Memoization Overview
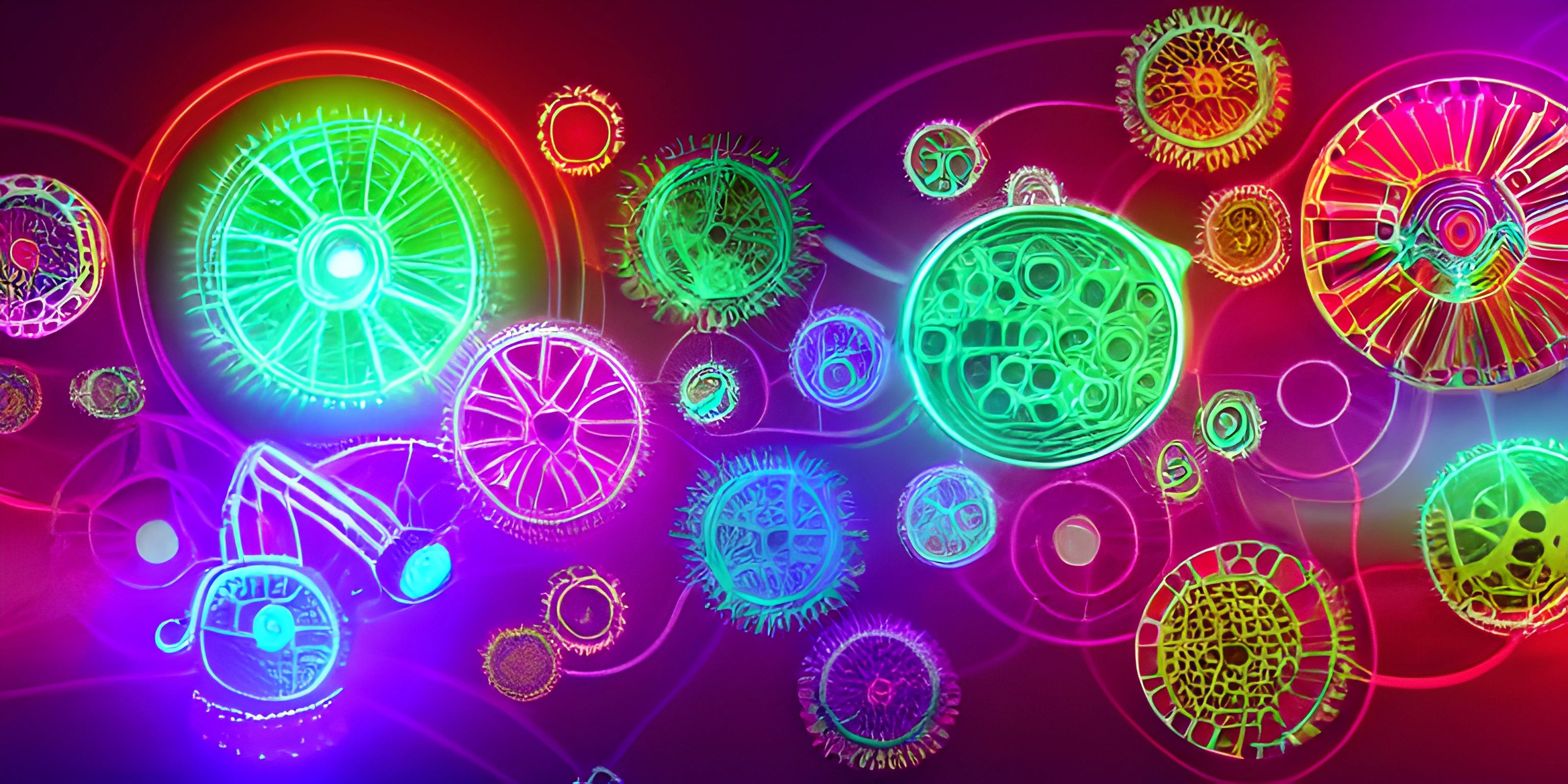
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Memoization is a technique used in programming to speed up the execution of a function by caching its results for specific input values. In other words, it's like a super-powered memory bank that allows programs to recall previous computations and avoid redundant work.
Why Memoize?
Imagine you're a student tasked with solving a series of math problems. With each problem, you have to perform the same set of calculations. After a while, you start to recognize the patterns and realize you've already done some of these calculations before. Instead of re-doing them, you could simply write down the results and consult your notes when you encounter the same calculations again. This is essentially what memoization does for your code.
By utilizing memoization, your program can significantly reduce the time it takes to run functions that involve repetitive calculations, especially in the case of recursive functions that tend to be called multiple times with the same input values.
How Does Memoization Work?
Memoization involves caching the results of function calls and using these cached values when the same function is called again with the same input parameters. This means that, instead of re-computing the result, your program can simply look it up in the cache, making the overall execution faster.
Here's a simple example of a Fibonacci sequence function without memoization:
def fib(n): if n <= 1: return n else: return fib(n - 1) + fib(n - 2)
The function above is inefficient because it recalculates the Fibonacci sequence for each call, leading to exponential growth in the number of calls. To improve its performance, we can implement memoization:
def fib_memoized(n, memo={}): if n <= 1: return n if n not in memo: memo[n] = fib_memoized(n - 1) + fib_memoized(n - 2) return memo[n]
With the memoized version, our function stores the results of previous calculations in a dictionary called memo
, using the input value as the key. When the function is called again with the same input, it returns the cached result instead of recalculating it.
Built-in Memoization in Programming Languages
Many programming languages provide built-in support for memoization. For example, Python offers a decorator called functools.lru_cache
, which can be used to easily add memoization to a function:
from functools import lru_cache @lru_cache def fib_lru_cache(n): if n <= 1: return n else: return fib_lru_cache(n - 1) + fib_lru_cache(n - 2)
In this case, the @lru_cache
decorator handles the caching and retrieval of results, making the implementation of memoization even more straightforward.
Conclusion
Memoization is a powerful technique that can greatly improve the performance of your programs, especially when dealing with functions that involve repetitive calculations. By caching the results of previous function calls and avoiding redundant work, memoization can help you write more efficient and optimized code. It's like giving your program a photographic memory, enabling it to breeze through complex calculations with ease.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Full-stack Web Frameworks (Next.js) (psst, it's free!).
FAQ
What is memoization and how does it optimize performance?
Memoization is a technique where you store the results of expensive function calls and return the cached result when the same inputs occur again. This improves the performance of a program by reducing the time spent on redundant calculations.
Can you provide a simple example of memoization in practice?
Certainly! Here's a basic example in Python, where we use memoization to optimize the calculation of Fibonacci numbers:
def memoize(f): cache = {} def wrapped(n): if n not in cache: cache[n] = f(n) return cache[n] return wrapped @memoize def fibonacci(n): if n <= 1: return n else: return fibonacci(n-1) + fibonacci(n-2) print(fibonacci(10)) # Output: 55
When is it appropriate to use memoization?
Memoization is most effective when used in functions that have a high time complexity and are called multiple times with the same inputs. This is because memoization can significantly reduce the time spent on repeated calculations, leading to an overall performance improvement.
Can memoization have any downsides or limitations?
Yes, there are some potential downsides to memoization:
- Memory usage: Memoization can increase memory usage because it stores the results of function calls. If you're dealing with a large number of cached results, this might lead to memory issues.
- Not always applicable: Memoization is not useful for functions with non-deterministic outputs, where the result depends on external factors or randomness.
Are there any built-in memoization tools in programming languages?
Yes, many programming languages offer built-in support for memoization. For example, in Python, you can use the functools.lru_cache
decorator to implement memoization easily:
from functools import lru_cache @lru_cache(maxsize=None) def fibonacci(n): if n <= 1: return n else: return fibonacci(n-1) + fibonacci(n-2) print(fibonacci(10)) # Output: 55
Keep in mind that the built-in memoization tools might have some limitations or different behaviors, so make sure to consult the documentation of the language you're using.