Next.js Static Site Generation
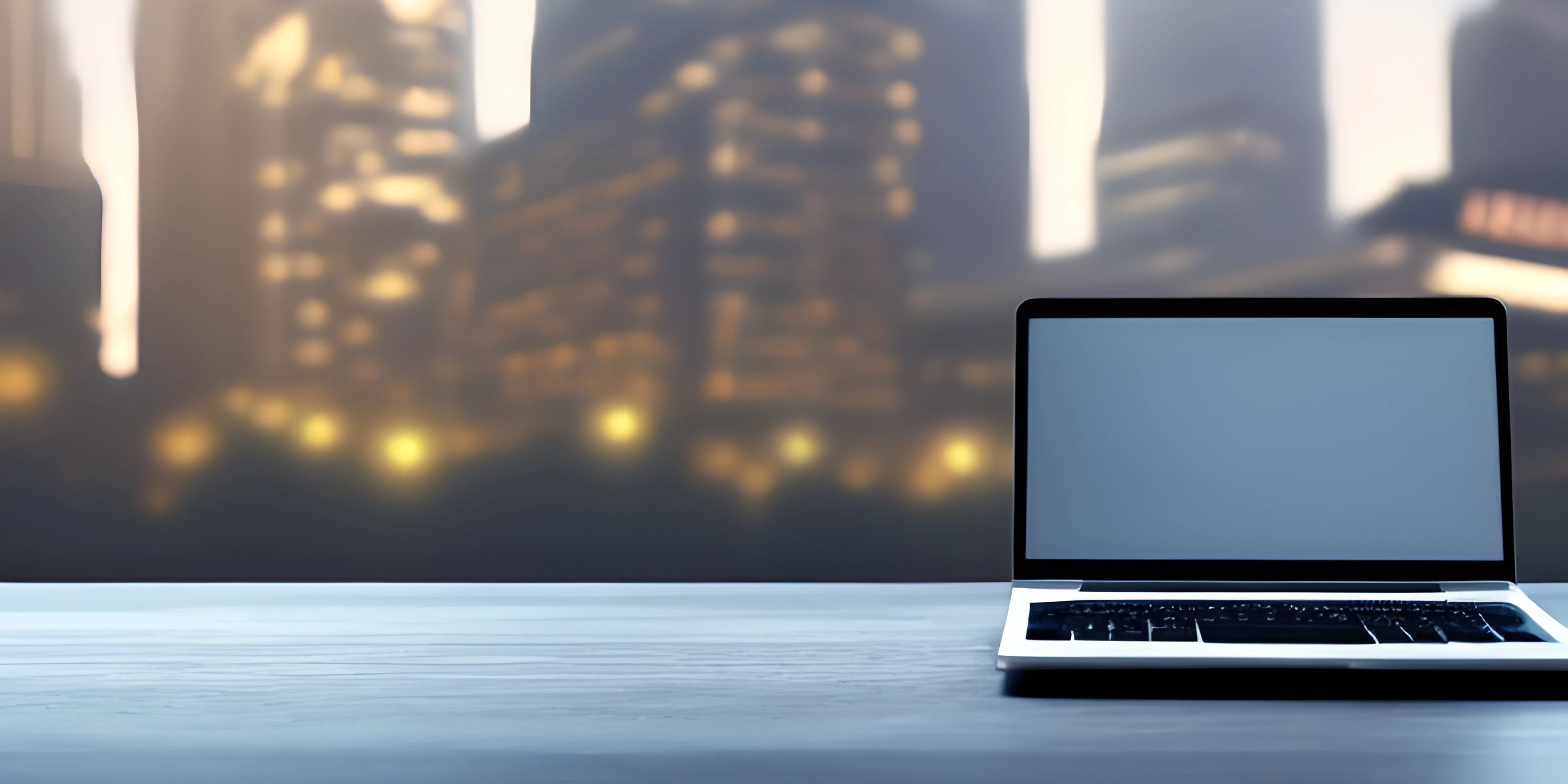
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Next.js, a popular React framework, has revolutionized the way we build modern web applications. One of its powerful features is Static Site Generation (SSG), which allows us to pre-render pages at build time, improving performance and providing a delightful user experience. So let's dive into the world of Next.js and explore its SSG capabilities!
What is Static Site Generation (SSG)?
Static Site Generation refers to the process of generating static HTML, CSS, and JavaScript files at build time, rather than sending requests to a server to fetch or generate content at runtime. SSG has gained popularity, as it provides fast load times, better SEO, and improved security.
How does Next.js implement SSG?
Next.js provides built-in support for SSG through two primary functions: getStaticProps
and getStaticPaths
. These functions enable developers to fetch data at build time and generate static pages based on that data.
getStaticProps
getStaticProps
is an asynchronous function that fetches the data required to pre-render a page. This function runs only on the server-side during build time, and its result is passed as props to the page component.
Here's a simple example:
// pages/index.js import { useState } from "react"; export default function Home({ posts }) { const [postList, setPostList] = useState(posts); return ( <div> {postList.map((post) => ( <div key={post.id}>{post.title}</div> ))} </div> ); } export async function getStaticProps() { const res = await fetch("https://jsonplaceholder.typicode.com/posts"); const posts = await res.json(); return { props: { posts }, }; }
In this example, getStaticProps
fetches a list of posts at build time, which is then passed as props to the Home
component.
getStaticPaths
getStaticPaths
is used for dynamic pages, where the page path depends on external data. This function defines a list of paths that should be pre-rendered as static pages. It is essential when using SSG with dynamic routes, such as /posts/[id]
.
Here's an example:
// pages/posts/[id].js import { useRouter } from "next/router"; export default function Post({ post }) { const router = useRouter(); if (router.isFallback) { return <div>Loading...</div>; } return <div>{post.title}</div>; } export async function getStaticPaths() { const res = await fetch("https://jsonplaceholder.typicode.com/posts"); const posts = await res.json(); const paths = posts.map((post) => `/posts/${post.id}`); return { paths, fallback: true }; } export async function getStaticProps({ params }) { const res = await fetch( `https://jsonplaceholder.typicode.com/posts/${params.id}` ); const post = await res.json(); return { props: { post } }; }
In this example, getStaticPaths
fetches the list of posts and generates paths for each post. Then, getStaticProps
fetches the data for a specific post based on the id
parameter.
Benefits of using SSG with Next.js
Utilizing Next.js's SSG capabilities offers several advantages, including:
- Improved performance: Static pages are served from a CDN, resulting in faster load times and a better user experience.
- Better SEO: Pre-rendered pages ensure that all content is available to search engines, improving visibility and rankings.
- Increased security: Since there's no direct connection to a database or server, the attack surface is significantly reduced.
- Simplified deployment: Generated static files can be easily deployed to various hosting providers, such as Vercel, Netlify, or GitHub Pages.
In conclusion, Next.js's Static Site Generation feature is a powerful tool for building modern web applications. It offers improved performance, better SEO, enhanced security, and simplified deployment, making it an excellent choice for developers looking to create static sites and web applications.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Full-stack Web Frameworks (Next.js) (psst, it's free!).
FAQ
What is Next.js Static Site Generation (SSG)?
Next.js Static Site Generation (SSG) is a feature offered by the popular React framework, Next.js, that allows developers to generate static HTML pages at build time. This provides improved performance and better SEO benefits, as the pre-rendered pages can be served directly by a CDN without the need for server-side rendering.
How does Next.js SSG work?
Next.js SSG works by pre-rendering your React components into static HTML files during the build process. These files are then served by a CDN, which delivers the content quickly and efficiently to users. To enable SSG in your Next.js application, you need to use the getStaticProps
function in your page components. This function is responsible for fetching the necessary data and returning it as props to your component. Here's an example:
import React from "react"; export async function getStaticProps() { const data = await fetchData(); // Your data fetching logic here return { props: { data, }, }; } const MyPage = ({ data }) => { return ( <div> {/* Render your data here */} </div> ); }; export default MyPage;
What are the benefits of using Next.js SSG?
Some of the key benefits of using Next.js SSG include:
- Improved performance: Static files are served directly by a CDN, which reduces server load and provides faster load times for users.
- Better SEO: Pre-rendered pages can be easily crawled and indexed by search engines.
- Scalability: Since the static pages are generated at build time, your application can easily scale to handle a large number of users.
- Reduced server costs: With SSG, there's no need for server-side rendering, which can result in lower server costs.
How can I enable incremental static regeneration in Next.js?
Incremental Static Regeneration (ISR) is a powerful feature in Next.js that allows you to update static pages after they've been generated, without having to rebuild the entire application. To enable ISR, you simply need to add a revalidate
property to the object returned by getStaticProps
. The revalidate
property specifies how often (in seconds) the page should be regenerated. Here's an example:
export async function getStaticProps() { const data = await fetchData(); return { props: { data, }, revalidate: 60, // Regenerate the page every 60 seconds }; }
Can I use Next.js SSG with dynamic routes?
Yes, you can use Next.js SSG with dynamic routes. To do so, you'll need to implement the getStaticPaths
function in your page component, which is responsible for specifying the dynamic routes that should be pre-rendered. Here's an example:
export async function getStaticPaths() { const paths = await fetchPaths(); // Your logic for fetching the paths return { paths, fallback: "blocking", // "blocking" ensures that new paths are generated on-demand with SSG }; }