Polymorphism in C++
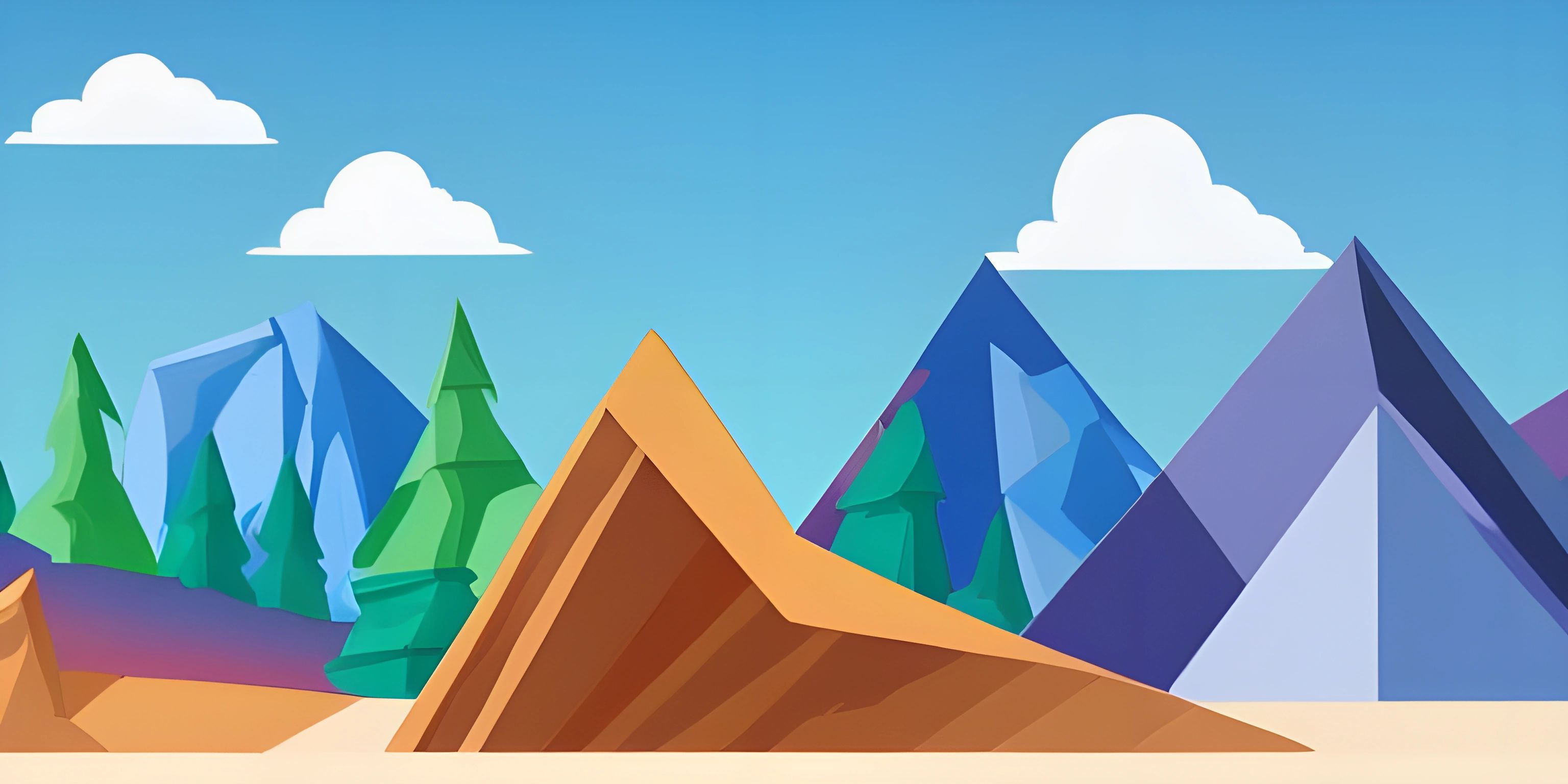
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Polymorphism is a powerful concept in object-oriented programming languages like C++. It allows objects of different classes to be treated as objects of a common superclass. This leads to a more flexible and reusable code. In this article, we'll dive into the world of polymorphism in C++, exploring its types and their implementations.
Static Polymorphism
Static polymorphism, also known as compile-time polymorphism, is when the function to be called is determined during the compilation of the program. C++ offers two ways to achieve static polymorphism: function overloading and operator overloading.
Function Overloading
Function overloading is the process of defining multiple functions with the same name but different parameters. The compiler selects the appropriate function based on the number and types of arguments during the compilation. Here's an example:
#include <iostream> void printArea(int length, int width) { std::cout << "Area of rectangle: " << length * width << std::endl; } void printArea(double radius) { std::cout << "Area of circle: " << 3.14159 * radius * radius << std::endl; } int main() { printArea(5, 3); // Calls rectangle area function printArea(7.0); // Calls circle area function return 0; }
Operator Overloading
Operator overloading allows you to redefine the behavior of existing operators for custom classes. This enhances code readability and makes it more intuitive. Here's an example using a Time
class:
#include <iostream> class Time { public: int hours, minutes; Time(int h, int m) { hours = h; minutes = m; } Time operator+(const Time& other) { int totalMinutes = minutes + other.minutes; int totalHours = hours + other.hours + (totalMinutes / 60); totalMinutes %= 60; return Time(totalHours, totalMinutes); } }; int main() { Time t1(2, 30); Time t2(1, 50); Time t3 = t1 + t2; std::cout << "Total time: " << t3.hours << " hours, " << t3.minutes << " minutes" << std::endl; return 0; }
Dynamic Polymorphism
Dynamic polymorphism, or runtime polymorphism, is when the function to be called is determined during the program's execution. C++ provides two ways to achieve dynamic polymorphism: virtual functions and function overriding.
Virtual Functions
Virtual functions are member functions of a base class that can be overridden in a derived class. When a virtual function is called on a base class pointer, the appropriate derived class's function is called. Here's an example with a Shape
class and two derived classes, Circle
and Rectangle
:
#include <iostream> class Shape { public: virtual double area() = 0; // Pure virtual function }; class Circle : public Shape { public: double radius; Circle(double r) : radius(r) {} double area() override { return 3.14159 * radius * radius; } }; class Rectangle : public Shape { public: double length, width; Rectangle(double l, double w) : length(l), width(w) {} double area() override { return length * width; } }; int main() { Shape* shape = new Circle(5.0); std::cout << "Area of circle: " << shape->area() << std::endl; shape = new Rectangle(4.0, 3.0); std::cout << "Area of rectangle: " << shape->area() << std::endl; return 0; }
Function Overriding
Function overriding is when a derived class provides its implementation of a base class function. This method allows you to achieve dynamic polymorphism using virtual functions. The example in the "Virtual Functions" section demonstrates function overriding as well.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is polymorphism in C++?
Polymorphism in C++ is a concept that allows objects of different classes to be treated as objects of a common superclass. It makes code more flexible and reusable. Polymorphism can be achieved at compile-time (static) or runtime (dynamic).
What are the types of polymorphism in C++?
The two types of polymorphism in C++ are static polymorphism (compile-time polymorphism) and dynamic polymorphism (runtime polymorphism). Static polymorphism is achieved using function overloading and operator overloading, while dynamic polymorphism is achieved using virtual functions and function overriding.
How does function overloading work in C++?
Function overloading in C++ is the process of defining multiple functions with the same name but different parameters. The compiler selects the appropriate function based on the number and types of arguments during the compilation.
What is the purpose of virtual functions in C++?
Virtual functions in C++ are member functions of a base class that can be overridden in a derived class. They allow for dynamic polymorphism, where the appropriate derived class's function is called when a virtual function is called on a base class pointer.