Creating Interactive Visuals with p5.js
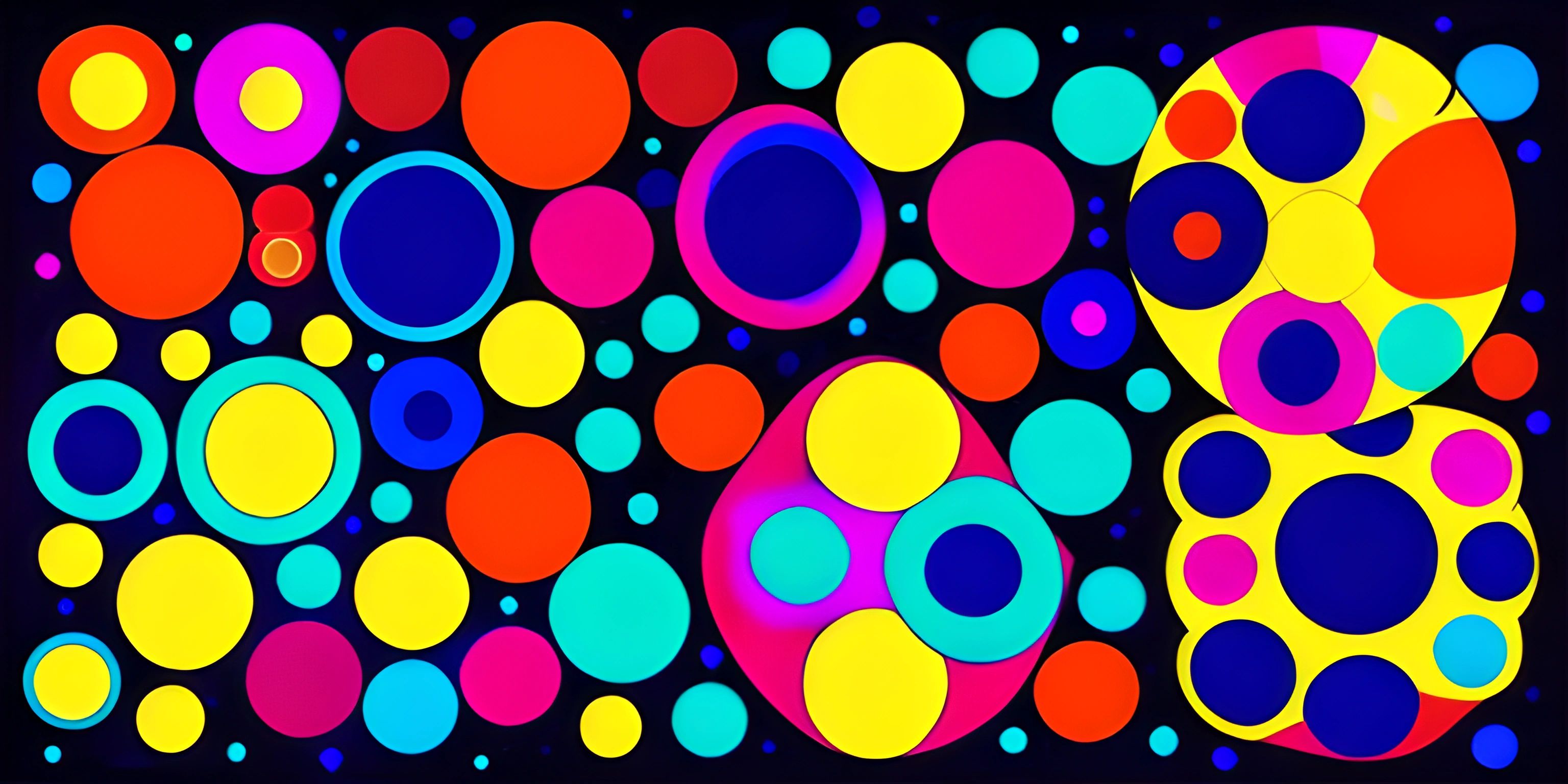
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Ready to create some eye-catching visuals and interactive animations with ease? Look no further than p5.js, a powerful and easy-to-use JavaScript library designed to help you create graphics, animations, and interactive applications in a snap. It was developed by the Processing Foundation and is a great tool for artists, designers, and programmers alike.
Getting Started with p5.js
To start using p5.js, you'll first need to include the library in your HTML file. You have two options: link to the online version of the library or download it and link to the local version. Here's an example of how to link to the online version:
<!DOCTYPE html> <html> <head> <script src="https://cdnjs.cloudflare.com/ajax/libs/p5.js/1.4.0/p5.js"></script> </head> <body> </body> </html>
With the library included, you can now create your first p5.js sketch.
Your First p5.js Sketch
A p5.js sketch is a JavaScript file that uses the p5.js library to create graphics and animations. The basic structure of a sketch includes two essential functions: setup()
and draw()
.
setup()
is called once at the beginning of your sketch, and it's the perfect place to initialize your canvas, set up any variables, or load assets. draw()
is called continuously after setup()
and is where you'll create your animations and visuals.
Here's a simple example sketch that creates a canvas and draws a moving circle:
function setup() { createCanvas(600, 400); } function draw() { background(200); ellipse(mouseX, mouseY, 50, 50); }
In this example, createCanvas(600, 400)
initializes a canvas of width 600 pixels and height 400 pixels. background(200)
sets the background color to a light gray, and ellipse(mouseX, mouseY, 50, 50)
draws a circle at the mouse position with a diameter of 50 pixels.
Interactivity with p5.js
One of the main strengths of p5.js is its ability to handle user input and create interactive applications. Here's a simple example that changes the color of the background based on the position of the mouse:
let bgColor; function setup() { createCanvas(600, 400); bgColor = color(200); } function draw() { background(bgColor); ellipse(mouseX, mouseY, 50, 50); } function mousePressed() { bgColor = color(random(255), random(255), random(255)); }
In this example, we've introduced the mousePressed()
function, which is called whenever the mouse is pressed. Inside mousePressed()
, we change the value of bgColor
to a random color using the color()
and random()
functions.
With just a few lines of code, you've created an interactive sketch that responds to user input!
Next Steps
There's so much more you can do with p5.js, from creating complex animations to visualizing data. To dive deeper, check out the p5.js official reference and examples to get inspired and learn about additional functionality.
So go ahead, unleash your creativity, and start exploring the world of interactive visuals with p5.js!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Taking User Input (psst, it's free!).
FAQ
What is p5.js, and why should I use it for creating interactive visuals?
p5.js is a JavaScript library that aims to make coding interactive graphics easy and accessible for everyone. It is inspired by the well-known Processing programming language and simplifies the process of creating visuals and animations in web browsers. Some benefits of using p5.js include:
- Simple and easy-to-understand syntax
- A large and active community of users and contributors
- An extensive collection of built-in functions for creating visuals and animations
- The ability to integrate easily with other JavaScript libraries and technologies
How do I set up p5.js in my project?
To begin using p5.js in your project, you can follow these steps:
- Download the p5.js library from the official website (https://p5js.org/download/).
- Include the p5.js file in your HTML file using a script tag:
<script src="path/to/p5.js"></script>
- Create a JavaScript file for your p5.js sketch and link it to your HTML file:
<script src="path/to/your/sketch.js"></script>
- In your sketch.js file, start by declaring the
setup
anddraw
functions, which are the core building blocks of a p5.js sketch:
function setup() { createCanvas(400, 400); } function draw() { background(255); }
How can I create simple shapes and animations with p5.js?
p5.js provides a variety of built-in functions to create shapes, set colors, and control animations. Here are some examples:
- Creating a circle with
ellipse(x, y, width, height)
:
ellipse(200, 200, 50, 50);
- Setting the fill color with
fill(red, green, blue)
:
fill(255, 0, 0);
- Animating a shape by updating its position each frame:
let xPos = 0; function draw() { background(255); xPos += 1; ellipse(xPos, 200, 50, 50); }
How do I make my visuals interactive with p5.js?
p5.js allows you to easily add interactivity to your sketches using built-in functions for handling user input. Some examples include:
- Detecting mouse clicks with
mouseIsPressed
:
function draw() { background(255); if (mouseIsPressed) { fill(0); } else { fill(255); } ellipse(mouseX, mouseY, 50, 50); }
- Responding to keyboard input with
keyIsDown(keyCode)
:
function draw() { background(255); if (keyIsDown(LEFT_ARROW)) { xPos -= 5; } else if (keyIsDown(RIGHT_ARROW)) { xPos += 5; } ellipse(xPos, 200, 50, 50); }
Where can I find more resources and examples for learning p5.js?
The p5.js community has a wealth of resources for learning and getting inspired. Some popular resources include:
- The official p5.js website (https://p5js.org/) has a comprehensive reference, examples, and tutorials
- The Coding Train (https://www.youtube.com/user/shiffman) YouTube channel by Daniel Shiffman provides excellent video tutorials for beginners and advanced users
- OpenProcessing (https://www.openprocessing.org/) is a platform where users can share and explore p5.js sketches created by others in the community