Designing and Building RESTful APIs with Node.js and Express
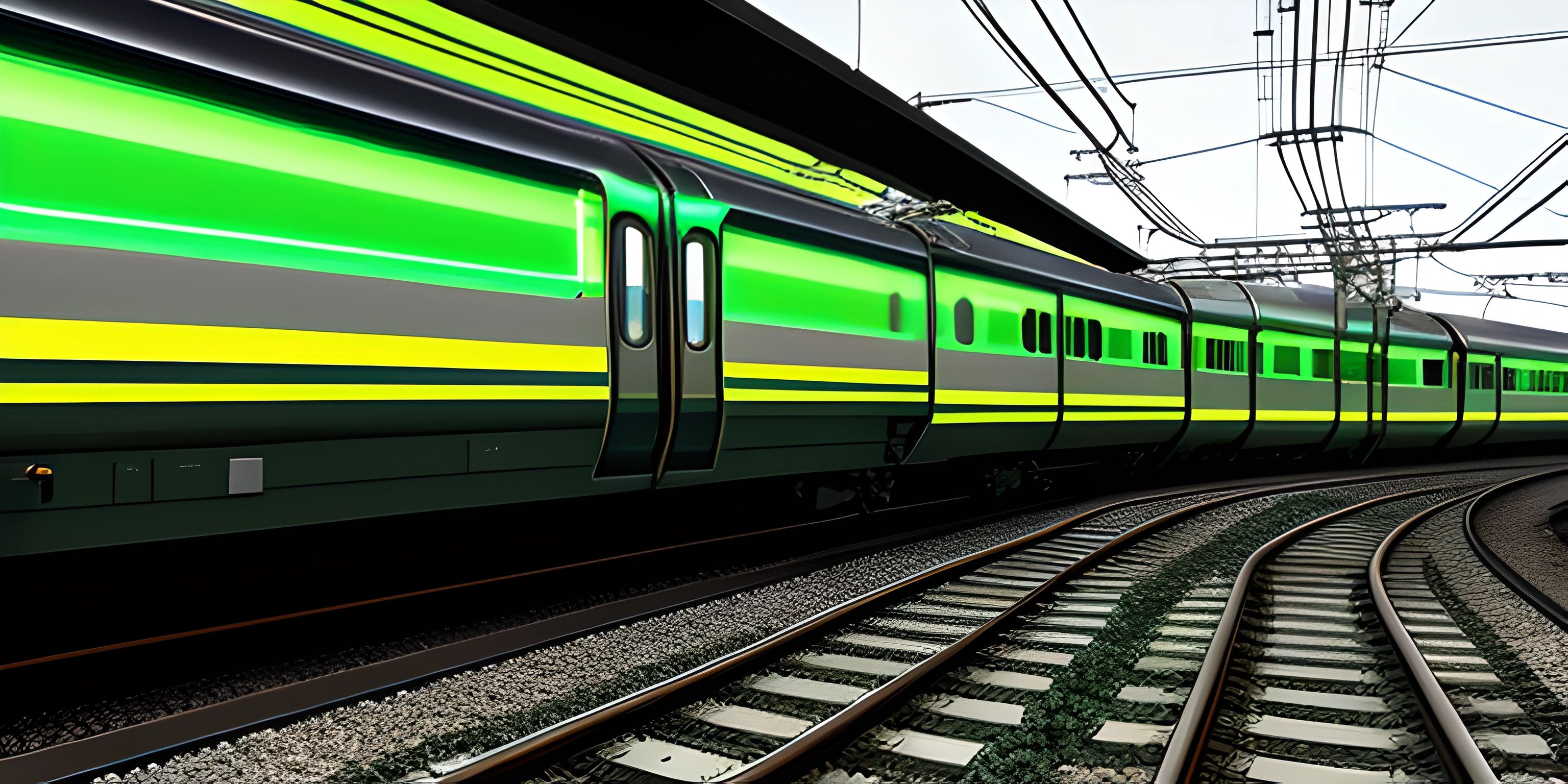
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
In this article, we will learn how to design and build a RESTful API using Node.js and Express. We will go through the process step by step, from setting up the environment to creating routes and testing our API.
Prerequisites
Before we begin, make sure you have the following installed on your system:
- Node.js
- npm (Node Package Manager)
Setting up the project
First, create a new directory for your project and navigate to it:
mkdir nodejs-restful-api cd nodejs-restful-api
Next, initialize a new Node.js project by running:
npm init -y
This will create a package.json
file in your project directory.
Installing Express
Express is a minimal and flexible Node.js web application framework that makes it easier to build web applications and APIs. To install Express, run:
npm install express
Creating the server
Create a new file called app.js
in your project directory and add the following code:
const express = require("express"); const app = express(); const port = 3000; app.get("/", (req, res) => { res.send("Welcome to our RESTful API!"); }); app.listen(port, () => { console.log(`Server is running on port ${port}`); });
In this code, we import the Express module, create an Express application, and define a simple route that returns a welcome message. We then start the server on port 3000.
Defining routes
Now, let's create routes for a simple CRUD (Create, Read, Update, Delete) API. We will use the following routes for this example:
GET /items
: Retrieve all itemsGET /items/:id
: Retrieve a specific item by IDPOST /items
: Create a new itemPUT /items/:id
: Update an existing item by IDDELETE /items/:id
: Delete an item by ID
Add the following code to app.js
:
app.use(express.json()); app.get("/items", (req, res) => { res.send("Retrieve all items"); }); app.get("/items/:id", (req, res) => { res.send(`Retrieve item with ID: ${req.params.id}`); }); app.post("/items", (req, res) => { res.send("Create a new item"); }); app.put("/items/:id", (req, res) => { res.send(`Update item with ID: ${req.params.id}`); }); app.delete("/items/:id", (req, res) => { res.send(`Delete item with ID: ${req.params.id}`); });
We define the routes using the app.get()
, app.post()
, app.put()
, and app.delete()
methods, which correspond to the HTTP methods GET, POST, PUT, and DELETE.
Testing the API
To test the API, you can use tools like Postman or Insomnia. Alternatively, you can use curl
from the command line.
Start your server by running:
node app.js
Then, in a separate terminal window, test the different routes:
curl http://localhost:3000/items curl http://localhost:3000/items/1 curl -X POST http://localhost:3000/items curl -X PUT http://localhost:3000/items/1 curl -X DELETE http://localhost:3000/items/1
You should see the corresponding messages for each route.
Conclusion
You have now learned how to design and build a simple RESTful API using Node.js and Express. You can further extend this API by adding data storage, validation, and authentication. Happy coding!
FAQ
What is a RESTful API and why is it important in web development?
A RESTful API (Representational State Transfer) is a set of conventions and principles for designing networked APIs. It enables communication between clients and servers using a stateless, cacheable, and client-server architecture. RESTful APIs are important as they provide a standardized, scalable, and maintainable way to build web services, making it easier for developers to create and consume APIs.
How do I get started with Node.js and Express to build a RESTful API?
To get started, you'll need to have Node.js installed on your system. Then, follow these steps:
- Create a new project directory and navigate to it.
- Run
npm init
to initialize a new Node.js project. - Install Express using
npm install express
. - Create an
app.js
file and set up a basic Express server. - Start building your RESTful API by defining routes and HTTP methods (GET, POST, PUT, DELETE) for each resource.
How can I structure my Node.js and Express project for better organization and maintainability?
Organizing your project using the Model-View-Controller (MVC) pattern is a great practice. Here's a simple structure:
models/
: Contains your data models.controllers/
: Houses your business logic and handles communication between the models and views.views/
: Contains your templates (if using a templating engine) or static files.routes/
: Defines your API routes and associates them with their respective controllers.app.js
: Configures your Express app and sets up middleware, routes, and other application-level settings.
How can I ensure my RESTful API is secure and handles errors properly?
To improve security and error handling in your Node.js and Express RESTful API, consider the following:
- Use HTTPS to encrypt data in transit.
- Implement authentication and authorization (e.g., JSON Web Tokens or OAuth).
- Validate user input to prevent security vulnerabilities and data corruption.
- Use middleware (e.g.,
express-validator
) to check and sanitize user input. - Set up proper error handling using Express error handling middleware.
- Limit the rate at which requests can be made to your API to prevent abuse or denial of service attacks.
How can I test and debug my RESTful API built with Node.js and Express?
To test and debug your API, you can:
- Use tools like Postman or Insomnia to send requests and inspect responses.
- Write automated tests using testing frameworks and libraries like Mocha, Chai, and Supertest.
- Use
console.log()
statements, or better yet, a logging library like Winston or Morgan, to log information to help you identify issues. - Utilize the built-in Node.js debugger or a third-party tool like Visual Studio Code's debugger for more advanced debugging.