Optimizing React App Performance
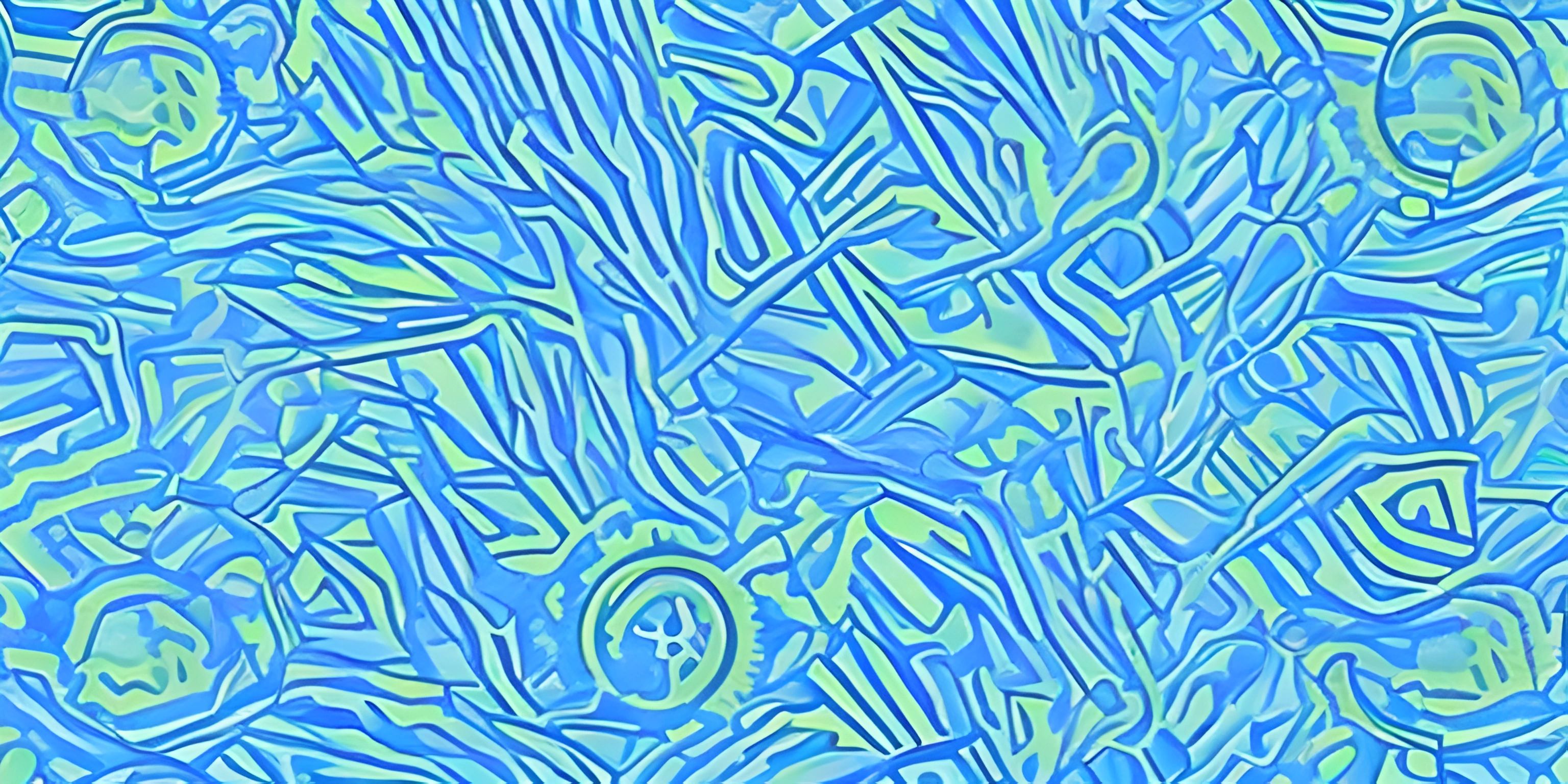
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
A fast, responsive user interface is vital for any web application, and as developers, we strive to achieve that. React, being a powerful library for building user interfaces, offers several techniques to help optimize the performance of your applications. Let's dive into some common optimization techniques, such as memoization, PureComponent, and code splitting.
Memoization
Memoization is a technique where you cache the results of function calls and return the cached result when the same inputs occur again. In the context of React, memoization can be done using the React.memo
higher-order component.
React.memo
performs a shallow comparison of the previous and current props to decide if a functional component should re-render. If the props are the same, it skips the re-render and returns the memoized result. This can help in reducing unnecessary re-renders and improving the performance of your app.
Here's a simple example of using React.memo
:
import React from "react"; const MyComponent = React.memo(function MyComponent(props) { // Your component logic goes here }); export default MyComponent;
PureComponent
For class components, React provides PureComponent
which automatically implements the shouldComponentUpdate
lifecycle method. PureComponent
performs a shallow comparison of the previous and current props and state, similar to React.memo
. If the comparison returns false
, the component is not re-rendered, thus improving performance.
Here's a simple example of using PureComponent
:
import React, { PureComponent } from "react"; class MyComponent extends PureComponent { // Your component logic goes here } export default MyComponent;
Keep in mind that PureComponent
only performs a shallow comparison, so if your component has complex data structures like nested objects, you might need to implement a custom shouldComponentUpdate
method for deep comparisons.
Code Splitting
Code splitting is another optimization technique that allows you to split your code into smaller chunks, which can be loaded on demand. This helps in reducing the initial load time of your app by only loading the necessary code for the current view.
React supports code splitting with the help of React.lazy
and React.Suspense
. React.lazy
is a function that allows you to load a component lazily as a separate chunk when it's needed, while React.Suspense
is a wrapper component that displays a fallback content until the component is loaded.
Here's a simple example of using code splitting in React:
import React, { lazy, Suspense } from "react"; const LazyComponent = lazy(() => import("./LazyComponent")); function App() { return ( <div> <Suspense fallback={<div>Loading...</div>}> <LazyComponent /> </Suspense> </div> ); } export default App;
In this example, LazyComponent
is loaded lazily as a separate chunk when it's rendered, and the fallback content "Loading..." is displayed until the component is loaded completely.
These are just a few of the many techniques available to optimize the performance of your React applications. By utilizing memoization, PureComponent, and code splitting, you can significantly improve the responsiveness and user experience of your app. Don't forget to measure your app's performance using React DevTools to identify bottlenecks and potential areas for optimization. Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Full-stack Web Frameworks (Next.js) (psst, it's free!).