Complex Generative Art with p5.js
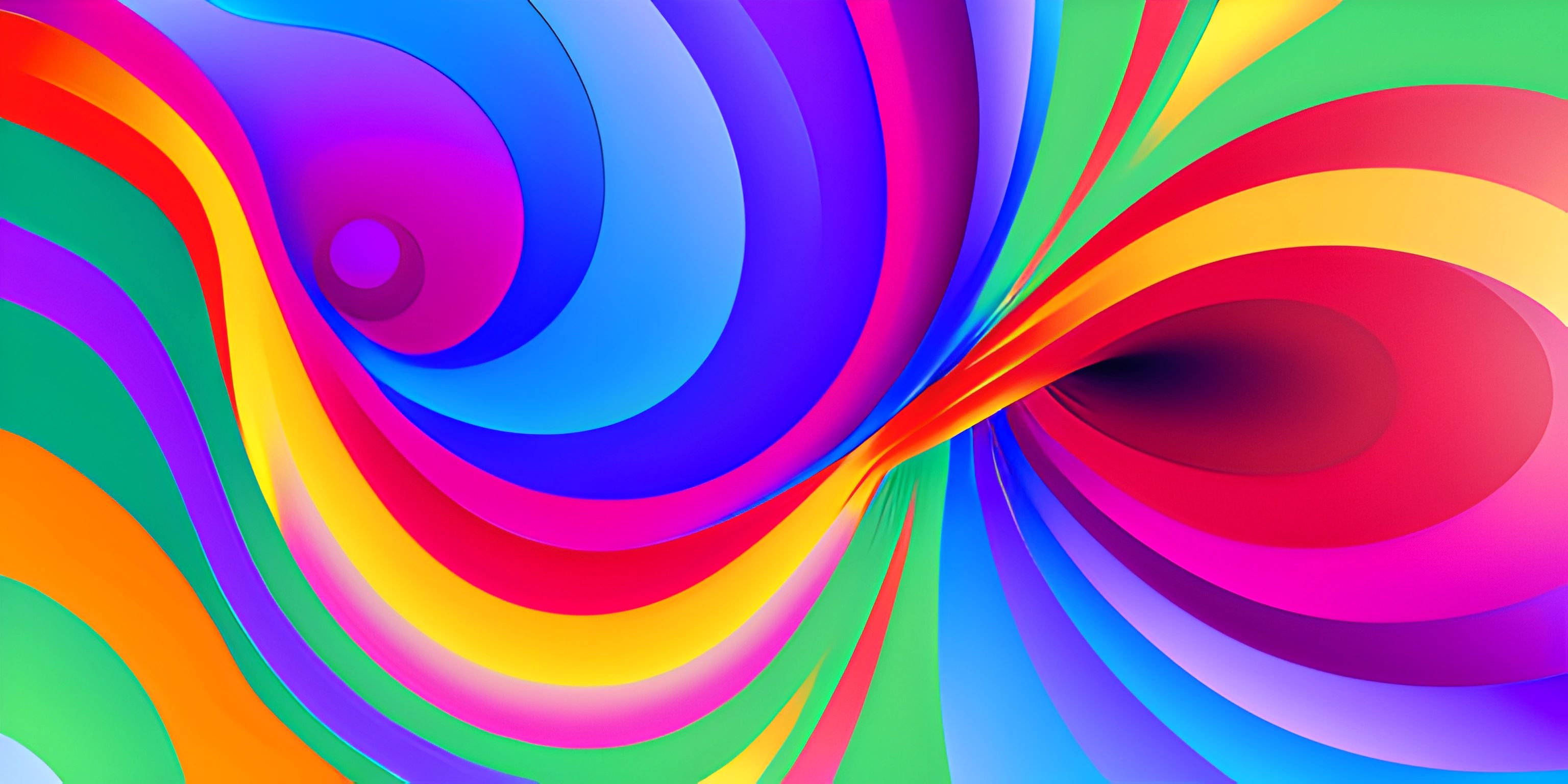
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
In the realm of generative art, exploring the visual beauty of mathematical functions can lead to stunning results. One such exploration is the graphing of complex functions. In this guide, we'll dive into using p5.js and a custom complex number class to create mesmerizing generative art by graphing complex functions. We'll also link to other pages on complex graph art to expand our artistic horizons.
Prerequisites
Before we start, make sure you're familiar with p5.js for creating interactive digital art and complex numbers for mathematical operations with imaginary numbers.
Setting Up p5.js and the Complex Number Class
First, we need to set up our p5.js environment. Create a new HTML file and include the p5.js library:
<!DOCTYPE html> <html> <head> <script src="https://cdnjs.cloudflare.com/ajax/libs/p5.js/1.4.0/p5.js"></script> <script src="complex.js"></script> <script src="sketch.js"></script> </head> <body> </body> </html>
Next, create a complex.js
file for our custom complex number class. This class will handle complex number operations such as addition, subtraction, multiplication, and division:
class Complex { constructor(real, imaginary) { this.real = real; this.imaginary = imaginary; } // Add complex numbers add(other) { return new Complex(this.real + other.real, this.imaginary + other.imaginary); } // Subtract complex numbers subtract(other) { return new Complex(this.real - other.real, this.imaginary - other.imaginary); } // Multiply complex numbers multiply(other) { return new Complex( this.real * other.real - this.imaginary * other.imaginary, this.real * other.imaginary + this.imaginary * other.real ); } // Divide complex numbers divide(other) { const denominator = other.real * other.real + other.imaginary * other.imaginary; return new Complex( (this.real * other.real + this.imaginary * other.imaginary) / denominator, (this.imaginary * other.real - this.real * other.imaginary) / denominator ); } }
Now we have the tools required to manipulate complex numbers and create generative art with p5.js.
Creating Generative Art with Complex Functions
In our sketch.js
file, let's set up the p5.js sketch and create a complex function to visualize. We'll use the Mandelbrot Set as an example, but feel free to experiment with other complex functions.
// Set up the p5.js canvas function setup() { createCanvas(800, 800); pixelDensity(1); noLoop(); } // Draw the Mandelbrot Set function draw() { loadPixels(); for (let x = 0; x < width; x++) { for (let y = 0; y < height; y++) { const a = map(x, 0, width, -2, 2); const b = map(y, 0, height, -2, 2); const c = new Complex(a, b); const n = mandelbrot(c); const color = map(n, 0, 100, 0, 255); const index = (x + y * width) * 4; pixels[index] = color; pixels[index + 1] = color; pixels[index + 2] = color; pixels[index + 3] = 255; } } updatePixels(); } // Calculate the Mandelbrot Set for a complex number function mandelbrot(c) { let z = new Complex(0, 0); let n = 0; while (n < 100) { z = z.multiply(z).add(c); if (z.real * z.real + z.imaginary * z.imaginary > 4) { break; } n++; } return n; }
Here, we create a p5.js sketch that renders the Mandelbrot Set as generative art. The mandelbrot
function calculates whether a complex number belongs to the Mandelbrot Set and returns the number of iterations before escaping.
Going Further with Complex Graph Art
To explore more complex graph art, consider investigating other intriguing functions like the Julia Set, experimenting with color gradients, or even animating the art using p5.js. The possibilities are endless, so unleash your creativity and delve into the mesmerizing world of complex generative art.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Making Art with Code (psst, it's free!).