MongoDB Indexing
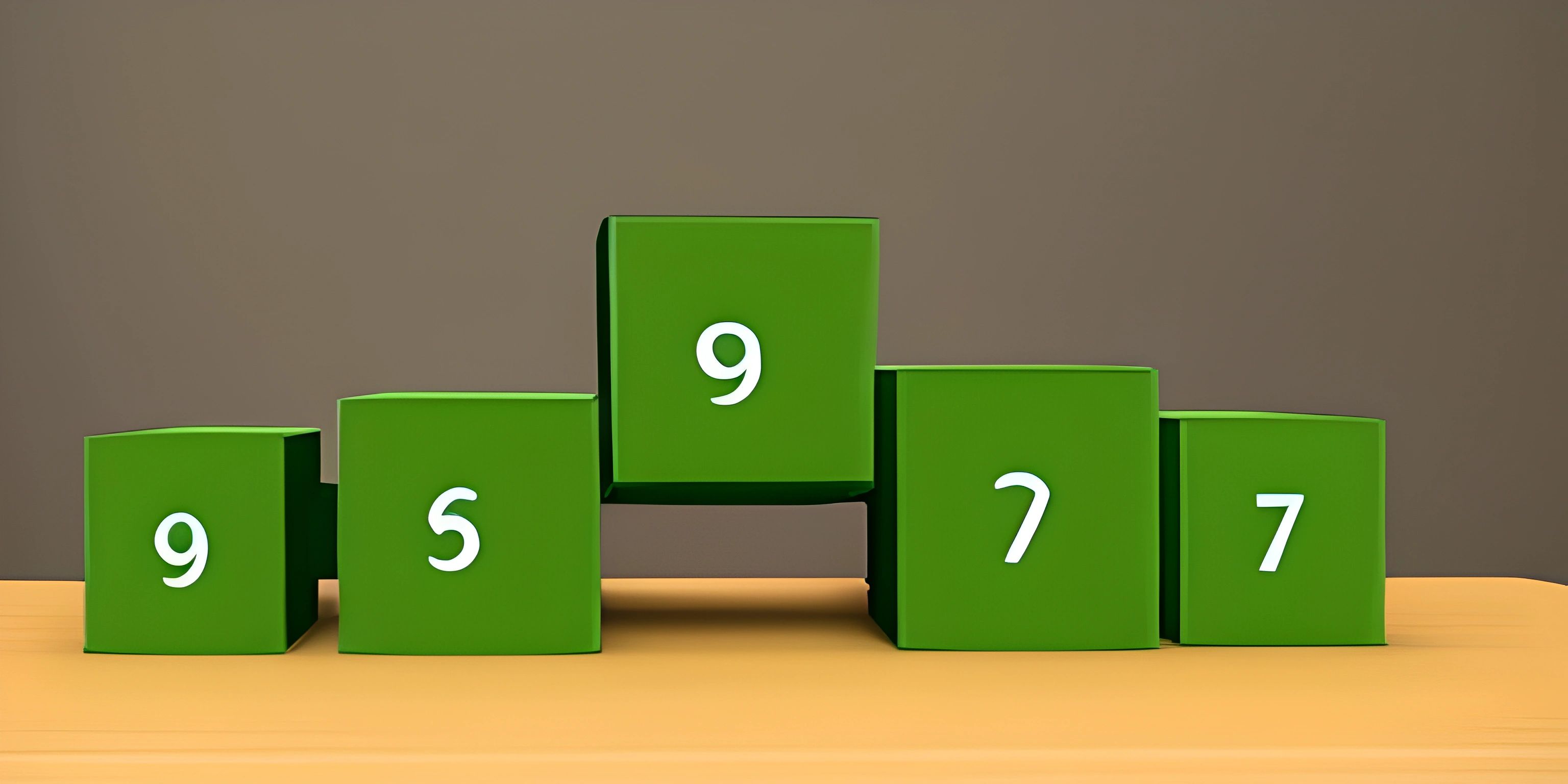
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Indexing is a powerful mechanism in MongoDB that helps speed up database queries. By using indexes, you can drastically improve the performance of your application, ensuring that your end-users are satisfied with quick and efficient data retrieval.
The Concept of Indexing
Imagine you have a large book, and you're looking for information on a specific topic. Without an index, you would have to go through the entire book page by page until you find what you're looking for. This would be both time-consuming and inefficient. But if the book has an index at the end, you can quickly locate the pages containing your desired information.
The same concept applies to databases. When a query is executed in MongoDB, the database searches through the documents in a collection to find the matching ones. Without an index, MongoDB performs a collection scan, going through every document until it finds the required data. This can be slow, especially when dealing with large collections.
An index in MongoDB is a data structure that stores a specific field or set of fields from documents in a collection, ordered by the value of those fields. An indexed field allows MongoDB to efficiently search for documents without scanning the entire collection.
Creating an Index
Creating an index in MongoDB is simple. You can use the createIndex
method on a collection to create an index on one or more fields. Here's an example using the MongoDB shell:
db.users.createIndex({ "name": 1 });
In this example, we're creating an index on the name
field of the users
collection. The 1
value indicates that the index should be sorted in ascending order. If you want to create an index in descending order, use -1
instead.
You can also create a compound index on multiple fields. This example creates a compound index on the name
and email
fields:
db.users.createIndex({ "name": 1, "email": 1 });
Index Types
MongoDB provides several types of indexes to cater to different query patterns:
-
Single Field Index: As the name suggests, this index is created on a single field of the documents in a collection. It supports queries on that specific field.
-
Compound Index: This index is created on multiple fields within a document. It can support queries on the indexed fields as well as their combinations.
-
Multikey Index: This index is used for fields that contain arrays. MongoDB automatically creates an index for each element of the array.
-
Text Index: A text index is designed specifically for searching text within string fields. It enables you to perform full-text searches and supports multiple language searches.
-
Geospatial Index: This index is used for geospatial data, such as points, lines, or polygons. It enables you to perform geospatial queries and analyze geographical data.
Removing an Index
If you no longer need an index or want to recreate it with different options, you can remove it using the dropIndex
method:
db.users.dropIndex("name_1");
In this example, we're removing the index on the name
field from the users
collection. The argument "name_1"
is the index name generated by MongoDB when the index was created.
Conclusion
Indexing is a critical aspect of optimizing your MongoDB database for performance. By creating the right indexes, you can significantly improve query speed and reduce the load on your database. Remember to carefully plan and maintain your indexes to ensure that your application delivers fast and efficient data retrieval.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Full-stack Web Frameworks (Next.js) (psst, it's free!).
FAQ
What is indexing in MongoDB and why is it important?
Indexing in MongoDB is a technique that provides an efficient way to search and store data in the database. It improves the performance of database operations by allowing the database engine to quickly locate and retrieve documents based on specified fields. Without indexing, MongoDB would have to perform a collection scan, which can be time-consuming for large datasets. By implementing proper indexing, it significantly speeds up database queries and enhances overall performance.
How do I create an index in MongoDB?
To create an index in MongoDB, use the createIndex()
method on a specific collection. The method takes an object as its argument, where the keys represent the fields to be indexed, and the values define the index type. For example, to create an ascending index on the "username" field:
db.collection("users").createIndex({ "username": 1 });
This command will create an ascending index on the "username" field in the "users" collection.
What are the different types of indexes in MongoDB?
MongoDB supports various types of indexes, including:
- Single Field Index: An index on a single field of the documents in a collection.
- Compound Index: An index on multiple fields in a collection. The order of fields in the index definition matters.
- Multikey Index: An index on fields that hold arrays. Multiple index entries are created for each key in the array.
- Text Index: An index for searching text content within documents. Supports full-text search and linguistic features such as stopwords, stemming, and tokenization.
- Geospatial Index: An index for querying geospatial data, such as points, lines, and polygons on a 2D plane or a sphere.
How do I drop or remove an index in MongoDB?
To drop an index in MongoDB, use the dropIndex()
method on the target collection. The method takes the name of the index you want to remove. For example, to drop an index named "username_1" in the "users" collection:
db.collection("users").dropIndex("username_1");
How can I check the existing indexes in a MongoDB collection?
To view the existing indexes in a MongoDB collection, use the getIndexes()
method on the target collection. For example, to check the indexes on the "users" collection:
db.collection("users").getIndexes();
This command will return an array of index objects, including their names, keys, and other properties.