MongoDB Aggregation Operations
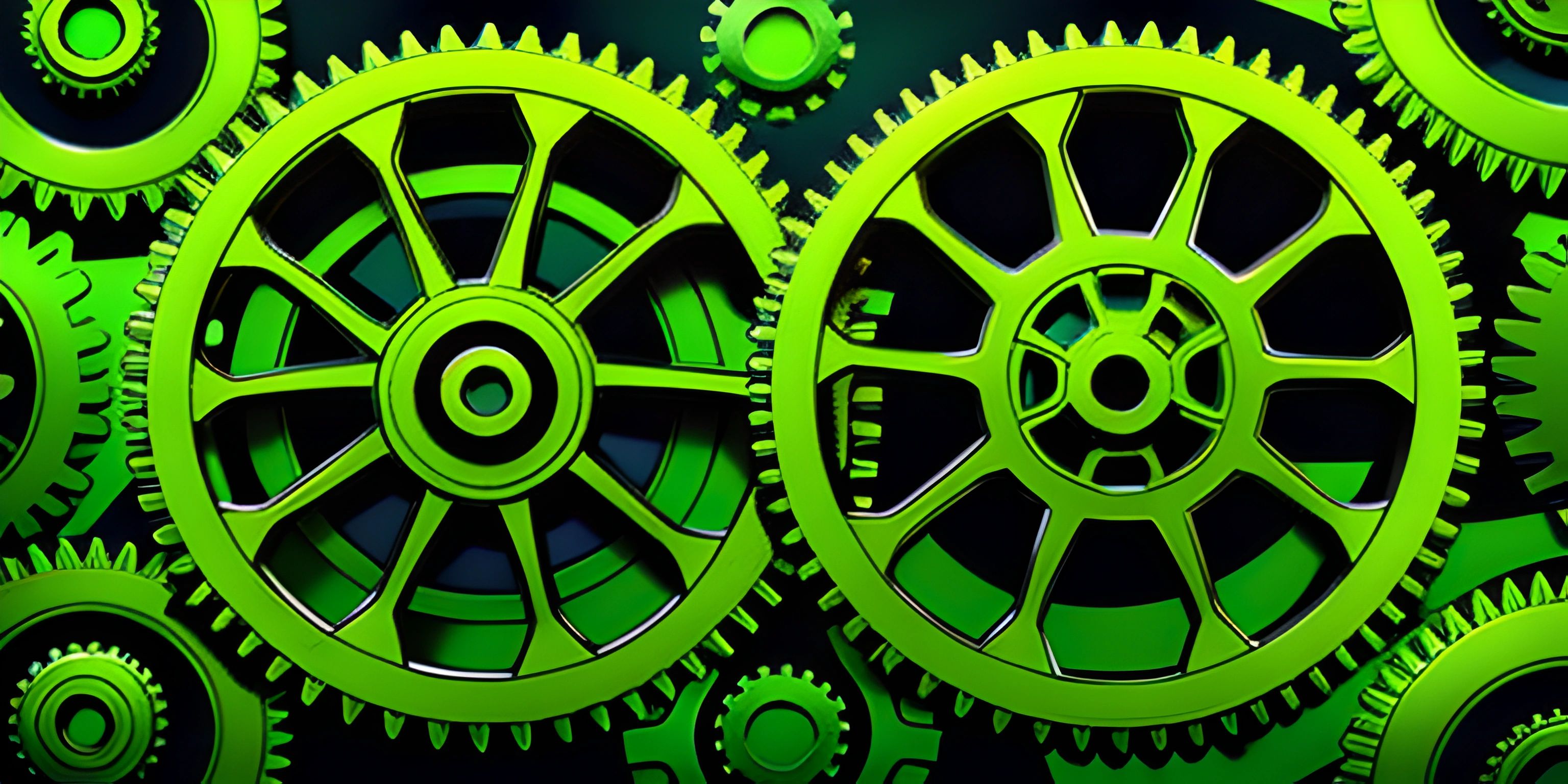
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
MongoDB, the popular NoSQL database, offers powerful tools to process and transform your data. One such tool is the aggregation framework, which allows you to perform complex data manipulation tasks in an efficient and straightforward manner. By combining various aggregation operations, you can create pipelines to transform your data into the desired format or perform calculations on it.
Aggregation Basics
Aggregation in MongoDB works by processing data records and returning computed results. Aggregation operations group values from multiple documents together and perform a variety of operations on the grouped data to return a single result.
Aggregation Pipeline
The aggregation pipeline is a powerful feature of MongoDB that allows you to perform complex data manipulation in a sequence of stages. Each stage in the pipeline processes the data and passes the transformed data to the next stage.
To create an aggregation pipeline, you use the aggregate()
method on a MongoDB collection. Here's a simple example:
db.sales.aggregate([ { $match: { status: "A" } }, { $group: { _id: "$customer_id", total: { $sum: "$amount" } } }, { $sort: { total: -1 } } ]);
This pipeline consists of three stages:
$match
filters the documents with thestatus
field equal to "A."$group
groups the documents bycustomer_id
and calculates the total amount for each group.$sort
sorts the results in descending order by thetotal
field.
Aggregation Operations
MongoDB provides a rich set of aggregation operations that you can use in your pipelines. Some of the most common operations include:
$match
: Filters the documents to pass only those that match the specified condition.$group
: Groups documents by a specified expression and applies an accumulator to each group.$project
: Reshapes the documents by adding or removing fields, renaming fields, or creating new fields from existing ones.$sort
: Sorts the documents by one or more fields.$limit
: Limits the number of documents passed to the next stage in the pipeline.$skip
: Skips a specified number of documents before passing the remaining documents to the next stage.
Example: Aggregating Sales Data
Let's walk through a practical example of using MongoDB aggregation operations to process sales data. Consider a sales
collection with documents that have the following structure:
{ "_id": ObjectId("..."), "date": ISODate("2021-01-01"), "product_id": 1, "customer_id": 123, "quantity": 5, "price": 10.99 }
Let's say we want to calculate the total sales for each product for the year 2021. We can create an aggregation pipeline with the following stages:
$match
to filter documents with adate
within the year 2021.$group
to group documents byproduct_id
and calculate the total sales using$sum
.$sort
to sort the results by total sales in descending order.
Here's the code for this pipeline:
db.sales.aggregate([ { $match: { date: { $gte: ISODate("2021-01-01"), $lt: ISODate("2022-01-01") } } }, { $group: { _id: "$product_id", total_sales: { $sum: { $multiply: ["$quantity", "$price"] } } } }, { $sort: { total_sales: -1 } } ]);
This example demonstrates how you can use MongoDB's aggregation framework to perform complex data processing tasks with just a few lines of code. By chaining aggregation operations in a pipeline, you can efficiently transform and analyze your data to meet your specific needs.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Supporting All Operations (psst, it's free!).
FAQ
What are MongoDB aggregation operations?
MongoDB aggregation operations are powerful tools that allow you to process and transform data stored in your MongoDB collections. These operations can perform complex tasks like data filtering, analysis, and summarization, making it easier for you to extract insights and valuable information from your stored data.
How can I create an aggregation pipeline in MongoDB?
An aggregation pipeline in MongoDB is a series of stages that transform and process the data as it passes through the pipeline. To create an aggregation pipeline, you can use the aggregate()
function on a collection and provide an array of stage operators. Here's an example in JavaScript:
db.collection("orders").aggregate([ { $match: { status: "completed" } }, { $group: { _id: "$customerID", total: { $sum: "$amount" } } }, { $sort: { total: -1 } } ])
This pipeline first filters the "orders" collection with the $match
stage to include only completed orders, then groups the orders by customerID and calculates the total amount per customer using the $group
stage, and finally sorts the results by the total amount in descending order using the $sort
stage.
What are some common aggregation operations in MongoDB?
Some common MongoDB aggregation operations include:
$match
: Filters the data according to specified conditions.$group
: Groups data by a specified identifier and allows aggregation over the grouped data.$sort
: Sorts the data by specified fields in ascending or descending order.$limit
: Limits the number of documents passed to the next stage.$project
: Selects or reshapes the fields included in the result documents. These are just a few examples, and there are many more powerful aggregation operations available in MongoDB.
Can I use aggregation operations to join data from multiple collections in MongoDB?
Yes, you can use the $lookup
aggregation operation to join data from multiple collections in MongoDB. The $lookup
stage allows you to perform a left outer join on another collection and combine the data based on a specified condition. Here's an example:
db.collection("orders").aggregate([ { $match: { status: "completed" } }, { $lookup: { from: "customers", localField: "customerID", foreignField: "_id", as: "customerDetails" } } ])
In this example, we first filter the "orders" collection to include only completed orders, and then use the $lookup
stage to join the "orders" collection with the "customers" collection, matching the "customerID" field in the "orders" collection with the "_id" field in the "customers" collection. The resulting joined data will be stored in a new array field called "customerDetails" in the output documents.