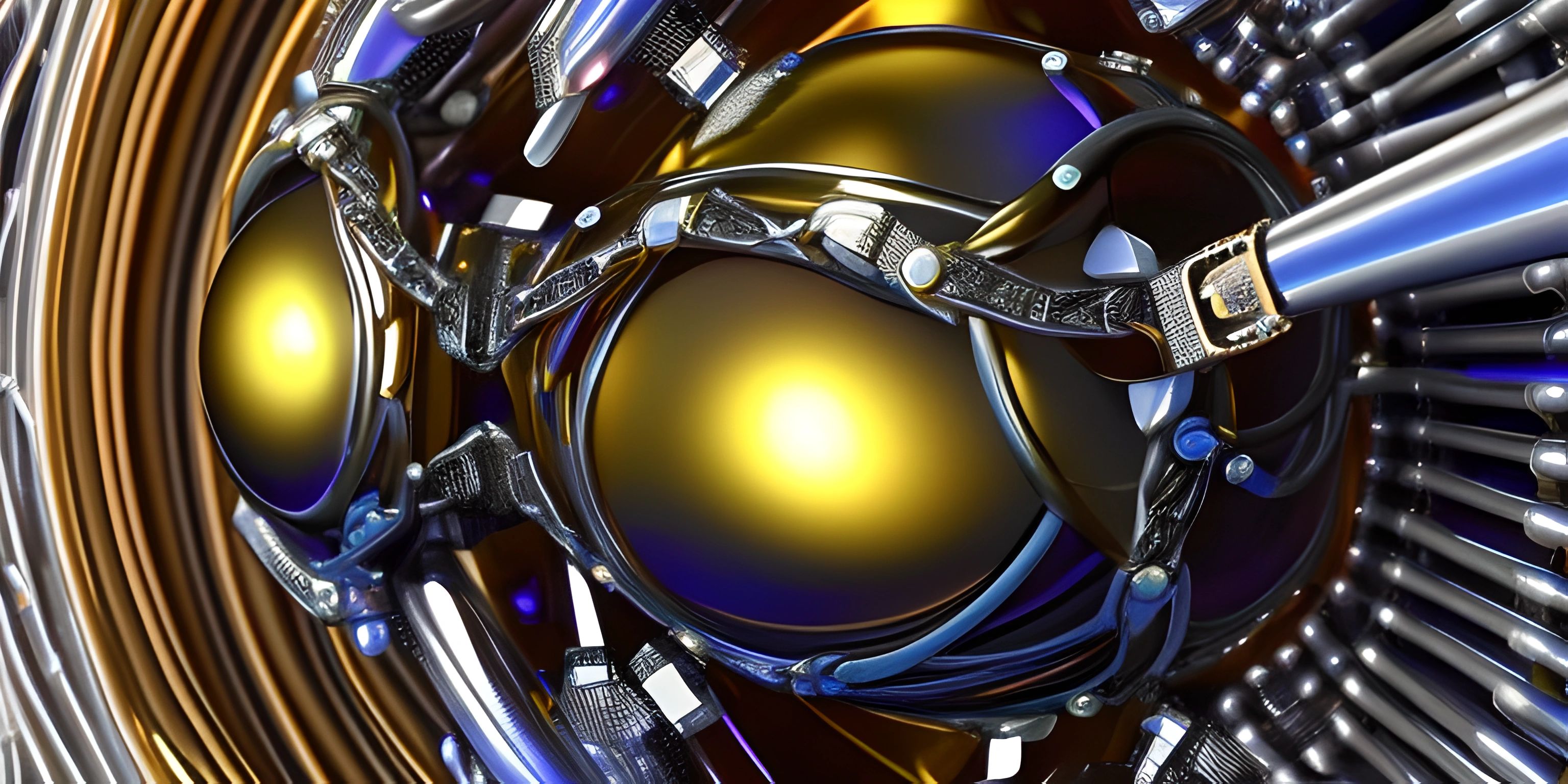
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Imagine you're running a restaurant and need to calculate the total cost of your daily ingredients. Each ingredient is used in multiple dishes, so you calculate the cost for each dish and sum them up. But after a while, you realize you're calculating the cost of the same ingredient multiple times. What a waste of brain power! If only there was a way to remember the cost of each ingredient so you don't have to recalculate it every time...
Well, in programming, there's a technique called memoization that does exactly this. It's a way to store the results of expensive function calls and return the cached result when the same inputs occur again. This can significantly improve the performance of your code, especially in cases where you have recursive functions or complex calculations.
How Memoization Works
Memoization is an optimization technique that involves caching the results of function calls. In essence, you create a "memory" of sorts that stores the results of previous calculations. When a function is called with the same inputs again, instead of recalculating the result, you simply look it up in the cache and return the stored value.
Here's a basic example using pseudocode:
function expensiveCalculation(x): if x is in cache: return cache[x] else: result = perform calculation on x cache[x] = result return result
Memoization can be particularly useful when dealing with recursive functions, where the same function is called multiple times with the same inputs.
Fibonacci Example
The Fibonacci sequence is a classic example of a problem that can benefit from memoization. The sequence is defined as:
F(0) = 0 F(1) = 1 F(n) = F(n-1) + F(n-2) for n > 1
Here's how a simple recursive implementation would look like without memoization:
function fibonacci(n): if n == 0: return 0 elif n == 1: return 1 else: return fibonacci(n-1) + fibonacci(n-2)
This implementation has an exponential time complexity, which means it's incredibly slow for large values of n
. However, we can use memoization to optimize the performance:
function memoizedFibonacci(n, cache = {}): if n == 0: return 0 elif n == 1: return 1 elif n in cache: return cache[n] else: result = memoizedFibonacci(n-1, cache) + memoizedFibonacci(n-2, cache) cache[n] = result return result
By caching the results of previous calculations, we can dramatically reduce the number of calls to fibonacci
, resulting in a much faster execution.
When to Use Memoization
Memoization is a powerful technique, but it's not a magic bullet. Here are some things to consider when deciding whether to use memoization:
- Function calls with the same inputs: Memoization is only useful when a function is called multiple times with the same inputs. If the inputs are always different, caching the results won't provide any benefits.
- Expensive calculations: Memoization is most effective when the function being memoized involves a complex or time-consuming calculation. If the function is already very fast, the overhead of caching may negate any performance improvements.
- Memory usage: Storing the results of previous calculations can consume a significant amount of memory, especially for large inputs or long-running programs. Be mindful of the memory implications when using memoization.
In conclusion, memoization is a valuable optimization technique that can greatly boost the performance of your code in certain scenarios. Like a savvy restaurant owner, you can save time and effort by remembering the results of your previous work and using them to your advantage.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is memoization in programming?
Memoization is a technique used in programming to optimize the execution time of a function by caching its results for a given set of input parameters. If the function is called again with the same parameters, the cached result is returned instead of recalculating the result. This can significantly improve the performance of functions with expensive computations, especially when they are called repeatedly with the same inputs.
How does memoization work in practice?
Memoization is typically implemented using a dictionary, hash table or some other data structure that can store key-value pairs. When a memoized function is called, it first checks whether the result for the given input parameters is already stored in the cache. If yes, the cached result is returned, otherwise the function calculates the result, stores it in the cache, and returns the result. Here's an example of memoization in Python using a dictionary:
def memoized_fibonacci(n, cache={}): if n in cache: return cache[n] elif n <= 1: result = n else: result = memoized_fibonacci(n - 1) + memoized_fibonacci(n - 2) cache[n] = result return result
When should I use memoization in my code?
Memoization is most beneficial when applied to functions that have the following characteristics:
- Expensive computations: The function performs time-consuming calculations, so caching the results can save significant time.
- Repeated calls with the same inputs: The function is called multiple times with the same set of parameters, which makes caching results more effective.
- Pure functions: The function's output depends solely on its input parameters, and it does not have any side effects. This ensures that the cached results remain valid for future calls.
Are there any downsides to using memoization?
While memoization can significantly improve the performance of certain functions, it also has a few downsides:
- Increased memory usage: Storing results in a cache consumes memory, which can be a concern if you're working with limited resources or if the cache grows too large.
- Cache management: Depending on the implementation, you may need to manage the cache to prevent it from growing indefinitely, either by using a fixed-size cache, implementing a cache eviction strategy, or manually clearing the cache when it's no longer needed.
Can I use memoization with recursive functions?
Yes, memoization can be especially beneficial for recursive functions with overlapping subproblems, such as the Fibonacci sequence calculation shown in the example above. By caching the results of intermediate subproblems, you can avoid redundant calculations and significantly speed up the execution of the function.