Overview of the Processing Language
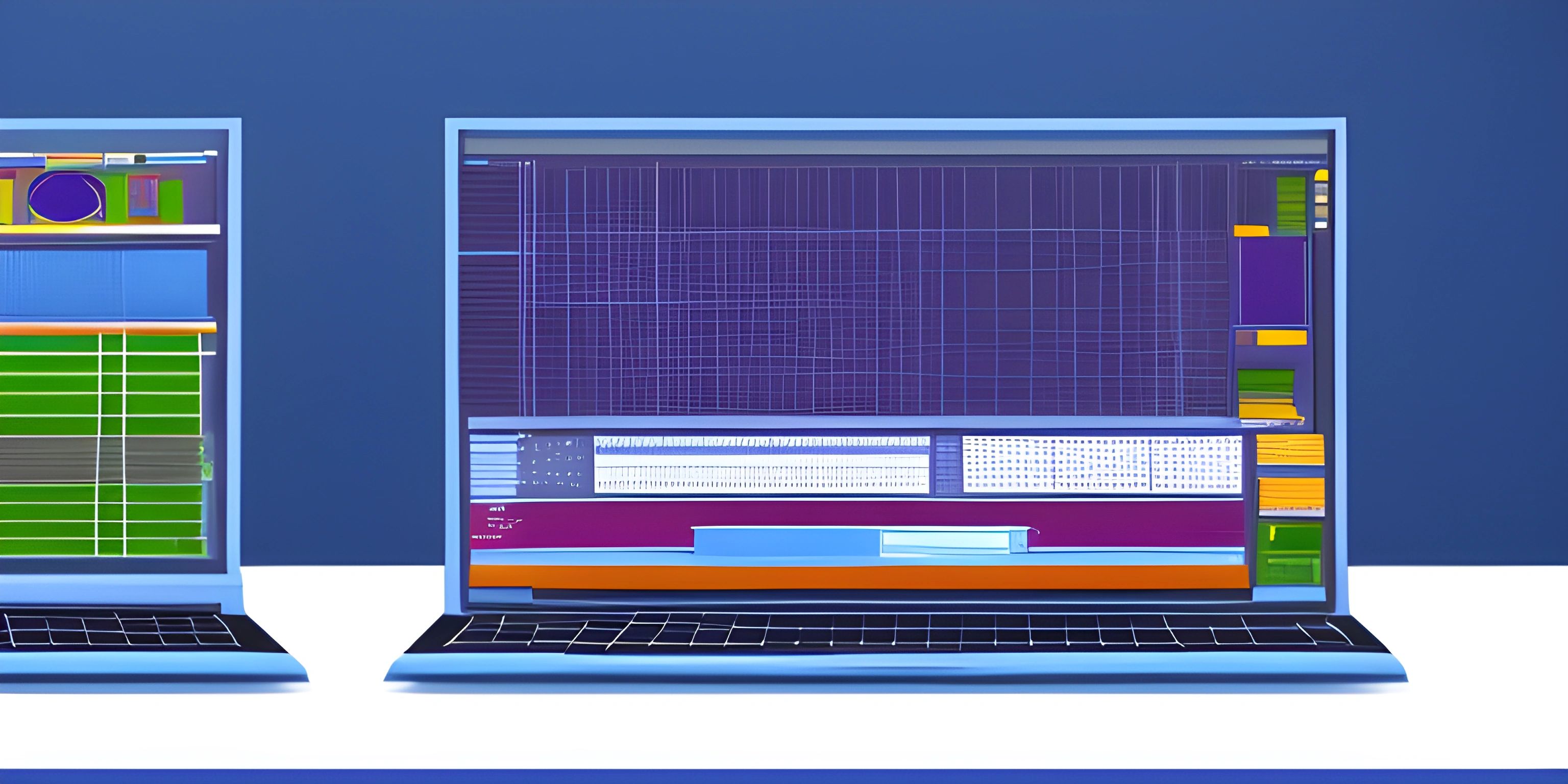
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Ready to dive into a world where code meets art? Enter Processing, a flexible software sketchbook and a language for learning how to code within the context of the visual arts. If you've ever wanted to create stunning visuals or interactive art with a few lines of code, Processing is your new best friend.
What is Processing?
Processing is an open-source graphical library and integrated development environment (IDE) built for artists, designers, and anyone interested in creating visual art through code. Think of it as the secret sauce that artists use to turn algorithms into mesmerizing visuals. It's not just limited to static images; you can create animations, interactive installations, and even complex data visualizations.
The language itself is built on Java, but with a simplified syntax that makes it easy to pick up even if you're new to coding. It's like Java's artsy cousin who went to design school and came back with a flair for creativity.
Setting Up Your Environment
Before you can start making your own digital masterpieces, you need to set up your environment. Head over to the Processing download page and grab the latest version for your operating system. Installation is straightforward: just follow the prompts like you're installing any other application.
Once installed, open the Processing IDE, and you'll be greeted with a blank canvas. This is where your sketches will come to life.
Your First Sketch
Let's start with something simple. A sketch in Processing is essentially a program that draws something on the screen. Here's a classic "Hello World" in the form of a circle:
// Setup function runs once at the start void setup() { // Set the size of the window size(400, 400); // Set the background color background(255); } // Draw function loops continuously void draw() { // Set the fill color fill(0); // Draw a circle at (200, 200) with a diameter of 50 ellipse(200, 200, 50, 50); }
When you hit the "Run" button (the one that looks like a play icon), you'll see a window pop up with a white background and a black circle in the center. Congratulations, you've just created your first Processing sketch!
The Setup and Draw Functions
Processing sketches are structured around two core functions: setup()
and draw()
. Think of setup()
as the stage crew setting up the scene. It's called once when the program starts and is used to initialize settings like the window size and background color.
The draw()
function is the main performer, called repeatedly to create animations or update the display. If you've ever watched a flipbook animation, draw()
is like each page being flipped, creating the illusion of movement.
Drawing Shapes
Processing makes it easy to draw basic shapes. Here are some of the most commonly used functions:
point(x, y)
: Draws a point at (x, y).line(x1, y1, x2, y2)
: Draws a line from (x1, y1) to (x2, y2).rect(x, y, width, height)
: Draws a rectangle with the top-left corner at (x, y).ellipse(x, y, width, height)
: Draws an ellipse centered at (x, y).
Here's an example that combines these shapes:
void setup() { size(400, 400); background(255); } void draw() { fill(0, 102, 153); rect(50, 50, 100, 100); // Draws a blue rectangle fill(255, 0, 0); ellipse(200, 200, 75, 75); // Draws a red circle stroke(0); line(300, 300, 350, 350); // Draws a black line }
Run this, and you'll see a blue rectangle, a red circle, and a black line on the screen. Notice how we can change the fill color using the fill(r, g, b)
function, where r
, g
, and b
are the red, green, and blue color values.
Interactivity
One of the coolest things about Processing is how easy it is to make your sketches interactive. You can respond to mouse movements, keyboard input, and more. Here's an example that makes a circle follow your mouse:
void setup() { size(400, 400); background(255); } void draw() { background(255); // Clear the screen fill(0); ellipse(mouseX, mouseY, 50, 50); // Draw a circle at the mouse position }
In this sketch, the mouseX
and mouseY
variables always contain the current position of the mouse. Run the sketch and move your mouse around the window. The circle will follow your cursor, creating a simple but fun interactive experience.
Animation
Creating animations in Processing is a breeze. Since draw()
is called continuously, you can change the position, size, or color of shapes over time to create animations. Here's a bouncing ball example:
int x = 200; int y = 200; int xSpeed = 2; int ySpeed = 3; void setup() { size(400, 400); background(255); } void draw() { background(255); fill(0); ellipse(x, y, 50, 50); // Update position x += xSpeed; y += ySpeed; // Check for collision with walls if (x > width - 25 || x < 25) { xSpeed *= -1; } if (y > height - 25 || y < 25) { ySpeed *= -1; } }
In this example, the ball moves by xSpeed
and ySpeed
pixels each frame. When it hits the edge of the window, it bounces back by reversing its speed.
Exporting Your Sketch
Once you're happy with your creation, you might want to share it with the world. Processing makes it easy to export your sketch as a standalone application. Just go to File > Export Application
, and you'll get a folder with everything you need to run your sketch on any computer.
Conclusion
Processing is a fantastic tool for anyone interested in combining art and code. With its simple syntax and powerful capabilities, you can create everything from simple drawings to complex interactive installations. Whether you're a seasoned developer or a complete beginner, Processing offers a fun and engaging way to explore the world of creative coding.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Lifetimes (psst, it's free!).
FAQ
What is Processing?
Processing is an open-source graphical library and IDE designed for artists and designers to create visual art through code. It simplifies Java syntax for easy learning and is used for animations, interactive art, and data visualizations.
How do I install Processing?
You can download Processing from the official Processing website. Follow the installation prompts for your operating system, and you'll be ready to start coding in no time.
What are `setup()` and `draw()` functions in Processing?
The setup()
function runs once at the start of your sketch to initialize settings, while the draw()
function loops continuously, acting as the main performer to update the display and create animations.
How can I make my sketches interactive?
Processing allows you to easily add interactivity by responding to mouse movements, keyboard input, and more. For example, mouseX
and mouseY
variables track the current mouse position, which you can use to dynamically update your sketch.
Can I export my Processing sketch?
Yes, Processing allows you to export your sketch as a standalone application. Go to File > Export Application
, and you'll get a folder with everything needed to run your sketch on any computer.