Mandelbrot Set for Generative Art
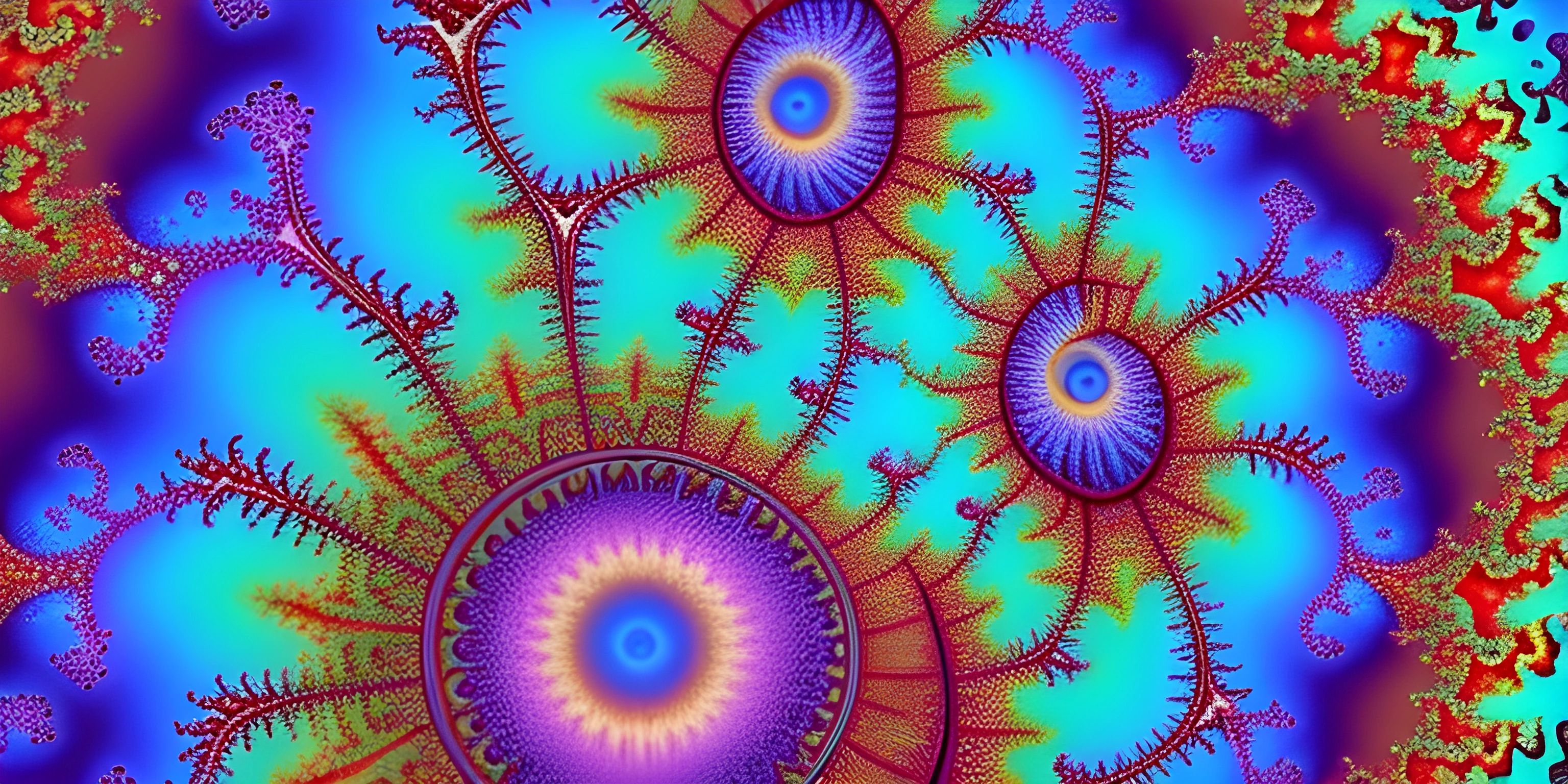
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Are you ready to dive into the mind-bending world of fractals? Buckle up, because we're about to explore the Mandelbrot set, a mathematical marvel that has been a source of fascination for mathematicians, artists, and programmers alike. Named after the mathematician Benoît B. Mandelbrot, this set is not only a cornerstone of complex numbers but also a fantastic tool for generating intricate and captivating art.
What is the Mandelbrot Set?
Imagine a world where you can zoom in forever, revealing more and more detail, all generated by a simple mathematical formula. Sounds like science fiction? Nope! Welcome to the Mandelbrot set. At its core, the Mandelbrot set is a collection of complex numbers that do not escape to infinity when iterated through a specific function.
In simpler terms, we start with a complex number ( c ). Then, we iterate the function ( f(z) = z^2 + c ) starting with ( z = 0 ). If the sequence of ( z ) values remains bounded (doesn't go off to infinity), then ( c ) is part of the Mandelbrot set.
Plotting the Mandelbrot Set
Alright, enough talk. Let's fire up some Python and see this bad boy in action. We'll use the matplotlib
library to visualize our Mandelbrot set.
Step 1: Setting Up
First, let's install matplotlib
if you haven't already:
pip install matplotlib
Now, let's import the necessary libraries and set up our canvas.
import matplotlib.pyplot as plt import numpy as np # Set up dimensions width, height = 800, 800 # Generate a 2D grid of complex numbers real = np.linspace(-2.0, 1.0, width) imag = np.linspace(-1.5, 1.5, height) X, Y = np.meshgrid(real, imag) C = X + 1j * Y
Step 2: Iteration Function
Next, let's define our iteration function to determine which points belong to the Mandelbrot set.
def mandelbrot(c, max_iter): z = 0 n = 0 while abs(z) <= 2 and n < max_iter: z = z*z + c n += 1 return n
Step 3: Creating the Fractal
We will now apply our iteration function to each point in our grid and store the results.
max_iter = 100 mandelbrot_set = np.zeros((width, height)) for i in range(width): for j in range(height): mandelbrot_set[i, j] = mandelbrot(C[i, j], max_iter)
Step 4: Visualizing
Finally, let's visualize our Mandelbrot set using matplotlib
.
plt.imshow(mandelbrot_set.T, extent=[-2, 1, -1.5, 1.5], cmap="inferno") plt.colorbar() plt.title("Mandelbrot Set") plt.show()
Creating Generative Art
Now that we've got the basics down, let's take our fractal game to the next level. By tweaking parameters, adding colors, and layering images, we can create truly unique and stunning pieces of generative art.
Adding Color
One way to spice things up is by coloring the points based on the number of iterations it took to determine their membership in the Mandelbrot set.
plt.imshow(mandelbrot_set.T, extent=[-2, 1, -1.5, 1.5], cmap="twilight_shifted") plt.colorbar() plt.title("Colorful Mandelbrot Set") plt.show()
Zooming In
Want to explore deeper? Let's zoom into a specific region of the Mandelbrot set.
# Define zoom region zoom_real = np.linspace(-0.75, -0.7, width) zoom_imag = np.linspace(0.1, 0.15, height) X_zoom, Y_zoom = np.meshgrid(zoom_real, zoom_imag) C_zoom = X_zoom + 1j * Y_zoom # Apply the iteration function mandelbrot_zoom = np.zeros((width, height)) for i in range(width): for j in range(height): mandelbrot_zoom[i, j] = mandelbrot(C_zoom[i, j], max_iter) # Visualize the zoomed region plt.imshow(mandelbrot_zoom.T, extent=[-0.75, -0.7, 0.1, 0.15], cmap="magma") plt.colorbar() plt.title("Zoomed Mandelbrot Set") plt.show()
Experimenting with Different Functions
Want more variety? Try iterating different functions or using different escape conditions. The possibilities are endless!
def mandelbrot_variant(c, max_iter): z = 0 n = 0 while abs(z) <= 2 and n < max_iter: z = z**3 + c # Cubic function instead of quadratic n += 1 return n mandelbrot_var_set = np.zeros((width, height)) for i in range(width): for j in range(height): mandelbrot_var_set[i, j] = mandelbrot_variant(C[i, j], max_iter) # Visualize the variant Mandelbrot set plt.imshow(mandelbrot_var_set.T, extent=[-2, 1, -1.5, 1.5], cmap="plasma") plt.colorbar() plt.title("Mandelbrot Set Variant") plt.show()
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Mandelbrot Set (psst, it's free!).
FAQ
What is the Mandelbrot set?
The Mandelbrot set is a collection of complex numbers that, when iterated through the function ( f(z) = z^2 + c ), do not escape to infinity. It's known for its intricate and infinitely detailed fractal boundary.
What is generative art?
Generative art is art created using algorithms and computational processes. It often involves using mathematical principles or rules to generate complex and visually appealing patterns.
Why use Python for visualizing the Mandelbrot set?
Python, with libraries like numpy
and matplotlib
, offers powerful tools for numerical computation and visualization. It's relatively easy to use and allows for rapid experimentation and iteration.
Can I use other programming languages to create Mandelbrot set visualizations?
Absolutely! While Python is popular due to its simplicity, other languages like JavaScript (with libraries like p5.js), C++, and even Java can be used to create Mandelbrot set visualizations.
How can I make my Mandelbrot art unique?
Experiment with different iteration functions, color maps, and zoom regions. You can also combine multiple fractal images, apply transformations, or incorporate randomness to create unique and captivating pieces.