Generative Art with p5.js
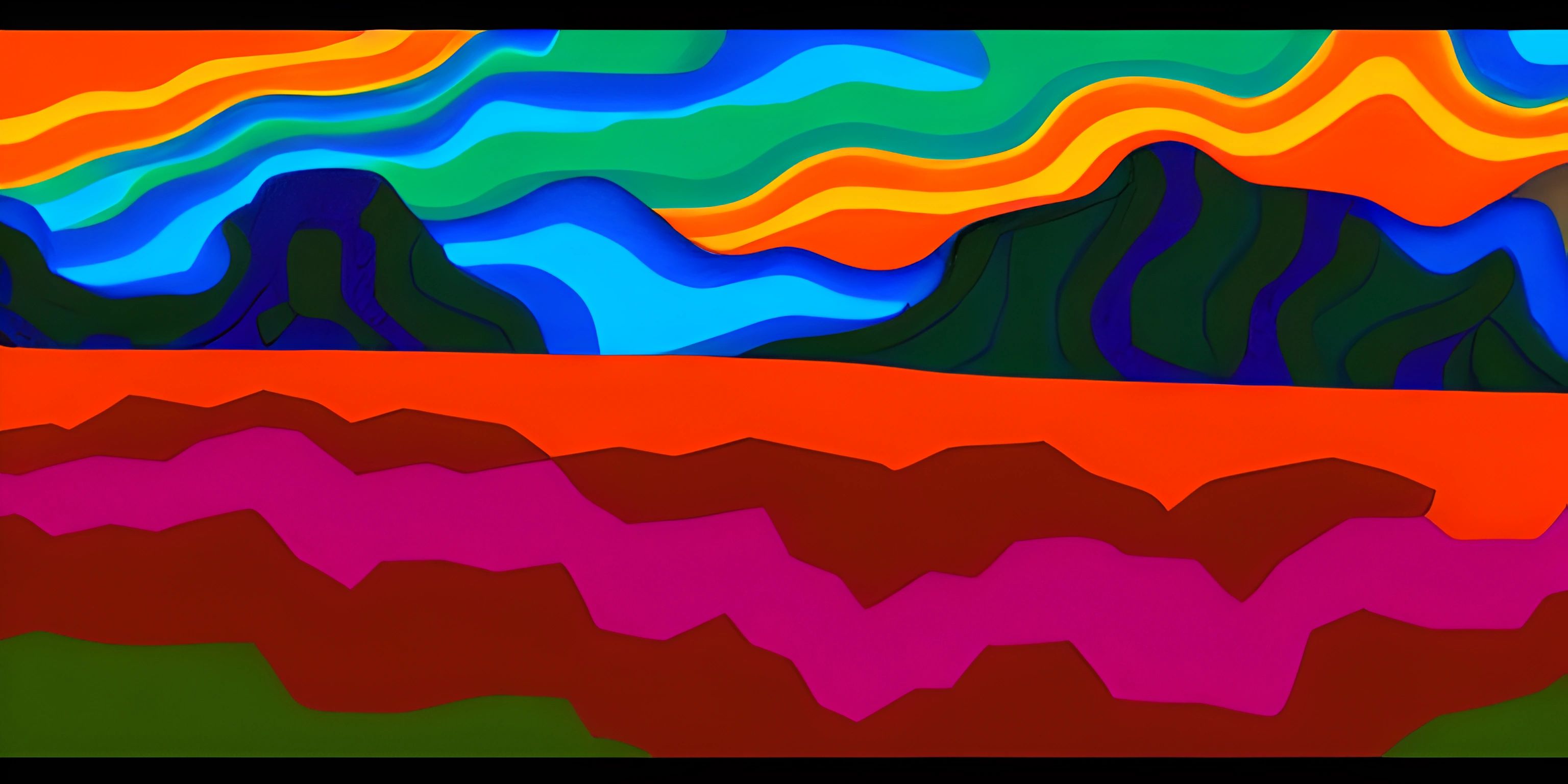
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Unleashing your creative side has never been easier, thanks to the magic of p5.js, a powerful JavaScript library that simplifies the process of creating interactive graphics, animations, and generative art in the browser. Get ready to dive into a new world of artistic expression!
Setting the Stage
Before we start painting our digital canvas with code, let's first set up our workspace. To create a new project with p5.js, you'll need to include the library in your HTML file:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <script src="https://cdnjs.cloudflare.com/ajax/libs/p5.js/1.4.0/p5.js"></script> <script src="sketch.js"></script> </head> <body> </body> </html>
Now that we have our HTML file ready, let's create a sketch.js
file, which will contain our p5.js code.
The p5.js Life Cycle
In p5.js, the script execution revolves around two main functions: setup()
and draw()
. The setup()
function runs once at the beginning of your program, while the draw()
function loops continuously, allowing for animations and real-time interactivity.
function setup() { createCanvas(800, 600); // Create an 800x600 canvas background(255); // Set a white background } function draw() { // Your generative art code will go here }
Creating Generative Art
Now that we have our canvas set up, let's start creating some generative art. For this example, we'll create a simple piece that uses circles with random colors and sizes.
function draw() { let x = random(width); // Random x-coordinate let y = random(height); // Random y-coordinate let size = random(10, 50); // Random size between 10 and 50 let color = [random(255), random(255), random(255)]; // Random RGB color fill(color); noStroke(); ellipse(x, y, size, size); }
With just a few lines of code, we've created an ever-changing piece of generative art. The draw()
function continually generates new circles with random positions, sizes, and colors, painting our canvas with an infinite variety of patterns.
Going Further
This is just the tip of the iceberg when it comes to generative art with p5.js. There are countless possibilities for creating intricate designs, interactive installations, and mesmerizing animations. To dive deeper into the world of creative coding, explore additional p5.js features and generative art techniques.
As you continue to develop your skills, remember to embrace the spirit of experimentation and playfulness that lies at the heart of generative art. Happy coding, and may your artistic journey be filled with endless inspiration!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Making Art with Code (psst, it's free!).
FAQ
What is p5.js and why is it useful for generative art?
p5.js is an open-source JavaScript library designed to make coding accessible for artists, designers, educators, and beginners. It simplifies the process of working with graphics and interactivity in web-based projects. p5.js is particularly useful for generative art because it provides an intuitive and friendly interface for creating visuals and animations, while still being powerful enough to create complex and dynamic pieces of art.
How do I get started with p5.js for generative art?
To get started with p5.js for generative art, follow these steps:
- Visit the p5.js website (https://p5js.org/) and download the latest version of the library.
- Create an HTML file and include the p5.js library in your project.
- Write a basic p5.js sketch using the
setup()
anddraw()
functions. - Start adding your own custom code to generate visuals and animations. You can also use the p5.js web editor (https://editor.p5js.org/) for a more streamlined experience, as it allows you to code and see the results directly in your browser without needing to set up a local development environment.
Can you provide a simple example of generative art using p5.js?
Sure! Here's a minimal example of generative art using p5.js:
function setup() { createCanvas(800, 600); background(0); stroke(255); noFill(); } function draw() { let x1 = random(width); let y1 = random(height); let x2 = random(width); let y2 = random(height); line(x1, y1, x2, y2); }
This code creates a canvas with a black background and then continuously draws random white lines on it. This is a very basic example, but you can build upon it and create more complex generative art pieces by adding colors, shapes, and interactivity.
Can I add interactivity to my generative art with p5.js?
Absolutely! p5.js provides a wide range of functions that enable you to add interactivity to your generative art projects. You can detect user input, such as mouse clicks and key presses, and use this input to control various aspects of your artwork, like colors, shapes, and animations.
Are there any resources for learning more about generative art with p5.js?
Yes, there are many resources available to help you learn more about generative art with p5.js. Some recommended resources include:
- The p5.js website (https://p5js.org/), which includes tutorials, examples, and documentation.
- The Coding Train YouTube channel (https://www.youtube.com/user/shiffman), which features numerous video tutorials on generative art with p5.js. Happy coding and creating!