Working with Files in NodeJS
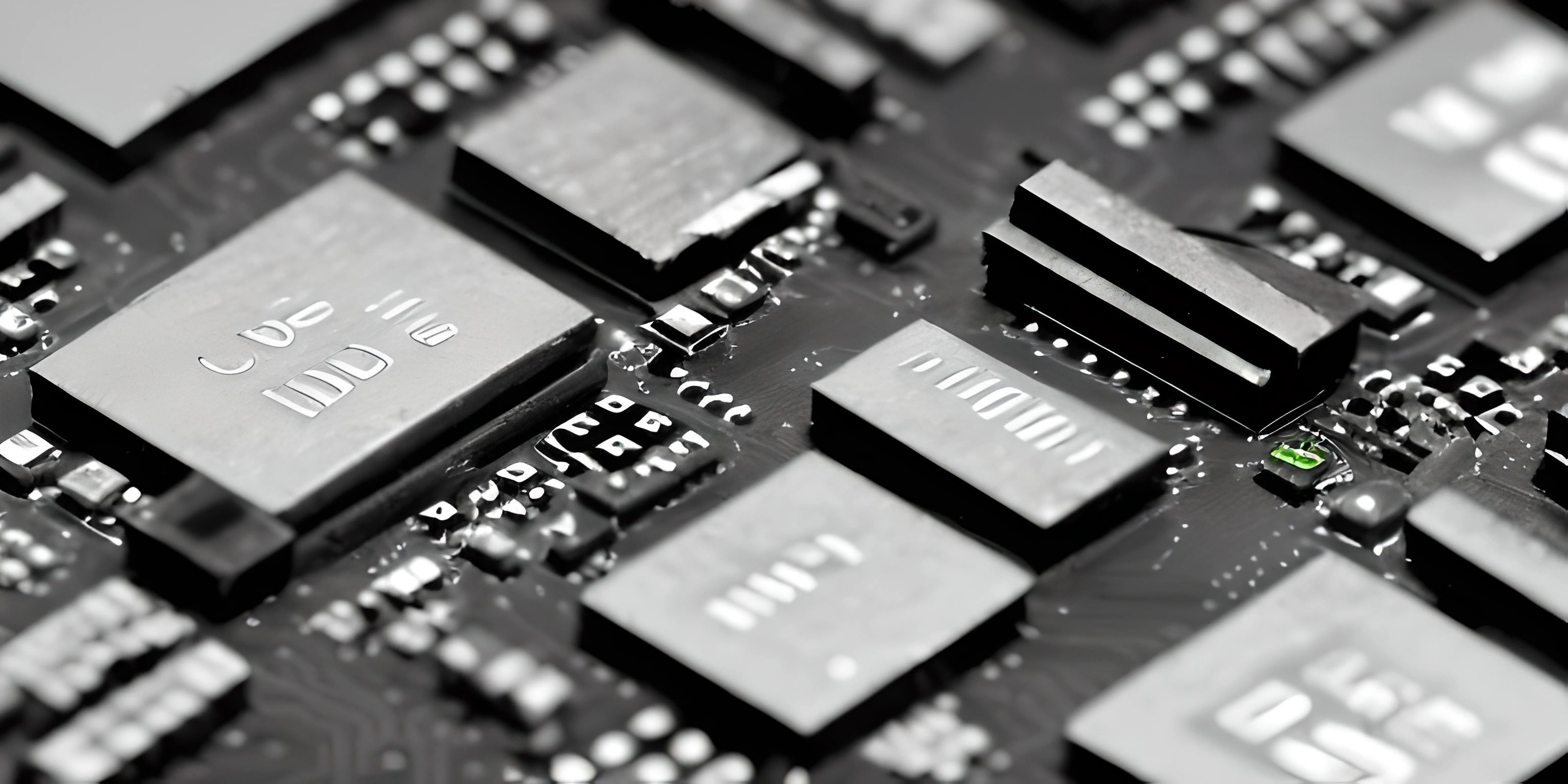
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
In NodeJS, the File System (FS) module provides an API to interact with the file system on your computer. This article will cover the basics of working with the FS module, including reading, writing, and manipulating files.
Importing the FS Module
To use the FS module, you'll need to import it into your Node.js script. You can do this using the require
function:
const fs = require("fs");
Reading Files
To read a file, you can use the fs.readFile
or fs.readFileSync
functions. The former is asynchronous, while the latter is synchronous.
Asynchronous File Reading
fs.readFile("example.txt", "utf8", (err, data) => { if (err) { console.error("Error reading file:", err); } else { console.log("File content:", data); } });
Synchronous File Reading
try { const data = fs.readFileSync("example.txt", "utf8"); console.log("File content:", data); } catch (err) { console.error("Error reading file:", err); }
Writing Files
To write to a file, you can use the fs.writeFile
or fs.writeFileSync
functions. The former is asynchronous, while the latter is synchronous.
Asynchronous File Writing
const content = "This is a new text."; fs.writeFile("example.txt", content, (err) => { if (err) { console.error("Error writing file:", err); } else { console.log("File written successfully."); } });
Synchronous File Writing
const content = "This is a new text."; try { fs.writeFileSync("example.txt", content); console.log("File written successfully."); } catch (err) { console.error("Error writing file:", err); }
Manipulating Files
The FS module also provides functions for manipulating files, like renaming and deleting them.
Renaming Files
fs.rename("oldName.txt", "newName.txt", (err) => { if (err) { console.error("Error renaming file:", err); } else { console.log("File renamed successfully."); } });
Deleting Files
fs.unlink("example.txt", (err) => { if (err) { console.error("Error deleting file:", err); } else { console.log("File deleted successfully."); } });
These are just the basics of working with the File System module in NodeJS. For more advanced use cases, you can refer to the official Node.js documentation.
FAQ
How do I import the File System module in NodeJS?
To import the File System module in NodeJS, you can use the require()
function. Here's an example:
const fs = require("fs");
How do I read a file using the File System module in NodeJS?
To read a file in NodeJS using the File System module, you can use the fs.readFile()
function. Here's an example:
fs.readFile("example.txt", "utf8", (err, data) => { if (err) throw err; console.log(data); });
How do I write to a file using the File System module in NodeJS?
To write to a file in NodeJS using the File System module, you can use the fs.writeFile()
function. Here's an example:
const data = "Hello, World!"; fs.writeFile("example.txt", data, (err) => { if (err) throw err; console.log("The file has been saved!"); });
How do I delete a file using the File System module in NodeJS?
To delete a file in NodeJS using the File System module, you can use the fs.unlink()
function. Here's an example:
fs.unlink("example.txt", (err) => { if (err) throw err; console.log("The file has been deleted!"); });
How can I check if a file exists using the File System module in NodeJS?
To check if a file exists in NodeJS using the File System module, you can use the fs.existsSync()
function. Here's an example:
const fileExists = fs.existsSync("example.txt"); console.log(fileExists ? "The file exists" : "The file does not exist");